The Java Tutorials have been written for JDK 8. Examples and practices described in this page don't take advantage of improvements introduced in later releases and might use technology no longer available. See Java Language Changes for a summary of updated language features in Java SE 9 and subsequent releases. See JDK Release Notes for information about new features, enhancements, and removed or deprecated options for all JDK releases.

Defining Methods
Here is an example of a typical method declaration:
The only required elements of a method declaration are the method's return type, name, a pair of parentheses, () , and a body between braces, {} .
More generally, method declarations have six components, in order:
- Modifierssuch as public , private , and others you will learn about later.
- The return typethe data type of the value returned by the method, or void if the method does not return a value.
- The method namethe rules for field names apply to method names as well, but the convention is a little different.
- The parameter list in parenthesisa comma-delimited list of input parameters, preceded by their data types, enclosed by parentheses, () . If there are no parameters, you must use empty parentheses.
- An exception listto be discussed later.
- The method body, enclosed between bracesthe method's code, including the declaration of local variables, goes here.
Modifiers, return types, and parameters will be discussed later in this lesson. Exceptions are discussed in a later lesson.
The signature of the method declared above is:
Naming a Method
Although a method name can be any legal identifier, code conventions restrict method names. By convention, method names should be a verb in lowercase or a multi-word name that begins with a verb in lowercase, followed by adjectives, nouns, etc. In multi-word names, the first letter of each of the second and following words should be capitalized. Here are some examples:
Typically, a method has a unique name within its class. However, a method might have the same name as other methods due to method overloading .
Overloading Methods
The Java programming language supports overloading methods, and Java can distinguish between methods with different method signatures . This means that methods within a class can have the same name if they have different parameter lists (there are some qualifications to this that will be discussed in the lesson titled "Interfaces and Inheritance").
Suppose that you have a class that can use calligraphy to draw various types of data (strings, integers, and so on) and that contains a method for drawing each data type. It is cumbersome to use a new name for each methodfor example, drawString , drawInteger , drawFloat , and so on. In the Java programming language, you can use the same name for all the drawing methods but pass a different argument list to each method. Thus, the data drawing class might declare four methods named draw , each of which has a different parameter list.
Overloaded methods are differentiated by the number and the type of the arguments passed into the method. In the code sample, draw(String s) and draw(int i) are distinct and unique methods because they require different argument types.
You cannot declare more than one method with the same name and the same number and type of arguments, because the compiler cannot tell them apart.
The compiler does not consider return type when differentiating methods, so you cannot declare two methods with the same signature even if they have a different return type.
About Oracle | Contact Us | Legal Notices | Terms of Use | Your Privacy Rights
Copyright © 1995, 2022 Oracle and/or its affiliates. All rights reserved.
Programming Duck
Home » Articles » Naming (in code) – The ultimate guide and reference
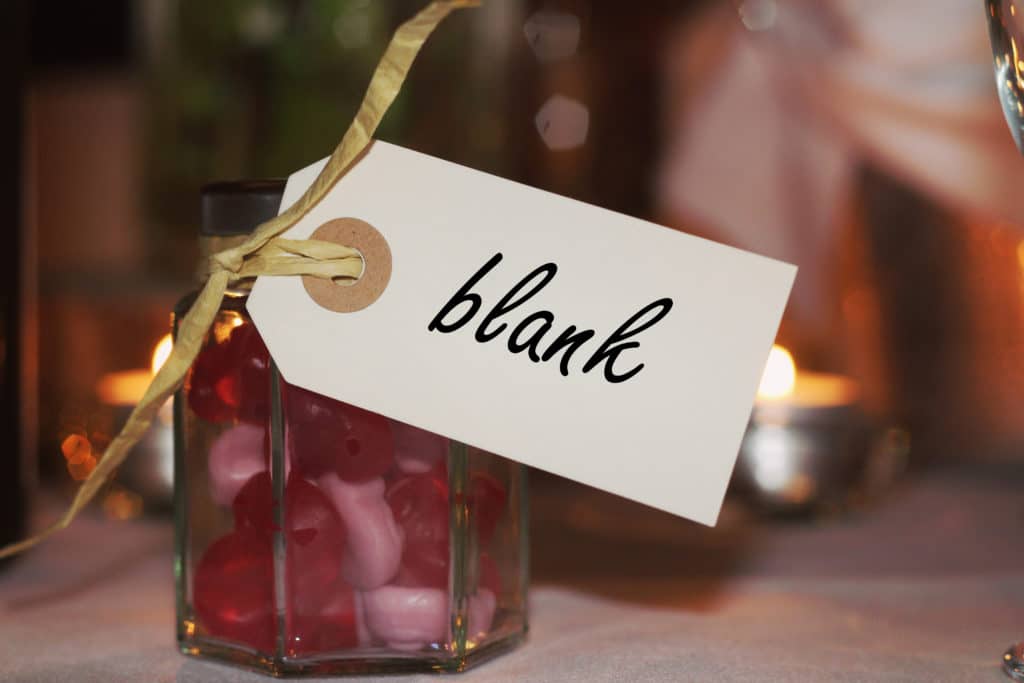
Spyros Argalias
Naming (in code) – the ultimate guide and reference.
- Updated on 21st June 2021
Programming principles tell us that naming in code is important. This article aims to be a complete learner’s guide for naming in code. It also aims to be a reference about naming that you can refer to in the future if you need to.
For certain things, such as naming methods, there are different naming conventions. We’ll mention a few so that you’re aware of how they work and the options you can choose from.
Overall, we’ll examine:
- the motivation for wanting good names
- general naming tips for all code
- established naming conventions for specific things such as variables and classes
Motivation for good names
The motivation for good names comes from clean code and programming principles . Code should be:
- immediately obvious and easy to understand
- easy to change
Being easy to understand helps because:
- code that’s easy to understand will be faster to understand. This means that you can work faster. You’ll spend less time trying to understand old code and more time writing new code.
- code will be possible to understand. In comparison, if some code is difficult to understand, you may not understand it even after spending a long time reading it. Someone who’s less experienced may have even worse luck. Additionally, you may misunderstand how the code works, especially if you’re not fully paying attention that day. Misunderstanding code makes it very easy to create bugs.
Good names help with both cases.
How good names make code faster to understand
If something is well-named, then you don’t need further details to understand it. This saves you time.
For example, consider:
- a function named printHelloToTheScreen
- a function named multiplyBy2
- a constant named PI
- a constant named MAXIMUM_ALLOWED_LOGIN_ATTEMPTS
- a static class or module named Math
- a variable named circumference
- a variable named userInfo
In a codebase, you would have a pretty good idea of what those do just from the name.
How good names make code easier to understand
When reading code, you have to:
- read (parse) what it does
- understand why it does it, or rather, understand what it’s trying to do conceptually
For example, it’s not enough to think "this code removes initial whitespace from the string, then it sends a network request". Instead, you have to understand "this code formats the username and then sends a password reset request".
Here’s a code example that’s difficult to understand:
What’s the 8 there for? What’s b ? Why does it return a minimum of 1 ? What’s the purpose of this function?
If you’re trying to do something such as change the area, you don’t know if this function is relevant or not. Even if you suspect it is, you don’t know what it does or why.
Something like this would be much better:
So, good names help, because they provide meaning . They help you understand what the code does and why it does it.
To get the benefit, all you have to do is give a good name to the thing.
As another example, someone may not understand PI * a ** 2 . They may think " PI , a , power of 2… What the heck is that??". To make it easier, all you have to do is replace that line with const circleArea = PI * radius ** 2 . It helps a lot.
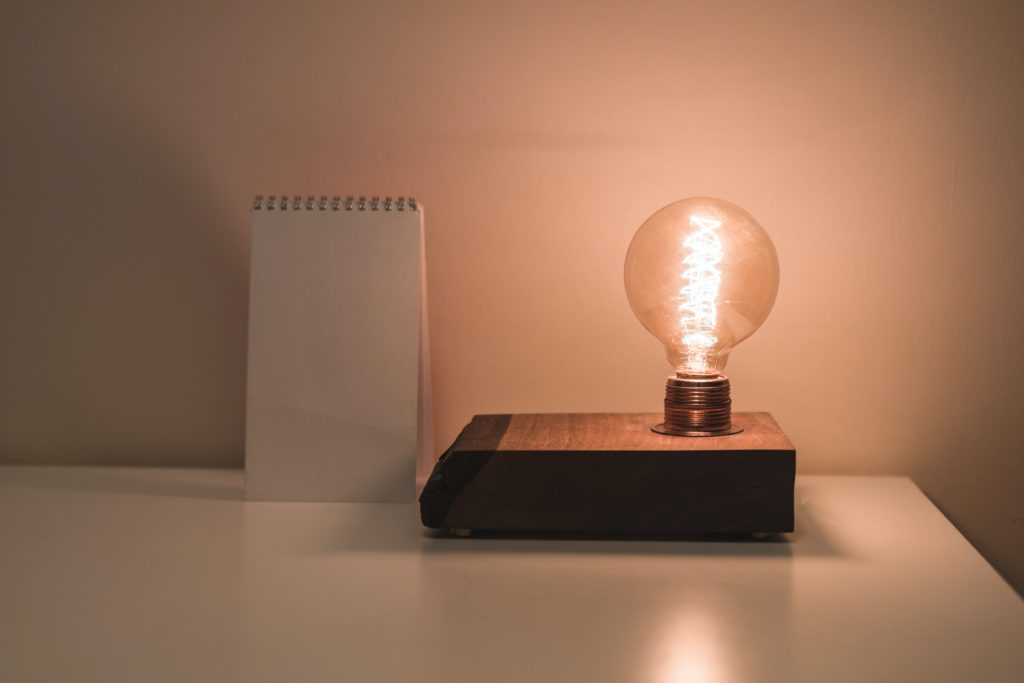
General naming tips for code
In short, a good name is something that immediately tells you what something is or does. It’s not surprising. It doesn’t take effort to understand it. You understand it without thinking about it.
A bad name is something that you read and you wonder "what’s that", or "what does that do?". Something that requires you to think more.
When writing names, they should be understandable by someone that’s never seen this code before. They need to make sense, be consistent and be sufficiently descriptive.
Here are some pointers to accomplish this.
Have clear naming conventions
Your codebase should have clear conventions. This is probably the most important point in this article. If you’re already following a convention, it’s probably best to stick to it, even if an alternative may be more descriptive.
Conventions apply consistency. Programming principles tell us that consistency is very important.
It allows you to work faster. You can make certain assumptions about how the code works and what certain things mean. Additionally, when thinking of a name for something, the convention may already have a rule for it. This makes things easier.
Also, not following conventions can cause mistakes. You may assume that a common convention is being followed in the codebase. If you’re wrong, then you may have the wrong idea about how some code works. At that point, it’s very easy to create bugs.
Ideally, you should follow conventions that already exist in your programming language or framework. This makes things easier for new developers to the company. Some examples are the .NET naming guidelines and Airbnb JavaScript style guide . Otherwise, you can also create your own custom conventions for your project or company.
Prefer descriptive names
Always consider, will the next person who looks at this name understand it easily?
Use full words
Generally, full words are easier to understand. For example calculateArea or createWindow . Abbreviated words may be harder to understand. In general, avoid names like calcArea or cWin .
Avoid very short variable names
In particular, avoid single-letter or very short variables, such as d . It could mean anything: the document , data , date , or days .
The exception to this is when the name is used in a very small scope and what it represents is immediately obvious. In this case, it’s easy to look one or two lines up and see where it was defined. Here’s a code example using the variable e .
Here, the variable e represents the event object. We know this because the name handleFormSubmission and the function signature represent an event handler. e is only used one line under where it is defined, so it’s still easy to work with.
Nevertheless, I would still personally use event . I consider readability more important than minor saving of keystrokes like that.
Another acceptable example is using i in for loops and such. That’s a convention that everyone understands.
If an acronym is very common, then it’s okay to use it. Some examples are the acronyms HTML, UI, IO, OS, JSON, XML, HTTP.
If an acronym is uncommon, then prefer the fully expanded name. If in doubt, you should probably use the full version.
Conciseness matters
Names that are sufficiently descriptive come first. However, that doesn’t mean that you need super long names.
Super long names, such as a variable named integerNumberOfEnginesInObject are difficult to work with. engineCount would be sufficient.
In general, if you can have the same clarity with a shorter name, use the shorter name.
Consider the context
The name of the surrounding context can provide useful clues for the purpose of something. This means that sometimes, you can get away with using shorter names.
For example, if you have a class called Users , then the method for creating a new user can be called create . The usage of it would be something like users.create() (where users is an instance of Users ). That’s sufficiently descriptive. You don’t need to call the method createUser in this case.
The prevalent casings in programming (excluding HTML and CSS) are pascal case, camel case and snake case. The one you use depends on the conventions for your programming language or framework.
General format of each casing
Snake casing is lower cased and uses underscores to separate words. For example this_is_snake_case .
In pascal casing, every word begins with a capital letter. For example ThisIsPascalCase .
Camel casing is similar to pascal casing, except that the first word begins with a lowercase letter. For example thisIsCamelCase .
Casing for acronyms
For acronyms, the conventions vary.
On the front end, it seems that acronyms are always fully capitalised, regardless of length. Some examples are performance.toJSON() , XMLDocument , HTMLCollection and DOMString .
However, in some other languages, such as the .NET languages, the convention is:
- if an acronym is only two letters long, the second letter should have the same casing (upper or lower case) as the first one. For example, UIInput (pascal case), uiInput (camel case) and fooUIInput .
- if an acronym is three letters or longer, only the first letter should be capitalised if necessary. For example JsonFoo (pascal case), jsonFoo (camel case).
Capitalisation for compound words
It’s a convention to treat compound words as one word in terms of capitalisation. For example, use callback and endpoint instead of callBack and endPoint . You can find a thorough list of common compound words, used in programming, on the .NET naming guidelines on capitalisation .
Prefer semantic naming
Semantic naming means to name something after its purpose or meaning. In order of priority, this means:
- what the purpose of it is, or what it does
- how it does it
As a bonus, it also results in code that is less likely to change in the future.
When naming things, consider: what’s the most important thing about the thing you’re naming? What’s the most suitable name for someone else to understand it conceptually?
Usually, users care more about what something is doing, rather than how it’s doing it. Although, sometimes, it’s the "how" that’s the most important.
Here are some examples of semantic naming.
Example with a variable name
If you have a variable that holds a collection of users, the important part is that it contains users. Whether it’s a list or a map is less important. Not to mention that your IDE and type declarations provide that information anyway.
Therefore, the name:
- users would be suitable
- userList is less preferable. The "list" part is less important than the users part. Also, if you change the data structure in the future you’ll have to update the variable name.
- userCollection is also less preferable, because the "collection" part is less important than the "user" part. However, at least you won’t have to update the variable name if you change the data structure in the future.
Example with interfaces and implementations
In OOP code, you tend to have interfaces with potentially multiple implementations. An interface may be called something semantic and generic, such as Sorter , for a sorting algorithm. That’s the significant thing about it, the fact that it sorts. The how isn’t important at the interface level.
The different implementations can be called after the sorting algorithm they implement. That’s the important thing about them and the only thing that separates them from one another. It’s the information a user of that specific class would want. For example BubbleSort , MergeSort , Quicksort .
Example with a method for sorting
Imagine that you have a class containing a collection of something. You also have a method for sorting it, say, alphabetically.
If you only have one sorting method, then it would be preferable to name it sort . A name such as sortWithQuicksort or quicksort would be unnecessary information that the user doesn’t care about. If you think about it, the caller of the code wants to sort. They aren’t particularly interested in the specific algorithm your class uses. (The only exception is if your class is an actual bottleneck to performance or something, but that’s a different topic.)
Additionally, in the future, you may change the sorting algorithm that the class uses to merge sort. In that case, the name sortWithQuicksort wouldn’t make sense any more.
The solution is to name your public method sort .
The fact that you’re using quicksort is still important. Developers working on the implementation of the class would want to know that. Some options for that are to have a private method named quicksort , or import and use a quicksort function from somewhere else in the codebase.
For example:
Example with front end CSS
In front end CSS, there are a few approaches. The approach you choose decides what’s important about the name of your class.
When using the BEM naming convention, the important thing is the purpose of the element, not the styling. For example, you would use the CSS class page-heading instead of large-heading-red . That’s because, tomorrow, the styling of the page heading may change. At that point, the non-semantic name won’t make sense anymore, but the semantic name will.
If you’re using a UI component library, then the styling of the component is more important than the purpose of the component. For example button-primary instead of add-to-cart-button . That’s because classes like button-primary are the main ones you’ll be working with throughout your code.
Other tips from Uncle Bob
Some tips that are mentioned in Uncle Bob’s book Clean Code are to:
- make meaningful distinctions. Avoid variables that seem similar or the same, such as accountInfo or accountData . A user of that code won’t be able to tell the difference.
- use pronounceable names. Names should be easy to read out loud. Some examples to avoid are names such as genymdhms and modymdhms . Better names would be generationTimestamp and modificationTimestamp .
- use searchable names. Names should be easy to search using your code editor. Essentially, avoid names that are one or two letters long, as searching for them will return too many matches.
- avoid using cute / offensive words. Avoid names such as whack() and seeYouLaterFiles() . Be professional and consistent.
- pick one word per concept. For example, don’t mix the words "fetch", "get" and "retrieve" for the same kind of operation throughout your codebase. Pick one of them and use it consistently.
- avoid puns. Avoid using the same word for different concepts. For example, if in one class add() adds two numbers, in a different class add() shouldn’t insert into a list.
Other tips from the .NET naming conventions
.NET makes some additional recommendations. Some of them are specifically for .NET languages. However, you may want to keep them in mind anyway.
The recommendations are to:
- prefer naturally readable names. For example, use HorizontalAlignment instead of AlignmentHorizontal .
- avoid using names that conflict with common keywords in other programming languages
- prefer semantic names rather than language specific names. For example, use GetLength instead of GetInt .
- prefer generic CLR type names instead of language-specific names. For example name a method ToInt64 instead of ToLong . That’s because methods like these may be used in other CLR-compatible languages where the data type long doesn’t exist. Therefore, that name won’t make sense in those languages. However, Int64 exists and makes sense for all CLR languages.
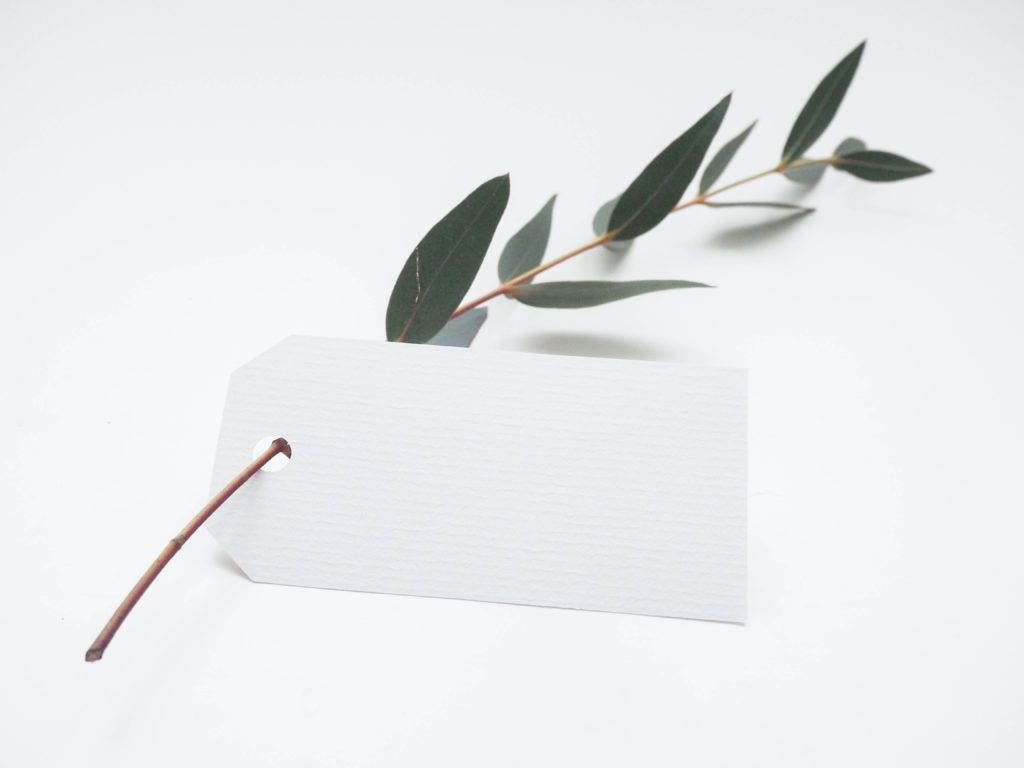
Naming conventions for specific use cases
Here are some common naming conventions for things such as variables, functions, classes, etc.
Variables are just names or labels for values and objects. Some general conventions for them are that:
- they should be nouns. For example car , user , userLocation , student , result , area , player
- they generally use camel case or snake case, depending on your programming language
The rules change a bit for constants and variables holding Boolean values.
Some programming languages write constants fully capitalised and with snake case. This includes JavaScript, Python and Java. Some example constants are:
- Number.EPSILON
- NUMBER_OF_LIVES
- MAX_ATTEMPTS_BEFORE_LOCKOUT
- SOME_SPECIAL_VALUE
Here, "constant" refers to special values. These are values that don’t depend on runtime. They could easily be placed in a configuration file, away from your application code.
"Constant" doesn’t refer to a normal local variable that just happens to be immutable. Those follow the same conventions as normal variables.
Here are some examples of "constants" and "normal variables that just happen to be immutable":
- final MAX_ATTEMPS_BEFORE_LOCKOUT = 5 (this could be in a configuration file, it’s a special constant)
- final float result = SomeClass.factorial(someNumber) (this is just a local variable that happens to be immutable. It varies at runtime depending on someNumber . It can’t be placed in a configuration file)
- const URL_ENDPOINT = '/ajax/foo' (this could be in a configuration file, it’s a special constant)
- const filteredArray = array.filter(a => !a) (this is just a local variable that happens to be immutable. It varies at runtime depending on array . It can’t be placed in a configuration file)
For variables that have Boolean values, the convention is to phrase them as a question. Start them with a predicate such as "is", "has", "had" and "can". These clearly denote that the variable holds a Boolean.
Some example Boolean variables are:
- hasDescendants
- canTakeDamage
In addition to being readable standalone, they read nicely in conditional statements:
In comparison, if you don’t use a predicate, you would use a name such as complete . This is both a verb and an adjective. It can denote a number of things. It could be a function that you run to complete something, or a variable that holds things that have already completed, or a Boolean that states whether something has completed or not, or an event name. What it represents is more ambiguous, so prefer predicates instead.
A verb like completing is slightly better. It can’t denote a function, because the "ing" in "completing" means that something is already happening. It’s not something that you can start running now (like a function call). However, it can still be any of the other options. Overall, it’s still preferable to use predicates.
Functions are units of code that do something. Conventions for function names are that:
- they should be verbs
Some example function names are:
- createReport
- sendRequest
For functions that return a Boolean, a common convention is for them to start with a predicate. This is similar to variables that contain Boolean values.
Some example names for functions that return Booleans are:
- hasSignedIn
- isFormatted
Another convention I’ve seen is for "transformer" or "converter" functions. These are functions that convert from one thing to another. They usually begin with "to", followed by the type they’re converting to.
Some examples of transformer function names are:
- toLowerCase
- toUpperCase
Classes are units of code that contain methods and attributes.
Some conventions for classes are that:
- they use Pascal case
- they should be nouns (or noun phrases)
Some example class names are:
- ImageSprite
General property names
In general, attributes are named similarly to variables. Methods are named similarly to functions.
However, as mentioned earlier, the name of the class provides some context.
This means that you may be able to use a less specific name for a method / attribute than you would use for the equivalent function / variable.
For example, inside a class Users , you may have a method named create . The usage of it would be something like users.create() (where users is an instance of Users ), which is sufficiently descriptive.
Casing and prefixing for properties
In terms of casing and prefixes, different languages and frameworks have different conventions.
For example, the Java naming conventions mention that methods and attributes should be camel cased.
The PEP 8 Python naming convention also mentions camel casing. However, it adds that private properties should be prefixed with an underscore. For example ( _privateProperty ).
The C# coding conventions seem the strictest. They state that:
- public properties should be pascal cased (for example PublicMethod or PublicVariable )
- private or internal properties should be prefixed with underscore and be camel cased (for example private void _privateMethod )
- private or internal static properties should be prefixed with s_ and be camel cased (for example private static foo s_workerQueue )
- private or internal thread properties should be prefixed with t_ and be camel cased (for example private static foo t_timeSpan )
If, for whatever reason, you were creating your own convention, I would personally recommend:
- pascal casing for methods and camel casing for attributes (this is the convention used in the Unity game engine). The reason for this is be to differentiate between Boolean attributes (such as isValid ) and methods that return Booleans (such as IsValid(data) ). As a second choice, I would use camel case for methods.
- underscore prefix for non-public properties
- possibly (not sure yet) the s and t prefixes from C# for the relevant things. As the Zen of Python says "Namespaces are one honking great idea — let’s do more of those!" (I consider prefixes to have a similar effect)
Names for interfaces are similar to class names. They use pascal case and are usually nouns. Sometimes they can be named with an adjective, for example "Readable".
In the book Clean Code , Uncle Bob recommends avoiding the I prefix for interfaces. The Java naming conventions recommend the same. For example interface Foo .
The C# coding conventions recommend prefixing interfaces with I. For example interface IFoo .
Personally, I have a minor preference for avoiding the prefix. That’s because, as a user of the code, I’m not particularly interested on whether I’m working with an interface or a class.
The Java convention is to treat enums like classes with constants. This means to name the enum type with pascal case and the fields fully uppercase.
C# also treats them the same as classes. This means to name both the enum type and the fields using pascal case.
Personally, I prefer the Java convention because it differentiates between constants and other values.
Events, event handlers, messaging systems and commands
There are a few things to consider regarding events and their relevant functions.
Event names
Event names:
- refer to an action
- are normally named using verbs. For example "downloaded", "loaded", "deleted", "damaged", "moved".
- generally use present tense for events that fire before an action starts. For example, before submitting a password reset request, you might fire an event named "passwordResetRequestSubmitting".
- generally use past tense for events that fire after an action completes. For example, after submitting a password reset request, you might fire an event named "passwordResetRequestSubmitted".
In my experience, these conventions are common across many languages. However, there isn’t much official documentation for them. One exception is with .NET, where they formally state these guidelines .
For the actual name of the event, you can use whatever makes sense to you. If your event is created in a class, the class name may provide sufficient context. For example, if you have a class Shape , you may have an event named areaChanged . The usage would be something like myShape.areaChanged += someHandler or myShape.areaChanged.subscribe(someHandler) . The event name "areaChanged" is sufficiently descriptive in this case.
In terms of casing, follow the convention in your programming language. C# uses pascal casing for public members (including events). Most other languages use camel casing.
Some example event names are:
- fileDownloading / fileDownloaded
- userRegistering / userRegistered
- uiElementXUpdating / uiElementXUpdated
Event handlers
For event handler functions, there are a few conventions. In front end, React tends to use the prefix "handle" . C# recommends the suffix "EventHandler". Other languages may have other conventions.
Some example names of event handler functions are:
- handleUserSubscribed / userSubscribedHandler / UserSubscribedEventHandler
- handleFileDownloaded / fileDownloadedHandler / FileDownloadedEventHandler
My personal preference is to use the "handle" prefix. This keeps the function a verb, which is the convention for functions.
"On " functions
Functions that exist solely to raise events tend to have the name on<EventName> . For example "onAreaChanged".
One case for using these is in classes that may be derived.
Here’s a C# example:
Here, we have a method named OnFileDownloaded whose only purpose is to raise the event.
This convention may work a bit differently in front end, but it still follows the general idea. For example, in React, you can name props something like "onClick" or "onSomeEvent". Event handler functions that you define inside a component can use the "handle" prefix.
Here’s a React example:
Here, each component creates event handler functions prefixed with "handle". The child component has the prop named "onClick". In the child component, the handleClick function calls the onClick prop.
More global message names
PubSub (message bus) and analytics have similarities to local events, but they are more global. They span a much larger area of the codebase. The can even span multiple applications (as might be the case for analytics).
With these, it’s important to use more specific names, otherwise you won’t know what they refer to.
A good way to do this is to use namespaces and prefixes, along with specified delimiters.
For example, with PubSub event names, you can have a namespace for the relevant area of the codebase. The format of the event name can be <areaOfCodebase>/<eventName> . For example:
- ui/themeChanged
- ui/fontSizeChanged
- player/damaged
- player/poweredUp
- user/registering
- user/registered
- user/deleted
And, as explained on clean analytics by David Wells , for analytics you could use a format like <Source>:<object>_<actionName> . For example:
- site:newsletter_subscribed
- app:site_deployed
- cli:user_login
- api:site_created
These are just examples. In your own codebase, you can use as many namespaces as you want. The namespaces can be anything.
Likewise, the delimiter(s) can be anything you want. Some examples are "/", "::", ":", "_", or even no delimiter.
Commands are written similarly to functions. They are verbs in an imperative mood. They are also used in PubSub. The notes about namespaces and delimiters, mentioned above, apply to them.
Commands also usually expect a response. In other words, a command such as "CreateUser" will have a response message such as "CreateUserResult", "CreateUserSucceeded" or even "UserCreatedNotification". Overall, I’m not aware of strong conventions for these, so you can probably use whatever you like.
My personal preference for response names comes from Jimmy Bogard’s post on message naming conventions . I generally append "Result", "Reply" or "Response" to the original command name.
Some example formats for command names and their namespaces are <Verb><Subject> and <subject>/<verb> . For example:
- Possible commands are "RegisterUser" or "user/register". Possible responses are "registerUserResponse" or "user/register_result"
- Possible commands are "DamagePlayer", "player/damage". Possible responses are "DamagePlayerResponse", "player/damage_result"
For file names, you need to consider conventions for casing, as well as what to name the file based on the code it contains.
File name casing conventions and delimiters
For file naming, there are different conventions depending on the language, framework and style guide you follow.
Many conventions recommend file names that are all lower case. Words can be separated with either hyphens (-) or underscores (_). For example:
- in HTML, the convention is all lower case, with hyphens as separators. Underscores are far less common. One reason for this is because the HTML file name may reflect in the URL (especially with older servers). Underscores in URLs are far less common than hyphens.
- in CSS, the convention is all lower case with hyphens or underscores as separators
- In Python, PEP 8 recommends file names to be all lower case, with underscores as separators
- the Google JavaScript style guide recommends file names to be all lower case, with underscores or hyphens as separators
Between hyphens and underscores, you can use either. Both are acceptable. In general, I prefer to use hyphens to be consistent with my CSS classes (which conventionally use hyphens) and for the reasons mentioned for the HTML. However, if you commonly use snake case in your programming language, or if you don’t write HTML and CSS, it might feel more consistent to use underscores instead of hyphens.
Some example file names are:
- index.html, index.css, index.js
- third-party-analytics.js
- enemy-mover.js
Along with that, there are other conventions that recommend camel or pascal case for your files. For example:
- C# and Java recommend naming your file the same as the main thing in your file. This means using pascal case, same as your classes and interfaces.
- the AirBnB JavaScript style guide recommends naming your file the same thing as your default export. Again, this means using camel or pascal case, at least for your JavaScript files.
- MyComponent.js
- MyComponent.css
- MyComponent.test.js (test files tend to have the special extension .test.js)
So which should you choose? First, consider if one of the conventions is more common than the others for your programming language or framework. That’s the natural choice. Otherwise, you can do whatever you like. My personal recommendation is to choose the naming convention that best matches the code in your files. For example, when working on the front end, the convention is for everything in your HTML and CSS to be lower case with hyphens as separators. Therefore, you might want to use that as the naming convention.
As another example, when working on React applications that use CSS modules, you might prefer to write CSS using pascal case and underscores. This makes it easier to use your CSS in your JavaScript, for example styles.Foo_bar (where Foo_bar is your CSS class), as hyphens aren’t allowed in JavaScript. In this case, it may feel more natural to name your files using pascal case.
Choosing a file name based on the code it contains
In general, you want to name your file based on its purpose.
This is supported by the C# naming conventions and Java naming conventions. They state that your file name should be the same as the main thing you define in your file. For example, if you define a class Foo, your file name should be Foo.cs or Foo.java.
The AirBnB JavaScript style guide also agrees with this. It states that your file should be named after your default export. Whether or not you use default exports is a separate matter. But the point is the same. It means to name it after the purpose, or the most important thing in your file. Normally (but not always) that’s thing that you would export default , if you were using export default.
One exception is if your default export is named "main", "setup" or something similar. It doesn’t make sense for a file to be called "main", especially if many files have a similar default export. In that case, consider what the purpose of the file is. An alternative is to consider what the equivalent OOP code would be.
For example, consider that you have a class that deals with carousel functionality. Your OOP code would probably be a class named "Carousel". In comparison, if you write it using functions, it might look like this:
In the equivalent OOP code, the code in main would be in the constructor of the class Carousel . In this case, my recommendation would be to name the file carousel . That’s its true purpose. Alternatively, you could also change main to setupCarousel or something and name the file after that.
As for some other cases:
- If your file defines multiple classes: Well, most style guides would tell you to avoid files with multiple classes. Consider whether you can separate each class into its own file. Although, if you only have one public class, then that’s fine. Name the file after the one public class, not the private ones.
- If you use functions instead of classes, there might be some cases where none of the functions would suitable to export default. Again, you need to consider what the purpose of the file is. For example, if you have some functions that are used in your test files, then perhaps "test-utilities" would be a good name. Alternatively, you can consider what the equivalent OOP code would be. You would probably have a static class with static methods. Name your file after the name of that static class.
Package names and namespaces
In practice, many packages don’t follow naming conventions at all. That’s because a developer can upload a package with whatever name they want. There usually aren’t many checks.
However, there are naming conventions for packages and for different package repositories.
Java and Maven use the format of <groupID><artifactID> . The group ID part is generally a reversed domain name. For example, if your domain is example.com, the group ID would be "com.example". It can have subgroups (additional namespaces). For example "com.example.plugins". The artifactID is the name of the jar. For example "junit" or "spring-web". In general, artifact IDs tend to be fully lower case with hyphens to separate words.
NPM (node package manager) tends to use direct package names, such as "react-dom", or prefixed with a namespace or company name, such as "@babel/preset-env". They tend to be fully lower case with hyphens to separate words.
With NuGet (package repository for .NET), the number of namespaces vary, just like with Maven and subgroups. There are many packages with a single name, such as "Moq". There are also many packages of the format <CompanyName>.<Package> , such as "AWSSDK.Core". There are also packages with many namespaces, such as "Microsoft.Extensions.FileProviders.Abstractions". If you want to follow the example of the packages released by Microsoft, then use pascal case, optionally a company prefix and as many namespaces as you need.
Namespaces (in code) seem to follow similar concepts and conventions to packages.
Remember that naming is hard.
There are only two hard things in Computer Science: cache invalidation and naming things. – Phil Karlton
However, spending some time to come up with a reasonable name is usually worth it.
Also, as always, be pragmatic. It’s okay to break these conventions once in a while. They are only here to help you make good and consistent decisions most of the time. For example, it’s okay if you feel that adding the data structure to the name will help, such as userList . It’s up to you to decide what’s best for your project.
In addition, you probably can’t spend an unreasonable amount of time coming up with good names. So, sometimes, if you’ve already spent too long, you might need to use the best name you’ve come up with so far and move on.
Overall, the most important thing from this article is that you understand the principles behind naming. In particular, that names should make the code easy to understand. If you understand that, then you’ll be fine. You’ll be able to come up with your own solutions and conventions even in unfamiliar situations.
Final notes
So that’s it for the article. I hope that you found it useful.
If you’ve encountered any awful names in code you’ve worked on, please leave a comment with them!
Also, if you want to discuss anything, disagree with anything, or have any feedback in general, please leave a comment below.
Alright, see you next time 🙂
Image credits
- Cover photo (modified) – Photo by Paul Stollery on Unsplash
- Clear Bulb Beside White Notepad on White Surface – Photo by Burak Kebapci on Pexels
- Green leaf with white card – Photo by Helena Hertz on Unsplash
Java Tutorial
Java methods, java classes, java file handling, java how to, java reference, java examples, java class methods.
You learned from the Java Methods chapter that methods are declared within a class, and that they are used to perform certain actions:
Create a method named myMethod() in Main:
myMethod() prints a text (the action), when it is called . To call a method, write the method's name followed by two parentheses () and a semicolon ;
Inside main , call myMethod() :
Try it Yourself »
Method Parameters
Methods can also have parameters, which is used to pass information:
Create a method that accepts an int parameter called x. In the main() method, we call myMethod() and set an int parameter of 10:
Static vs. Public
You will often see Java programs that have either static or public attributes and methods.
In the example above, we created a static method, which means that it can be accessed without creating an object of the class, unlike public , which can only be accessed by objects:
An example to demonstrate the differences between static and public methods :
Note: You will learn more about these keywords (called modifiers) in the Java Modifiers chapter.
Access Methods With an Object
Create a Car object named myCar . Call the fullThrottle() and speed() methods on the myCar object, and run the program:
Example explained
1) We created a custom Main class with the class keyword.
2) We created the fullThrottle() and speed() methods in the Main class.
3) The fullThrottle() method and the speed() method will print out some text, when they are called.
4) The speed() method accepts an int parameter called maxSpeed - we will use this in 8) .
5) In order to use the Main class and its methods, we need to create an object of the Main Class.
6) Then, go to the main() method, which you know by now is a built-in Java method that runs your program (any code inside main is executed).
7) By using the new keyword we created an object with the name myCar .
8) Then, we call the fullThrottle() and speed() methods on the myCar object, and run the program using the name of the object ( myCar ), followed by a dot ( . ), followed by the name of the method ( fullThrottle(); and speed(200); ). Notice that we add an int parameter of 200 inside the speed() method.
Remember that..
The dot ( . ) is used to access the object's attributes and methods.
To call a method in Java, write the method name followed by a set of parentheses () , followed by a semicolon ( ; ).
A class must have a matching filename ( Main and Main.java ).
Advertisement
Using Multiple Classes
Like we specified in the Classes chapter , it is a good practice to create an object of a class and access it in another class.
Remember that the name of the java file should match the class name. In this example, we have created two files in the same directory:
Second.java
When both files have been compiled:
Run the Second.java file:
And the output will be:

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
Learn Java practically and Get Certified .
Popular Tutorials
Popular examples, reference materials, learn java interactively, java introduction.
- Get Started With Java
- Your First Java Program
- Java Comments
Java Fundamentals
- Java Variables and Literals
- Java Data Types (Primitive)
- Java Operators
- Java Basic Input and Output
- Java Expressions, Statements and Blocks
Java Flow Control
- Java if...else Statement
- Java Ternary Operator
- Java for Loop
- Java for-each Loop
- Java while and do...while Loop
- Java break Statement
- Java continue Statement
- Java switch Statement
- Java Arrays
- Java Multidimensional Arrays
- Java Copy Arrays
Java OOP(I)
- Java Class and Objects
- Java Methods
- Java Method Overloading
- Java Constructors
- Java Static Keyword
- Java Strings
- Java Access Modifiers
- Java this Keyword
- Java final keyword
- Java Recursion
- Java instanceof Operator
Java OOP(II)
- Java Inheritance
- Java Method Overriding
- Java Abstract Class and Abstract Methods
- Java Interface
- Java Polymorphism
- Java Encapsulation
Java OOP(III)
- Java Nested and Inner Class
- Java Nested Static Class
- Java Anonymous Class
- Java Singleton Class
- Java enum Constructor
- Java enum Strings
- Java Reflection
- Java Package
- Java Exception Handling
- Java Exceptions
- Java try...catch
- Java throw and throws
- Java catch Multiple Exceptions
- Java try-with-resources
- Java Annotations
- Java Annotation Types
- Java Logging
- Java Assertions
- Java Collections Framework
- Java Collection Interface
- Java ArrayList
- Java Vector
- Java Stack Class
- Java Queue Interface
- Java PriorityQueue
- Java Deque Interface
- Java LinkedList
- Java ArrayDeque
- Java BlockingQueue
- Java ArrayBlockingQueue
- Java LinkedBlockingQueue
- Java Map Interface
- Java HashMap
- Java LinkedHashMap
- Java WeakHashMap
- Java EnumMap
- Java SortedMap Interface
- Java NavigableMap Interface
- Java TreeMap
- Java ConcurrentMap Interface
- Java ConcurrentHashMap
- Java Set Interface
- Java HashSet Class
- Java EnumSet
- Java LinkedHashSet
- Java SortedSet Interface
- Java NavigableSet Interface
- Java TreeSet
- Java Algorithms
- Java Iterator Interface
- Java ListIterator Interface
Java I/o Streams
- Java I/O Streams
- Java InputStream Class
- Java OutputStream Class
- Java FileInputStream Class
- Java FileOutputStream Class
- Java ByteArrayInputStream Class
- Java ByteArrayOutputStream Class
- Java ObjectInputStream Class
- Java ObjectOutputStream Class
- Java BufferedInputStream Class
- Java BufferedOutputStream Class
- Java PrintStream Class
Java Reader/Writer
- Java File Class
- Java Reader Class
- Java Writer Class
- Java InputStreamReader Class
- Java OutputStreamWriter Class
- Java FileReader Class
- Java FileWriter Class
- Java BufferedReader
- Java BufferedWriter Class
- Java StringReader Class
- Java StringWriter Class
- Java PrintWriter Class
Additional Topics
- Java Keywords and Identifiers
- Java Operator Precedence
- Java Bitwise and Shift Operators
- Java Scanner Class
- Java Type Casting
- Java Wrapper Class
- Java autoboxing and unboxing
- Java Lambda Expressions
- Java Generics
- Nested Loop in Java
- Java Command-Line Arguments
Java Tutorials
Java Math sqrt()
Java Math acos()
Java Math atan()
Java Math tan()
A method is a block of code that performs a specific task.
Suppose you need to create a program to create a circle and color it. You can create two methods to solve this problem:
- a method to draw the circle
- a method to color the circle
Dividing a complex problem into smaller chunks makes your program easy to understand and reusable.
In Java, there are two types of methods:
- User-defined Methods : We can create our own method based on our requirements.
- Standard Library Methods : These are built-in methods in Java that are available to use.
Let's first learn about user-defined methods.
- Declaring a Java Method
The syntax to declare a method is:
- returnType - It specifies what type of value a method returns For example if a method has an int return type then it returns an integer value. If the method does not return a value, its return type is void .
- methodName - It is an identifier that is used to refer to the particular method in a program.
- method body - It includes the programming statements that are used to perform some tasks. The method body is enclosed inside the curly braces { } .
For example,
In the above example, the name of the method is adddNumbers() . And, the return type is int . We will learn more about return types later in this tutorial.
This is the simple syntax of declaring a method. However, the complete syntax of declaring a method is
- modifier - It defines access types whether the method is public, private, and so on. To learn more, visit Java Access Specifier .
- static - If we use the static keyword, it can be accessed without creating objects. For example, the sqrt() method of standard Math class is static. Hence, we can directly call Math.sqrt() without creating an instance of Math class.
- parameter1/parameter2 - These are values passed to a method. We can pass any number of arguments to a method.
Calling a Method in Java
In the above example, we have declared a method named addNumbers() . Now, to use the method, we need to call it.
Here's is how we can call the addNumbers() method.
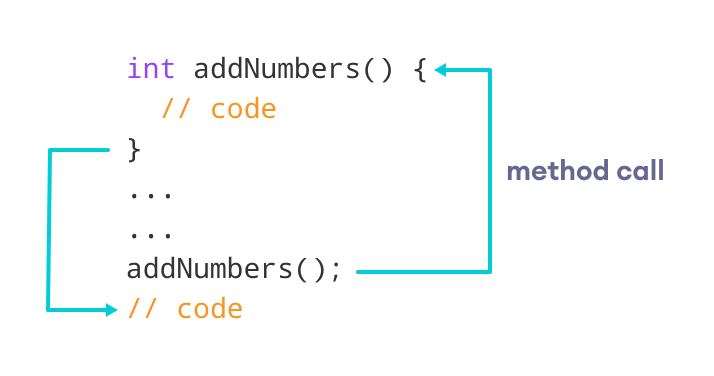
Example 1: Java Methods
In the above example, we have created a method named addNumbers() . The method takes two parameters a and b . Notice the line,
Here, we have called the method by passing two arguments num1 and num2 . Since the method is returning some value, we have stored the value in the result variable.
Note : The method is not static. Hence, we are calling the method using the object of the class.
- Java Method Return Type
A Java method may or may not return a value to the function call. We use the return statement to return any value. For example,
Here, we are returning the variable sum . Since the return type of the function is int . The sum variable should be of int type. Otherwise, it will generate an error.
Example 2: Method Return Type
In the above program, we have created a method named square() . The method takes a number as its parameter and returns the square of the number.
Here, we have mentioned the return type of the method as int . Hence, the method should always return an integer value.
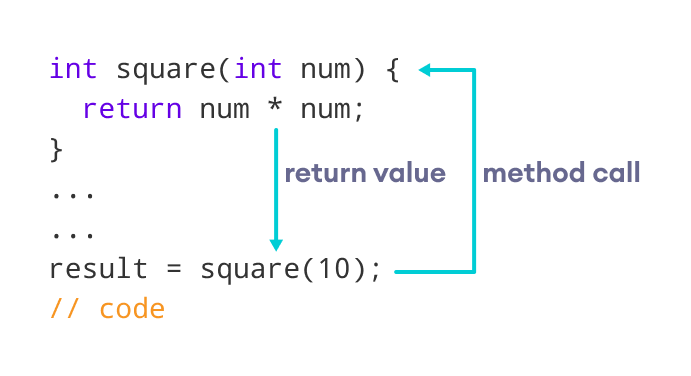
Note : If the method does not return any value, we use the void keyword as the return type of the method. For example,
Method Parameters in Java
A method parameter is a value accepted by the method. As mentioned earlier, a method can also have any number of parameters. For example,
If a method is created with parameters, we need to pass the corresponding values while calling the method. For example,
Example 3: Method Parameters
Here, the parameter of the method is int . Hence, if we pass any other data type instead of int , the compiler will throw an error. It is because Java is a strongly typed language.
Note : The argument 24 passed to the display2() method during the method call is called the actual argument.
The parameter num accepted by the method definition is known as a formal argument. We need to specify the type of formal arguments. And, the type of actual arguments and formal arguments should always match.
- Standard Library Methods
The standard library methods are built-in methods in Java that are readily available for use. These standard libraries come along with the Java Class Library (JCL) in a Java archive (*.jar) file with JVM and JRE.
- print() is a method of java.io.PrintSteam . The print("...") method prints the string inside quotation marks.
- sqrt() is a method of Math class. It returns the square root of a number.
Here's a working example:
Example 4: Java Standard Library Method
To learn more about standard library methods, visit Java Library Methods .
What are the advantages of using methods?
1. The main advantage is code reusability . We can write a method once, and use it multiple times. We do not have to rewrite the entire code each time. Think of it as, "write once, reuse multiple times".
Example 5: Java Method for Code Reusability
In the above program, we have created the method named getSquare() to calculate the square of a number. Here, the method is used to calculate the square of numbers less than 6 .
Hence, the same method is used again and again.
2. Methods make code more readable and easier to debug. Here, the getSquare() method keeps the code to compute the square in a block. Hence, makes it more readable.
Table of Contents
- Calling a Java Method
- Method Parameters
- Advantages of Java Methods
Sorry about that.
Related Tutorials
Java Library
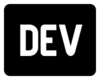
DEV Community

Posted on May 3, 2019 • Updated on May 8, 2019
Writing Good Method & Variable Names
What's in a name.
In Object Oriented Programming it's considered to be good practice to write methods that only do one major job. One strategy you can use to ensure that your methods follow this practice is to write method names that describe what the method does. "Clean Code" by Robert C. Martin shares the following old but sound advice: "Functions should do one thing. They should do it well. They should do it only."
Sometimes it can be hard to tell whether the method only does one thing; it's at those times that I turn to the name for assistance. Can I write a descriptive name without the use of "and" or "or"? If the answer is no, it's possible that the method has multiple things going on, but a yes answer means I'm safe (or not being descriptive enough in my naming). It's okay if method names are long - Martin states that "A long descriptive name is better than a short enigmatic name." It's also good convention to use similar verbage for actions taken or abstract concepts such as get whenever retrieving a value, set whenever setting a value, or write whenever writing something to a file.
A few examples of good descriptive method names:
- WriteToOrderXmlFile()
- CreateNewCustomer()
- CreateDeduplicatedListOfShippingContacts()
- GetListOfPartProductsNotInSalesforce()
- CreateNewPartProduct()
Whatever you do, don't make the mistake of using a method name like DoStuff() to get started, then forget to go back and update it! If you can't think of a descriptive name, you just need to do a little more conceptualization first.
Don't be afraid to use multiple words to get your point across because a descriptive name can act as a replacement for a long descriptive comment (and won't require extra documentation updates if the method changes!) It should be clear to future you and any developers who come after you what each method does before they've even inspected it. Once they have gone to the method, it should do everything they would have expected from the name.
Class and variable names won't necessarily be as long, but they are no less important for clarity and readability. These names should be nouns such as Customer() or ShippedPartProduct() , and string partIdCSV or int customerAge . I usually choose to make List and Array names longer so I can better describe whatever set of data it's holding such as partProductsRetrievedFromSalesforce or itemIdsFromThisShipment .
Variable and class names that are unacceptable:
- int x = 42;
- bool trueFalse = false;
- var object = "xyz";
- HelperClass()
Strategies To Help
I have developed a few strategies that help me with both good naming and ensuring my methods are all short and perform only one major function.
The first strategy seems like a no-brainer, but it took me awhile to actually figure it out:
Don't break out code into new methods until a full portion of code has you satisfied By holding off on breaking out code until you've completed one or several portions, you allow yourself to essentially finish your thought process before moving on to the next thing. Much like you might refine an article after you've written your first draft, you can get your ideas out there and refine them into more suitably-formed sentences and paragraphs (e.g. methods) before releasing it into the wild. You also open yourself up to seeing the whole picture which means you have the opportunity to see where the most optimal splits can be instead of trying to conceptualize the splits as you're working through how to solve the issue.
Typically I will start with one method and have it named according to what it should do. I'll write all of the supporting code within this method, even though it isn't part of its job, and run my tests and whatnot. Once I'm satisfied with the results, I will look at each portion of the code I've written and break it apart into its bite-sized method portions. These will either be called by the method I wrote them in, or I'll move them out to be called from a position before or after the method I originally wrote the code in. Then I will run through my tests again, just to make sure the changes didn't break anything unexpectedly.
Consider whether you have multiple similar data sources Are you using a database and another source of data such as Salesforce within your application? I like to indicate source of data in my variables because it's not uncommon that I'll be using an ItemID from our Item table as well as the ItemID from a Salesforce object. These two variables could have very different values, so it's important to keep them straight. A great example from my current code base would be partProductSerialNumbersFromSalesforce and partProductSerialNumbersFromDB . These lists will hold two different sets of potentially the same stored values - I compare them to determine whether a specific part in our database already exists in Salesforce to avoid creating duplicates.
I don't know whether this strategy is a best practice, but I will also occasionally include the actual table or stored procedure name if I am worried about any ambiguity. An example could be an application that updates two similar tables (let's say Shipments and ShipmentLines); these tables both have a UserDef field that must be updated and you want to avoid ambiguity in which one is being updated when. UpdateShipmentTableUserDef() and UpdateShipmentLinesUserDef() seem like perfectly reasonable and clear names for the methods that will update those fields. Obviously this would be overly wordy and unnecessary for most database updates, but I feel it certainly helps in the odd case like the example.
Is your method returning something? In the case of a method that returns something, I opt toward using names that describe whatever is being returned. If I'm returning a comma separated string of item Ids, I may choose a name like CommaSeparatedPartProductItemIds() ; or GetPartAccountIdFromSalesforce() if I'm running a query that returns the id of the Salesforce Account associated with a part. The important part is to indicate to the reader what the expected value being returned should be. If they're expecting an itemId and get back a customer name, they have a good idea where to start looking for that bug.
Grammar Lesson
Recently fellow dev.to writer @somedood released an article describing the details of naming grammar - essentially when you should be using camelCase, PascalCase, or SCREAMING_CASE. Check it out here:

A Grammar-Based Naming Convention
Did i miss anything.
Do you have any awesome ways you make sure your names tell the story of your code? Any techniques to ensure good readability for those who may come behind you? I'd love to hear your own favorite naming conventions.
I'd also love to hear the worst names you've ever seen. I'm sure there's some funny ones out there!
Top comments (23)
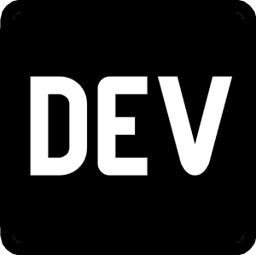
Templates let you quickly answer FAQs or store snippets for re-use.

- Email [email protected]
- Location Buffalo, NY
- Work Senior Software Developer
- Joined Sep 11, 2016
Loved this, thank you!
I've been ranting about a method I found in one of our old libraries for the last couple of weeks.
GetIdById() , really? What the heck is that supposed to even do?
After digging into it and figuring out what it did I eventually renamed it to GetPatientIdByChartId()

- Location Europe
- Joined Oct 28, 2018
You write that it is a method, not a standalone function. So it belongs to an object, probably an object of type Patient . So what about calling it GetIdByChartId() ? It makes no sense to put information into a name that is obvious from the context.
Because it was a standalone function. I tend to use method and function interchangeably when I really shouldn't.

- Location Fort Worth, TX
- Education Oregon Institute of Technology
- Work Software Developer at BOXX Technologies
- Joined Dec 18, 2018
Your rename makes a lot more sense than the original. "GetIdById" sounds like the code equivalent of trying to divide something by zero if you ask me!

- Location charlotte, nc
- Work software developer at vectorvest
- Joined Apr 25, 2019
Ha. I wish it had just contained

- Location Vancouver
- Work Senior Software Engineer
- Joined Jan 21, 2019
There's the industry-standard Javascript hack…
var that = this;

- Joined Aug 13, 2018
I sometimes encounter var self = this; too, kind of annoying. I prefer to use myFunction() { /* much stuff, very algorithm */}.bind(this) if I am in an event handler for example, much natural to work with this even if the bind is a bit ugly!
haha "much stuff, very algorithm" :)
Thankfully I don't need to deal with this very often at this point, but I agree with you. I'll keep this style in mind and try to remember to use it whenever I encounter it again!
I think, at my current level, I'd probably just sit there and cry (or laugh maniacally) for a few minutes if I came across this.

- Work Developer
- Joined May 3, 2019
I once implemented model class Room, with supported classes RoomView and of course RoomService :-) Nice article by the way, only thing I do not like in examples here is to use "list" or any other data typ word in names. I prefer simply to use plural nouns. So instead of getListOfProducts simply getProducts.

- Location CO, USA
- Education B.S. C.S.
- Pronouns They/Them
- Work Developer Advocate at Apollo GraphQL
- Joined Apr 15, 2019
I can see using the data type in the name for some weakly typed languages. Like if you’re not on the latest Python and type annotations are a pain, it’s nice to be able to see what you’re doing.
I have no excuse, I almost exclusively write in C#. sheepish grin
True, you're right. Using the variable will show clearly that it's a list - it's probably a bit redunant. Thank you!

- Email [email protected]
- Joined Apr 11, 2018
One of my favorites is when we spread a single giant method across multiple methods so that the names are UpdateCustomer , ExecuteUpdateCustomer , DoExecuteUpdateCustomer , and even PrepareToUpdateCustomer .

- Location Delhi,India
- Education Bachelor of engineering
- Work Software engineer
- Joined May 31, 2020
Great article. Curious about one thing. Have one another question. For example : getUserNearestLocation(user) by going through it we can say it this function is going to return user location based on user information. If suppose if I have to add another parameter in function let's say hospital getUserNearestLocation(user,hospital) now context has change name should be getUserNearestHospitalLocation . My question is that if tomorrow I have 10 parameters. Then this function name might be very big if I keep adding parameter. Eventually function is return userLocation based on parameter. In those cases what are good naming convention?

- Location Athens, Greece
- Work Software Engineer at SciFY
- Joined Oct 4, 2017
Totally agree with your point. Taking a step back and putting some thought on how to name a variable or a method can help your app have more SOLID foundations and save you hours of refactoring.
I feel like it saves me a lot of time when I do small refactoring as I go instead of waiting until I have a 6000 line method to break apart all at once. (not to mention the time saved debugging when the only clue you have is "thing broke in main()"
I am not talking only about breking long methods to smaller ones, but conceptual refactoring when trying to name a variable/method. Often times I find myself struggling with coming up with a name, only to end up deciding that my method needs to be a part of a better designed class (or to be extracted to a new class), even before start writing it 😃
I saw a great one in a meeting today and had to share:
very.long.name.to.work.around.bug();
Naming is so hard. I'm also much better at pointing out problems in other developer's names in a PR than I am at naming things myself. 😀

- Email [email protected]
- Location Turkey
- Education Web Dev
- Work Jr. Fullstack Developer at FOSS Dev
- Joined Nov 21, 2017
What if the class name would be ”Customer” and its method name would be ”create”.
Is it good,
I think a case like this would actually make sense, because I assume you'd do something like Customer customer = new Customer(); to instantiate your class... then you would be doing customer.create() whenever you called that create method. I hadn't considered the combination of classes and their methods - you made a really good point! Thank you!
Are you sure you want to hide this comment? It will become hidden in your post, but will still be visible via the comment's permalink .
Hide child comments as well
For further actions, you may consider blocking this person and/or reporting abuse
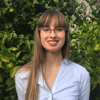
A Software Engineer's Tips and Tricks #2: Template Databases in PostgreSQL
alisdairbr - Apr 30
Powerful Practices Every New Developer Should Adopt
Baransel - May 13

5 Tips to improve your logic building in Programming.
Kachkol Asa - May 4
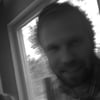
Constructors in Go?
Stefan Alfbo - Apr 29
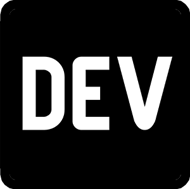
We're a place where coders share, stay up-to-date and grow their careers.
- Java Arrays
- Java Strings
- Java Collection
- Java 8 Tutorial
- Java Multithreading
- Java Exception Handling
- Java Programs
- Java Project
- Java Collections Interview
- Java Interview Questions
- Spring Boot
- Java Tutorial
Overview of Java
- Introduction to Java
- The Complete History of Java Programming Language
- C++ vs Java vs Python
- How to Download and Install Java for 64 bit machine?
- Setting up the environment in Java
- How to Download and Install Eclipse on Windows?
- JDK in Java
- How JVM Works - JVM Architecture?
- Differences between JDK, JRE and JVM
- Just In Time Compiler
- Difference between JIT and JVM in Java
- Difference between Byte Code and Machine Code
- How is Java platform independent?
Basics of Java
- Java Basic Syntax
- Java Hello World Program
- Java Data Types
- Primitive data type vs. Object data type in Java with Examples
- Java Identifiers
Operators in Java
- Java Variables
- Scope of Variables In Java
Wrapper Classes in Java
Input/output in java.
- How to Take Input From User in Java?
- Scanner Class in Java
- Java.io.BufferedReader Class in Java
- Difference Between Scanner and BufferedReader Class in Java
- Ways to read input from console in Java
- System.out.println in Java
- Difference between print() and println() in Java
- Formatted Output in Java using printf()
- Fast I/O in Java in Competitive Programming
Flow Control in Java
- Decision Making in Java (if, if-else, switch, break, continue, jump)
- Java if statement with Examples
- Java if-else
- Java if-else-if ladder with Examples
- Loops in Java
- For Loop in Java
- Java while loop with Examples
- Java do-while loop with Examples
- For-each loop in Java
- Continue Statement in Java
- Break statement in Java
- Usage of Break keyword in Java
- return keyword in Java
- Java Arithmetic Operators with Examples
- Java Unary Operator with Examples
- Java Assignment Operators with Examples
- Java Relational Operators with Examples
- Java Logical Operators with Examples
- Java Ternary Operator with Examples
- Bitwise Operators in Java
- Strings in Java
- String class in Java
- Java.lang.String class in Java | Set 2
- Why Java Strings are Immutable?
- StringBuffer class in Java
- StringBuilder Class in Java with Examples
- String vs StringBuilder vs StringBuffer in Java
- StringTokenizer Class in Java
- StringTokenizer Methods in Java with Examples | Set 2
- StringJoiner Class in Java
- Arrays in Java
- Arrays class in Java
- Multidimensional Arrays in Java
- Different Ways To Declare And Initialize 2-D Array in Java
- Jagged Array in Java
- Final Arrays in Java
- Reflection Array Class in Java
- util.Arrays vs reflect.Array in Java with Examples
OOPS in Java
- Object Oriented Programming (OOPs) Concept in Java
- Why Java is not a purely Object-Oriented Language?
- Classes and Objects in Java
Naming Conventions in Java
- Java Methods
Access Modifiers in Java
- Java Constructors
- Four Main Object Oriented Programming Concepts of Java
Inheritance in Java
Abstraction in java, encapsulation in java, polymorphism in java, interfaces in java.
- 'this' reference in Java
- Inheritance and Constructors in Java
- Java and Multiple Inheritance
- Interfaces and Inheritance in Java
- Association, Composition and Aggregation in Java
- Comparison of Inheritance in C++ and Java
- abstract keyword in java
- Abstract Class in Java
- Difference between Abstract Class and Interface in Java
- Control Abstraction in Java with Examples
- Difference Between Data Hiding and Abstraction in Java
- Difference between Abstraction and Encapsulation in Java with Examples
- Difference between Inheritance and Polymorphism
- Dynamic Method Dispatch or Runtime Polymorphism in Java
- Difference between Compile-time and Run-time Polymorphism in Java
Constructors in Java
- Copy Constructor in Java
- Constructor Overloading in Java
- Constructor Chaining In Java with Examples
- Private Constructors and Singleton Classes in Java
Methods in Java
- Static methods vs Instance methods in Java
- Abstract Method in Java with Examples
- Overriding in Java
- Method Overloading in Java
- Difference Between Method Overloading and Method Overriding in Java
- Differences between Interface and Class in Java
- Functional Interfaces in Java
- Nested Interface in Java
- Marker interface in Java
- Comparator Interface in Java with Examples
- Need of Wrapper Classes in Java
- Different Ways to Create the Instances of Wrapper Classes in Java
- Character Class in Java
- Java.Lang.Byte class in Java
- Java.Lang.Short class in Java
- Java.lang.Integer class in Java
- Java.Lang.Long class in Java
- Java.Lang.Float class in Java
- Java.Lang.Double Class in Java
- Java.lang.Boolean Class in Java
- Autoboxing and Unboxing in Java
- Type conversion in Java with Examples
Keywords in Java
- Java Keywords
- Important Keywords in Java
- Super Keyword in Java
- final Keyword in Java
- static Keyword in Java
- enum in Java
- transient keyword in Java
- volatile Keyword in Java
- final, finally and finalize in Java
- Public vs Protected vs Package vs Private Access Modifier in Java
- Access and Non Access Modifiers in Java
Memory Allocation in Java
- Java Memory Management
- How are Java objects stored in memory?
- Stack vs Heap Memory Allocation
- How many types of memory areas are allocated by JVM?
- Garbage Collection in Java
- Types of JVM Garbage Collectors in Java with implementation details
- Memory leaks in Java
- Java Virtual Machine (JVM) Stack Area
Classes of Java
- Understanding Classes and Objects in Java
- Singleton Method Design Pattern in Java
- Object Class in Java
- Inner Class in Java
- Throwable Class in Java with Examples
Packages in Java
- Packages In Java
- How to Create a Package in Java?
- Java.util Package in Java
- Java.lang package in Java
- Java.io Package in Java
- Java Collection Tutorial
Exception Handling in Java
- Exceptions in Java
- Types of Exception in Java with Examples
- Checked vs Unchecked Exceptions in Java
- Java Try Catch Block
- Flow control in try catch finally in Java
- throw and throws in Java
- User-defined Custom Exception in Java
- Chained Exceptions in Java
- Null Pointer Exception In Java
- Exception Handling with Method Overriding in Java
- Multithreading in Java
- Lifecycle and States of a Thread in Java
- Java Thread Priority in Multithreading
- Main thread in Java
- Java.lang.Thread Class in Java
- Runnable interface in Java
- Naming a thread and fetching name of current thread in Java
- What does start() function do in multithreading in Java?
- Difference between Thread.start() and Thread.run() in Java
- Thread.sleep() Method in Java With Examples
- Synchronization in Java
- Importance of Thread Synchronization in Java
- Method and Block Synchronization in Java
- Lock framework vs Thread synchronization in Java
- Difference Between Atomic, Volatile and Synchronized in Java
- Deadlock in Java Multithreading
- Deadlock Prevention And Avoidance
- Difference Between Lock and Monitor in Java Concurrency
- Reentrant Lock in Java
File Handling in Java
- Java.io.File Class in Java
- Java Program to Create a New File
- Different ways of Reading a text file in Java
- Java Program to Write into a File
- Delete a File Using Java
- File Permissions in Java
- FileWriter Class in Java
- Java.io.FileDescriptor in Java
- Java.io.RandomAccessFile Class Method | Set 1
- Regular Expressions in Java
- Regex Tutorial - How to write Regular Expressions?
- Matcher pattern() method in Java with Examples
- Pattern pattern() method in Java with Examples
- Quantifiers in Java
- java.lang.Character class methods | Set 1
- Java IO : Input-output in Java with Examples
- Java.io.Reader class in Java
- Java.io.Writer Class in Java
- Java.io.FileInputStream Class in Java
- FileOutputStream in Java
- Java.io.BufferedOutputStream class in Java
- Java Networking
- TCP/IP Model
- User Datagram Protocol (UDP)
- Differences between IPv4 and IPv6
- Difference between Connection-oriented and Connection-less Services
- Socket Programming in Java
- java.net.ServerSocket Class in Java
- URL Class in Java with Examples
JDBC - Java Database Connectivity
- Introduction to JDBC (Java Database Connectivity)
- JDBC Drivers
- Establishing JDBC Connection in Java
- Types of Statements in JDBC
- JDBC Tutorial
- Java 8 Features - Complete Tutorial
A programmer is always said to write clean codes, where naming has to be appropriate so that for any other programmer it acts as an easy way out to read the code. At a smaller level, this seems meaningless but think of the industrial level where it becomes necessary to write clean codes in order to save time for which there are certain rules been laid of which one of the factors is to name the keyword right which is termed as a naming convention in Java.
For example when you are using a variable name depicting displacement then it should be named as “displace” or similar likewise not likely x, d which becomes complex as the code widens up and decreases the readability aperture. Consider the below illustrations to get a better understanding which later on we will be discussing in detail.
Illustrations:
- Class: If you are naming any class then it should be a noun and so should be named as per the goal to be achieved in the program such as Add2Numbers, ReverseString, and so on not likely A1, Programming, etc. It should be specific pointing what exactly is there inside without glancing at the body of the class.
- Interface: If you are naming an interface, it should look like an adjective such as consider the existing ones: Runnable, Serializable, etc. Try to use ‘able’ at the end, yes it is said to try as there are no hard and fast bound rules as if we do consider an inbuilt interface such as ‘Remote’, it is not having ble at the end. Consider if you are supposed to create an interface to make read operation then it is suggested as per naming conventions in java to name a similar likely ‘Readable’ interface.
- Methods: Now if we do look closer a method is supposed to do something that it does contains in its body henceforth it should be a verb.
- Constants: As the name suggests it should look like as we read it looks like it is fixed for examples PI, MAX_INT, MIN_INT, etc as follows.
Naming Conventions in Java
In java, it is good practice to name class, variables, and methods name as what they are actually supposed to do instead of naming them randomly. Below are some naming conventions of the java programming language. They must be followed while developing software in java for good maintenance and readability of code. Java uses CamelCase as a practice for writing names of methods, variables, classes, packages, and constants.
Camel’s case in java programming consists of compound words or phrases such that each word or abbreviation begins with a capital letter or first word with a lowercase letter, rest all with capital. Here in simpler terms, it means if there are two
Note: Do look out for these exceptions cases to camel casing in java as follows: In package, everything is small even while we are combining two or more words in java In constants, we do use everything as uppercase and only ‘_’ character is used even if we are combining two or more words in java.
Type 1: Classes and Interfaces
- Class names should be nouns , in mixed cases with the first letter of each internal word capitalized. Interfaces names should also be capitalized just like class names.
- Use whole words and must avoid acronyms and abbreviations.
Type 2: Methods
- Methods should be verbs , in mixed case with the first letter lowercase and with the first letter of each internal word capitalized.
As the name suggests the method is supposed to be primarily method which indeed it is as main() method in java is the method from where the program begins its execution.
Type 3: Variables
Variable names should be short yet meaningful.
- Should be mnemonic i.e, designed to indicate to the casual observer the intent of its use.
- One-character variable names should be avoided except for temporary variables.
- Common names for temporary variables are i, j, k, m, and n for integers; c, d, and e for characters.
As the name suggests one stands for marks while the other for an answer be it of any e do not mind.
Type 4: Constant variables
- Should be all uppercase with words separated by underscores (“_”).
- There are various constants used in predefined classes like Float, Long, String etc.
Type 5: Packages
- The prefix of a unique package name is always written in all-lowercase ASCII letters and should be one of the top-level domain names, like com, edu, gov, mil, net, org.
- Subsequent components of the package name vary according to an organization’s own internal naming conventions.
As the name suggests in the first case we are trying to access the Scanner class from the java.util package and in other all classes(* standing for all) input-output classes making it so easy for another programmer to identify.
Note: For class and interfaces, the first letter has to be uppercase. For method , variable, package_name, and constants, the first letter has to be lowercase.
Please Login to comment...
Similar reads.
- School Programming
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
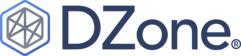
- Manage Email Subscriptions
- How to Post to DZone
- Article Submission Guidelines
- Manage My Drafts
Data Engineering: Work with DBs? Build data pipelines? Or maybe you're exploring AI-driven data capabilities? We want to hear your insights.
Modern API Management : Dive into APIs’ growing influence across domains, prevalent paradigms, microservices, the role AI plays, and more.
Programming in Python: Dive into the Python ecosystem to learn about popular libraries, tools, modules, and more.
PostgreSQL: Learn about the open-source RDBMS' advanced capabilities, core components, common commands and functions, and general DBA tasks.
- Mastering Concurrency: An In-Depth Guide to Java's ExecutorService
- Increase Your Code Quality in Java by Exploring the Power of Javadoc
- Providing Enum Consistency Between Application and Data
- Ultra-Fast Microservices: When MicroStream Meets Helidon
- API Gateway vs. Load Balancer
- Expose Your Database as a REST API Safely and Without Code
- Instant APIs With Copilot and API Logic Server
- Going with the Flow for CI/CD: Heroku Flow With Gitflow
- Data Engineering
Best Practices for Variable and Method Naming
Join the DZone community and get the full member experience.
- Use short enough and long enough variable names in each scope of code. Generally length may be 1 char for loop counters, 1 word for condition/loop variables, 1-2 words for methods, 2-3 words for classes, 3-4 words for globals.
- Use specific names for variables, for example "value", "equals", "data", ... are not valid names for any case.
- Use meaningful names for variables. Variable name must define the exact explanation of its content.
- Don't start variables with o_, obj_, m_ etc. A variable does not need tags which states it is a variable.
- Obey company naming standards and write variable names consistently in application: e.g. txtUserName, lblUserName, cmbSchoolType, ... Otherwise readability will reduce and find/replace tools will be unusable.
- use Camel Case (aka Upper Camel Case) for classes: VelocityResponseWriter
- use Lower Case for packages: com.company.project.ui
- use Mixed Case (aka Lower Camel Case) for variables: studentName
- use Upper Case for constants : MAX_PARAMETER_COUNT = 100
- use Camel Case for enum class names and Upper Case for enum values.
- don't use '_' anywhere except constants and enum values (which are constants).
- For example for Java,
- Don't reuse same variable name in the same class in different contexts: e.g. in method, constructor, class. So you can provide more simplicity for understandability and maintainability.
- Don't use same variable for different purposes in a method, conditional etc. Create a new and different named variable instead. This is also important for maintainability and readability.
- Don't use non-ASCII chars in variable names. Those may run on your platform but may not on others.
- Don't use too long variable names (e.g. 50 chars). Long names will bring ugly and hard-to-read code, also may not run on some compilers because of character limit.
- Decide and use one natural language for naming, e.g. using mixed English and German names will be inconsistent and unreadable.
- Use meaningful names for methods. The name must specify the exact action of the method and for most cases must start with a verb. (e.g. createPasswordHash)
- Obey company naming standards and write method names consistently in application: e.g. getTxtUserName(), getLblUserName(), isStudentApproved(), ... Otherwise readability will reduce and find/replace tools will be unusable.
- use Mixed Case for method names: getStudentSchoolType
- use Mixed Case for method parameters: setSchoolName(String schoolName)
- Use meaningful names for method parameters, so it can documentate itself in case of no documentation.
Opinions expressed by DZone contributors are their own.
Partner Resources
- About DZone
- Send feedback
- Community research
- Advertise with DZone
CONTRIBUTE ON DZONE
- Become a Contributor
- Core Program
- Visit the Writers' Zone
- Terms of Service
- Privacy Policy
- 3343 Perimeter Hill Drive
- Nashville, TN 37211
- [email protected]
Let's be friends:

- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
How do I write method names in Java?
While writing a method name we should follow the camel case i.e. first letter of the first word should be small and the first letters of the remaining (later) words should be capital.

Related Articles
- How do I write class names in Java?
- How do I write variable names in Java?
- How do I write constructor names in Java?
- How do I write interface names in Java?
- How do I write package names in Java?
- How do I write constants names in Java?
- How do I time a method execution in Java
- How do I write JSON in Python?
- How do I search for names starting with A in MySQL?
- How do you write a method in Java that can print object in array?
- How do I write CSS within HTML?
- How do I cache method calls in Python?
- How do I create dynamic variable names inside a JavaScript loop?
- How do I invoke a Java method when given the method name as a string?
- How do I compare strings in Java?
Kickstart Your Career
Get certified by completing the course
To Continue Learning Please Login
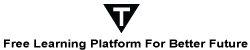

Java Object Class
Java inheritance, java polymorphism, java abstraction, java encapsulation, java oops misc.
CamelCase in Java naming conventions
Java follows camel-case syntax for naming the class, interface, method, and variable.
If the name is combined with two words, the second word will start with uppercase letter always such as actionPerformed(), firstName, ActionEvent, ActionListener, etc.

- Send your Feedback to [email protected]
Help Others, Please Share

Learn Latest Tutorials

Transact-SQL

Reinforcement Learning

R Programming

React Native

Python Design Patterns

Python Pillow

Python Turtle

Preparation

Verbal Ability

Interview Questions

Company Questions
Trending Technologies

Artificial Intelligence

Cloud Computing

Data Science

Machine Learning

B.Tech / MCA

Data Structures

Operating System

Computer Network

Compiler Design

Computer Organization

Discrete Mathematics

Ethical Hacking

Computer Graphics

Software Engineering

Web Technology

Cyber Security

C Programming

Control System

Data Mining

Data Warehouse

Java Guides
Search this blog, spring data jpa - query creation from method names, supported keywords inside method names.
Rules for Creating Query Methods
1. Creating Spring Boot Project
Spring boot provides a web tool called https://start.spring.io to bootstrap an application quickly. just go to https://start.spring.io and generate a new spring boot project. use the below details in the spring boot creation: project name: spring-data-jpa-course project type: maven choose dependencies: spring data jpa, mysql driver, lombok package name: net.javaguides.springboot, 2. maven dependencies, here is the complete pom.xml for your reference: <xml version="1.0" encoding="utf-8"> <project xmlns="http://maven.apache.org/pom/4.0.0" xmlns:xsi="http://www.w3.org/2001/xmlschema-instance" xsi:schemalocation="http://maven.apache.org/pom/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelversion>4.0.0</modelversion> <parent> <groupid>org.springframework.boot</groupid> <artifactid>spring-boot-starter-parent</artifactid> <version>3.0.4</version> <relativepath/> <-- lookup parent from repository --> </parent> <groupid>net.javaguides</groupid> <artifactid>spring-data-jpa-course</artifactid> <version>0.0.1-snapshot</version> <name>spring-data-jpa-course</name> <description>demo project for spring boot</description> <properties> <java.version>17</java.version> </properties> <dependencies> <dependency> <groupid>org.springframework.boot</groupid> <artifactid>spring-boot-starter-data-jpa</artifactid> </dependency> <dependency> <groupid>com.mysql</groupid> <artifactid>mysql-connector-j</artifactid> <scope>runtime</scope> </dependency> <dependency> <groupid>org.springframework.boot</groupid> <artifactid>spring-boot-starter-test</artifactid> <scope>test</scope> </dependency> </dependencies> <build> <plugins> <plugin> <groupid>org.springframework.boot</groupid> <artifactid>spring-boot-maven-plugin</artifactid> </plugin> </plugins> </build> </project>, 3. configure mysql database, let's use the mysql database to store and retrieve the data in this example and we gonna use hibernate properties to create and drop tables. open the application.properties file and add the following configuration to it: spring.datasource.url =jdbc:mysql://localhost: 3306 /demousessl= false spring.datasource.username =root spring.datasource.password =mysql@ 123 spring.jpa.properties.hibernate.dialect = org.hibernate.dialect.mysqldialect spring.jpa.hibernate.ddl-auto = create-drop make sure that you will create a demo database before running the spring boot application. also, change the mysql username and password as per your mysql installation on your machine., 4. create jpa entity - product.java, let's create an entity package inside a base package " net.javaguides.springboot ". within the entity package, create a product class with the following content: import lombok.*; import org.hibernate.annotations.creationtimestamp; import org.hibernate.annotations.updatetimestamp; import jakarta.persistence.*; import java.math.bigdecimal; import java.time.localdatetime; @entity @getter @setter @noargsconstructor @allargsconstructor @tostring @table( name = "products", schema = "ecommerce", uniqueconstraints = { @uniqueconstraint( name = "sku_unique", columnnames = "stock_keeping_unit" ) } ) public class product { @id @generatedvalue( strategy = generationtype.sequence, generator = "product_generator" ) @sequencegenerator( name = "product_generator", sequencename = "product_sequence_name", allocationsize = 1 ) private long id; @column(name = "stock_keeping_unit", nullable = false) private string sku; @column(nullable = false) private string name; private string description; private bigdecimal price; private boolean active; private string imageurl; @creationtimestamp private localdatetime datecreated; @updatetimestamp private localdatetime lastupdated; } note that we are using lombok annotations to reduce the boilerplate code., 5. create spring data jpa repository, import com.springdatajpa.springboot.entity.product; import org.springframework.data.jpa.repository.jparepository; import org.springframework.data.jpa.repository.query; import org.springframework.data.repository.query.param; import java.math.bigdecimal; import java.time.localdatetime; import java.util.list; import java.util.optional; public interface productrepository extends jparepository<product, long> { /** * returns the found product entry by using its name as search * criteria. if no product entry is found, this method * returns null. */ public product findbyname(string name); /** * returns an optional which contains the found product * entry by using its id as search criteria. if no product entry * is found, this method returns an empty optional. */ optional<product> findbyid(long id); /** * returns the found list of product entries whose name or description is given * as a method parameters. if no product entries is found, this method * returns an empty list. */ list<product> findbynameordescription(string name, string description); /** * returns the found list of product entries whose name and description is given * as a method parameters. if no product entries is found, this method * returns an empty list. */ list<product> findbynameanddescription(string name, string description); /** * return the distinct product entry whose name is given as a method parameter * if no product entry is found, this method returns null. */ product finddistinctbyname(string name); /** * return the products whose price is greater than given price as method parameter * @param price * @return */ list<product> findbypricegreaterthan(bigdecimal price); /** * return the products whose price is less than given price as method parameter * @param price * @return */ list<product> findbypricelessthan(bigdecimal price); /** * return the filtered the product records that match the given text * @param name * @return */ list<product> findbynamecontaining(string name); /** * return products based on sql like condition * @param name * @return */ list<product> findbynamelike(string name); /** * returns a products whose price between start price and end price * @param startprice * @param endprice * @return */ list<product> findbypricebetween(bigdecimal startprice, bigdecimal endprice); /** * returns a products whose datecreated between start date and end date * @param startdate * @param enddate * @return */ list<product> findbydatecreatedbetween(localdatetime startdate, localdatetime enddate); } let's understand the query methods from the above productrepository interface. query method to find or retrieve a product by name:, /** * returns the found product entry by using its name as search * criteria. if no product entry is found, this method * returns null. */ public product findbyname(string name);.
Query method to find or retrieve a product by id:
Query method to find or retrieve a product by name or description:
Query method to find or retrieve a product by name and description:
Query method to find or retrieve a unique product by name:
Query method to find or retrieve products whose price is greater than the given price as a method parameter:
6. Test Spring Data JPA Query Methods
Now let's write a JUnit test case to test above all Spring Data JPA query methods:
JUnit Test for findByName Query Method:
Junit test for findbyid query method:, junit test for findbynameordescription query method:, junit test for findbynameanddescription query method:, junit test for finddistinctbyname query method:, junit test for findbypricegreaterthan query method:, junit test for findbypricelessthan query method:, junit test for findbynamecontaining query method:, junit test for findbynamelike query method:, junit test for findbypricebetween query method:, junit test for findbydatecreatedbetween query method:, 7. conclusion, 8. related spring data jpa tutorials and examples.
- Spring Data JPA Tutorial - Getting Started // Popular
- How to use Spring Data JPA in Spring Boot Project // Popular
- Spring Data JPA Tutorial - Java-Based Configuration
- Spring Data JPA - Query Creation from Method Names // Popular
- Spring Data JPA - Creating Database Queries With Named Queries
- Spring Data JPA @NamedNativeQuery and @NamedNativeQueries Example
- Spring Data JPA - @NamedQuery Example
- Spring Data JPA - @NamedQueries Example
- Spring Data JPA - Creating Database Queries using @Query Annotation
- Spring Data JPA - Auditing with Spring Boot 2 and MySQL Example // Popular
- Spring Data JPA - Working with Multiple Datasources using Spring Boot
- Spring @Repository Annotation
- Spring MVC 5 + Spring Data JPA + Hibernate 5 + JSP + MySQL Tutorial
- Spring Data REST Tutorial
- Spring Boot CRUD Tutorial with Spring MVC, Spring Data JPA, Thymeleaf, Hibernate, MySQL
- Spring Data JPA CRUD Example Tutorial // Popular

How to write this query by method @Query(value = "SELECT user FROM User server WHERE user.firstName LIKE :s% or user.lastName LIKE :s% ")
This one actually @Query(value = "SELECT user FROM User user WHERE user.firstName LIKE :s% or user.lastName LIKE :s% ") REPLY
u r the best...keepgoing...gbu....
Post a Comment
Leave Comment
My Top and Bestseller Udemy Courses
- Spring 6 and Spring Boot 3 for Beginners (Includes Projects)
- Building Real-Time REST APIs with Spring Boot
- Building Microservices with Spring Boot and Spring Cloud
- Full-Stack Java Development with Spring Boot 3 & React
- Testing Spring Boot Application with JUnit and Mockito
- Master Spring Data JPA with Hibernate
- Spring Boot Thymeleaf Real-Time Web Application - Blog App
Check out all my Udemy courses and updates: Udemy Courses - Ramesh Fadatare
Copyright © 2018 - 2025 Java Guides All rights reversed | Privacy Policy | Contact | About Me | YouTube | GitHub

26 Types of Punctuation Marks & Typographical Symbols
- What Is Punctuation?
- What Is A Typographical Symbol?
- Punctuation Vs. Typographical Symbols
- Types Of Punctuation And Symbols
- Try Grammar Coach
We use words in writing. Shocking, I know! Do you know what else we use in writing? Here is a hint: they have already appeared in this paragraph. In addition to words, we use many different symbols and characters to organize our thoughts and make text easier to read. All of these symbols come in two major categories: punctuation marks and typographical symbols . These symbols have many different uses and include everything from the humble period ( . ) to the rarely used caret symbol ( ^ ). There may even be a few symbols out there that you’ve never even heard of before that leave you scratching your head when you see them on your keyboard!
What is punctuation ?
Punctuation is the act or system of using specific marks or symbols in writing to separate different elements from each other or to make writing more clear. Punctuation is used in English and the other languages that use the Latin alphabet. Many other writing systems also use punctuation, too. Thanks to punctuation, we don’t have to suffer through a block of text that looks like this:
- My favorite color is red do you like red red is great my sister likes green she always says green is the color of champions regardless of which color is better we both agree that no one likes salmon which is a fish and not a color seriously
Punctuation examples
The following sentences give examples of the many different punctuation marks that we use:
- My dog , Bark Scruffalo , was featured in a superhero movie .
- If there ’ s something strange in your neighborhood , who are you going to call ?
- A wise man once said , “ Within the body of every person lies a skeleton .”
- Hooray ! I found everything on the map : the lake , the mountain , and the forest .
- I told Ashley ( if that was her real name ) that I needed the copy lickety-split .
What is a typographical symbol ?
The term typographical symbol , or any other number of phrases, refers to a character or symbol that isn’t considered to be a punctuation mark but may still be used in writing for various purposes. Typographical symbols are generally avoided in formal writing under most circumstances. However, you may see typographic symbols used quite a bit in informal writing.
Typographical symbol examples
The following examples show some ways that a writer might use typographical symbols. Keep in mind that some of these sentences may not be considered appropriate in formal writing.
- The frustrated actor said she was tired of her co-star’s “annoying bull **** .”
- For questions, email us at anascabana @ bananacabanas.fake!
- The band had five # 1 singles on the American music charts during the 1990s.
- My internet provider is AT & T.
⚡️ Punctuation vs. typographical symbols
Punctuation marks are considered part of grammar and often have well-established rules for how to use them properly. For example, the rules of proper grammar state that a letter after a period should be capitalized and that a comma must be used before a coordinating conjunction.
Typographical symbols, on the other hand, may not have widely accepted rules for how, or even when, they should be used. Generally speaking, most grammar resources will only allow the use of typographical symbols under very specific circumstances and will otherwise advise a writer to avoid using them.
Types of punctuation and symbols
There are many different types of punctuation marks and typographical symbols. We’ll briefly touch on them now, but you can learn more about these characters by checking out the links in this list and also each section below:
- Question mark
- Exclamation point
- Parentheses
- Square brackets
- Curly brackets
- Angle brackets
- Quotation marks
- Bullet point
- Pound symbol
- Caret symbol
- Pipe symbol
Period, question mark, and exclamation point
These three commonly used punctuation marks are used for the same reason: to end an independent thought.
A period is used to end a declarative sentence . A period indicates that a sentence is finished.
- Today is Friday .
Unique to them, periods are also often used in abbreviations.
- Prof . Dumbledore once again awarded a ludicrous amount of points to Gryffindor.
Question mark (?)
The question mark is used to end a question, also known as an interrogative sentence .
- Do you feel lucky ?
Exclamation point (!)
The exclamation point is used at the end of exclamations and interjections .
- Our house is haunted !
Comma, colon, and semicolon
Commas, colons, and semicolons can all be used to connect sentences together.
The comma is often the punctuation mark that gives writers the most problems. It has many different uses and often requires good knowledge of grammar to avoid making mistakes when using it. Some common uses of the comma include:
- Joining clauses: Mario loves Peach , and she loves him .
- Nonrestrictive elements: My favorite team , the Fighting Mongooses , won the championship this year.
- Lists: The flag was red , white , and blue.
- Coordinate adjectives: The cute , happy puppy licked my hand.
Try out this quiz on the Oxford comma!
The colon is typically used to introduce additional information.
- The detective had three suspects : the salesman, the gardener, and the lawyer.
Like commas, colons can also connect clauses together.
- We forgot to ask the most important question : who was buying lunch?
Colons have a few other uses, too.
- The meeting starts at 8:15 p.m.
- The priest started reading from Mark 3:6 .
Semicolon (;)
Like the comma and the colon, the semicolon is used to connect sentences together. The semicolon typically indicates that the second sentence is closely related to the one before it.
- I can’t eat peanuts ; I am highly allergic to them.
- Lucy loves to eat all kinds of sweets ; lollipops are her favorite.
Hyphen and dashes (en dash and em dash)
All three of these punctuation marks are often referred to as “dashes.” However, they are all used for entirely different reasons.
The hyphen is used to form compound words.
- I went to lunch with my father-in-law .
- She was playing with a jack-in-the-box .
- He was accused of having pro-British sympathies.
En dash (–)
The en dash is used to express ranges or is sometimes used in more complex compound words.
- The homework exercises are on pages 20–27 .
- The songwriter had worked on many Tony Award–winning productions.
Em dash (—)
The em dash is used to indicate a pause or interrupted speech.
- The thief was someone nobody expected —me !
- “Those kids will— ” was all he managed to say before he was hit by a water balloon.
Test your knowledge on the different dashes here.
Parentheses, brackets, and braces
These pairs of punctuation marks look similar, but they all have different uses. In general, the parentheses are much more commonly used than the others.
Parentheses ()
Typically, parentheses are used to add additional information.
- I thought (for a very long time) if I should actually give an honest answer.
- Tomorrow is Christmas (my favorite holiday) !
Parentheses have a variety of other uses, too.
- Pollution increased significantly. (See Chart 14B)
- He was at an Alcoholics Anonymous (AA) meeting.
- Richard I of England (1157–1199) had the heart of a lion.
Square brackets []
Typically, square brackets are used to clarify or add information to quotations.
- According to an eyewitness, the chimpanzees “climbed on the roof and juggled [bananas] .”
- The judge said that “the defense attorney [Mr. Wright] had made it clear that the case was far from closed.”
Curly brackets {}
Curly brackets , also known as braces , are rarely used punctuation marks that are used to group a set.
- I was impressed by the many different colors {red, green, yellow, blue, purple, black, white} they selected for the flag’s design.
Angle brackets <>
Angle brackets have no usage in formal writing and are rarely ever used even in informal writing. These characters have more uses in other fields, such as math or computing.
Quotation marks and apostrophe
You’ll find these punctuation marks hanging out at the top of a line of text.
Quotation marks (“”)
The most common use of quotation marks is to contain quotations.
- She said, “ Don’t let the dog out of the house. ”
- Bob Ross liked to put “ happy little trees ” in many of his paintings.
Apostrophe (‘)
The apostrophe is most often used to form possessives and contractions.
- The house ’ s back door is open.
- My cousin ’ s birthday is next week.
- It isn ’ t ready yet.
- We should ’ ve stayed outside.
Slash and ellipses
These are two punctuation marks you may not see too often, but they are still useful.
The slash has several different uses. Here are some examples:
- Relationships: The existence of boxer briefs somehow hasn’t ended the boxers/briefs debate.
- Alternatives: They accept cash and/or credit.
- Fractions: After an hour, 2/3 of the audience had already left.
Ellipses (…)
In formal writing, ellipses are used to indicate that words were removed from a quote.
- The mayor said, “The damages will be … paid for by the city … as soon as possible.”
In informal writing, ellipses are often used to indicate pauses or speech that trails off.
- He nervously stammered and said, “Look, I … You see … I wasn’t … Forget it, okay.”
Make Your Writing Shine!
- By clicking "Sign Up", you are accepting Dictionary.com Terms & Conditions and Privacy policies.
- Email This field is for validation purposes and should be left unchanged.
Typographical symbols
Typographical symbols rarely appear in formal writing. You are much more likely to see them used for a variety of reasons in informal writing.
Asterisk (*)
In formal writing, especially academic and scientific writing, the asterisk is used to indicate a footnote.
- Chocolate is the preferred flavor of ice cream.* * According to survey data from the Ice Cream Data Center.
The asterisk may also be used to direct a reader toward a clarification or may be used to censor inappropriate words or phrases.
Ampersand (&)
The ampersand substitutes for the word and . Besides its use in the official names of things, the ampersand is typically avoided in formal writing.
- The band gave a speech at the Rock & Roll Hall of Fame .
Bullet Point (•)
Bullet points are used to create lists. For example,
For this recipe you will need:
- baking powder
Pound symbol (#)
Informally, the pound symbol is typically used to mean number or is used in social media hashtags.
- The catchy pop song reached #1 on the charts.
- Ready 4 Halloween 2morrow!!! #spooky #TrickorTreat
Besides being used as an accent mark in Spanish and Portuguese words, the tilde is rarely used. Informally, a person may use it to mean “about” or “approximately.”
- We visited São Paulo during our vacation.
- I think my dog weighs ~20 pounds.
Backslash (\)
The backslash is primarily used in computer programming and coding. It might be used online and in texting to draw emoticons , but it has no other common uses in writing. Be careful not to mix it up with the similar forward slash (/), which is a punctuation mark.
At symbol (@)
The at symbol substitutes for the word at in informal writing. In formal writing, it is used when writing email addresses.
- His email address is [email protected] .
Caret symbol (^)
The caret symbol is used in proofreading, but may be used to indicate an exponent if a writer is unable to use superscript .
- Do you know what 3 ^ 4 (3 to the power of 4) is equal to?
Pipe symbol (|)
The pipe symbol is not used in writing. Instead, it has a variety of functions in the fields of math, physics, or computing.
How much do you know about verbs? Learn about them here.

Ways To Say
Synonym of the day
TechRepublic
Project management.
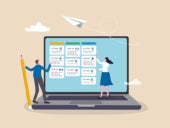
10 Best Free Project Management Software & Tools for 2024
Free project management software provides flexibility for managing projects without paying a cent. Our list includes free and freemium products that can manage most projects or introduce you to your next paid project management software.
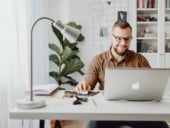
12 Best Project Management Software for Mac Users in 2024
This is a comprehensive list of the best project management software for Mac. Use this guide to compare and choose the best solutions for your business.
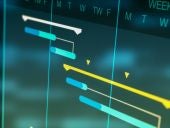
Asana vs Monday 2024: The Ultimate Project Management Showdown
Discover which project management tool leads in 2024. Compare Asana vs Monday on their features, usability, and more for the best fit, thanks to our expert research and guidance. Make your decision today.
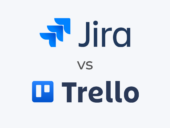
Trello vs. Jira Showdown: Who Wins in 2024?
Discover the key differences between Trello and Jira. Find out which project management tool tops your needs with our expertly researched guide.
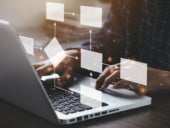
8 Best Flowchart Software Tools for 2024
This is a comprehensive list of the best flowchart software, covering features, pricing and more. Use this guide to determine the most suitable software for you.
Latest Articles
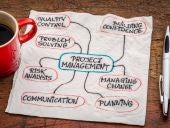
ClickUp vs Notion (2024): Which Tool Is Better For Your Team?
What is the difference between ClickUp and Notion? Read our article to compare their standout features, pros, cons, and more.
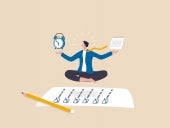
The 10 Best Project Management Software and Tools for 2024
With so many project management software options to choose from, it can seem daunting to find the right one for your projects or company. We’ve narrowed them down to these 10.
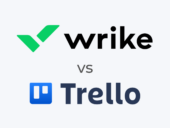
Wrike vs Trello (2024): Which Tool Is Best for Your Team?
This guide compares Trello and Wrike and helps you pick which one is the better project management tool for your business.
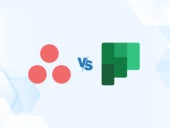
Microsoft Planner vs Asana: Which Tool is Better? (Updated for 2024)
Deciding between Asana and Planner? This comparison guide will help you evaluate their features, pricing, and more.
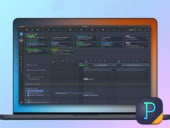
The Ultimate Task Management Tool is Just $25.50 for Life
Get organized and on-task with Pagico 10. Currently more than half off its regular price for a permanent lifetime license. Only through May 20, use code TRA15 at checkout for an extra 15% off its already discounted price.
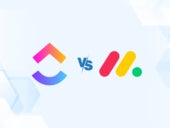
ClickUp vs monday.com (2024): Which is Best for Your Team?
Which is better for your team: ClickUp or monday.com? Use our guide to compare each tool's pricing, features, and more.
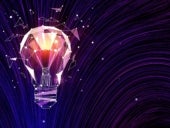
TechRepublic Premium Editorial Calendar: Policies, Checklists, Hiring Kits and Glossaries for Download
TechRepublic Premium content helps you solve your toughest IT issues and jump-start your career or next project.
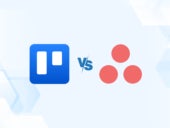
Trello vs Asana in 2024: The Best Tool Determined by Experts
Utilize our expert research on Trello vs. Asana to see which tool leads in 2024 for streamlining your projects and boosting team productivity.
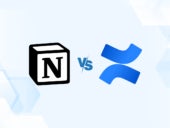
Notion vs Confluence (2024): Which Tool Should You Choose?
Which is better for your team, Notion or Confluence? Use our guide to compare each tool's pricing, features and more.
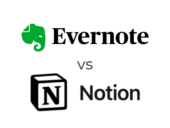
Notion vs. Evernote: Which Software Should You Use?
Notion vs Evernote. Compare features, pricing and more with our guide and get better informed decision about what would fit best for you.
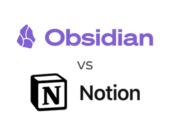
Obsidian vs. Notion (2024): Which Tool Is Better?
Which tool is better, Obsidian or Notion? Read our guide to learn more about pricing, features and more.
Create a TechRepublic Account
Get the web's best business technology news, tutorials, reviews, trends, and analysis—in your inbox. Let's start with the basics.
* - indicates required fields
Sign in to TechRepublic
Lost your password? Request a new password
Reset Password
Please enter your email adress. You will receive an email message with instructions on how to reset your password.
Check your email for a password reset link. If you didn't receive an email don't forgot to check your spam folder, otherwise contact support .
Welcome. Tell us a little bit about you.
This will help us provide you with customized content.
Want to receive more TechRepublic news?
You're all set.
Thanks for signing up! Keep an eye out for a confirmation email from our team. To ensure any newsletters you subscribed to hit your inbox, make sure to add [email protected] to your contacts list.
How to get the blue checkmark on X
What does the blue checkmark mean.
The blue checkmark means that the account has an active subscription to X Premium and meets our eligibility requirements.
Starting April 1, 2023 we began winding down our legacy Verification program and accounts that were verified under the previous criteria (active, notable, and authentic) will not retain a blue checkmark unless they are subscribed to X Premium.
Subscribers will need to meet our eligibility criteria below to receive or retain the blue checkmark.
Eligibility criteria for the blue checkmark
Only accounts actively subscribed to X Premium are eligible to receive the blue checkmark.
Our team uses an eligibility criteria on when the checkmark is given to ensure we maintain the integrity of the platform. Your account must meet the following criteria to receive or retain the blue checkmark:
Complete: Your account must have a display name and profile photo
Active use: Your account must be active in the past 30 days to subscribe to X Premium
Security: Your account must have a confirmed phone number
Non-Deceptive:
Your account must have no recent changes to your profile photo, display name, or username (@handle)
Your account must have no signs of being misleading or deceptive
Your account must have no signs of engaging in platform manipulation and spam
The checkmark will appear once our team reviews your Premium subscribed account and if it meets our requirements.
As a result of this change, X will no longer be accepting applications for the blue Verification checkmarks under the previous criteria (active, notable, and authentic) .
Loss of the blue checkmark
In accordance with the Terms of Service , including our Purchaser terms , X may remove the checkmark of an account at any time without notice.
To minimize confusion and promote integrity on the platform, changes to your profile photo, display name, or username (@handle) will result in a temporary loss of the blue checkmark until your account is validated as continuing to meet our requirements. No further changes to your profile photo, display name, or username will be allowed during this review period.
The checkmark will appear again once our team reviews your X Premium subscribed account and if it continues to meet our requirements.
X may also remove the checkmark from accounts that are found to be in violation of the X Rules.
You may not purchase X Premium if you are a person with whom X is not permitted to have dealings under US and any other applicable economic sanctions and trade compliance law.
Any violation of the X Terms of Service or X Rules, manipulation of X processes, or circumvention of enforcement actions may result in the loss of the checkmark or in certain instances, suspension.
Share this article
Did someone say … cookies? X and its partners use cookies to provide you with a better, safer and faster service and to support our business. Some cookies are necessary to use our services, improve our services, and make sure they work properly. Show more about your choices .
- Accept all cookies
- Refuse non-essential cookies
- Top Courses
- Online Degrees
- Find your New Career
- Join for Free
How to Write a Cover Letter When You’re Changing Careers (Sample + Tips)
As a career changer, you need to help recruiters understand why you’re moving away from your former line of work and what you want to achieve in your new career path..
![how to write name method [Featured Image] A man in a blue button-up is sitting down in a conference room holding pieces of paper.](https://d3njjcbhbojbot.cloudfront.net/api/utilities/v1/imageproxy/https://images.ctfassets.net/wp1lcwdav1p1/28u80RCd3SjJJ03qjwbPZJ/95337dc542ebaf56e3e04ba4835c2bab/9T9Z7AiJ.jpeg?w=1500&h=680&q=60&fit=fill&f=faces&fm=jpg&fl=progressive&auto=format%2Ccompress&dpr=1&w=1000)
You will inevitably change jobs throughout your career as you seek more responsibility, growth, or even a higher salary. According to the US Bureau of Labor Statistics, the average employee stays at each job for around four years [ 1 ]. However, for career changers—or those interested in exploring an entirely new path or industry—making that switch can sometimes involve unique challenges.
Even so, making a career change has become an increasingly popular move. More than half of workers in the United States anticipated looking for a new opportunity in 2022 [ 2 ]. Changing careers can allow you to find more meaningful work, better align your career path with your larger goals, and move into a more energizing role.
When you draft your cover letter to apply for a job in a new line of work, you must take time to explain your larger objectives. In this article, we’ll review specific information you can feature in your cover letter to help recruiters understand your goals and reasons for changing careers.
Learn more: How to Plan for a Career Change: Step-by-Step Guide
How to write a career change cover letter
A cover letter is a chance to expand upon the bullet points outlined in your resume . It’s a space where you can explain your interest in the role and company, highlight your experience and skills, and sell a recruiter on the overall fit you’d make.
But a career changer needs to do all of that and more. You also need to help recruiters and hiring managers understand why you’re moving away from your former line of work, what you want to achieve in your new career path, and any transferable skills that will help make your transition smooth.
Let’s review four key pieces of information you can weave into your career change cover letter.
1. Clarify your career change context
Explaining why you’re interested in changing careers and how the role you’re applying to fits within your larger career aspirations can preemptively contextualize your story. Plan to include a career change objective somewhere in your cover letter, much like you would a resume objective to provide a summary of a person’s experience and goals. Don’t be afraid to build a sense of personality so recruiters can better connect you with your objective.
What this looks like: I’ve spent the last six years translating complex topics for various users as a technical writer. But in that time, I’ve realized that what drives me is the user’s experience. It’s the lightbulb moment behind my career change to UX design . I believe I’ll make a strong addition to your team because my work has largely put the user front and center, and now I’m interested in focusing on a different facet of that goal.
2. Specify the value of your certificates, courses, or trainings
It costs over $4,000 to hire an employee, according to the Society for Human Resources Management [ 3 ]. That’s all the more reason why recruiters and hiring managers want to find the right candidate. It can be costly otherwise. Help explain what you’ve done to prepare for your career change by highlighting any professional certificates or trainings you’ve completed to prepare you for your new line of work.
What this looks like: In order to familiarize myself with the tools and processes used in data analysis, I completed the Google Data Analytics Professional Certificate , which taught me SQL and R, and trained me to clean and visualize data. Thanks to this preparation, I feel confident that I will make a strong addition to your team from the very start.
3. Bring attention to your transferable skills
Transferable skills are “portable,” in that you take them from job to job. They include problem-solving, critical thinking, attention to detail, and more. Show recruiters that you have important skills to help you do the job so they can understand the unique value you’d bring to their company.
It can also help to find out the key technical skills the job requires and spend time learning what you can, especially when it comes to important software or tools.
What this looks like: As a software developer, I regularly relied on my problem-solving skills to think through complex issues. I’ll bring that same skill, as well as my attention to detail, listening, and decision-making, to ABC High School as the new algebra teacher.
4. Highlight your past achievements
Any time you can highlight what you’ve managed to accomplish in your past roles, you help a recruiter see your potential in a new role. Where possible, summarize any moments that showcase your strengths and illustrate your work ethic or character.
What this looks like: I pride myself on being a team player and a problem-solver. As a social media manager at Company X, I identified a better program to help my team schedule content. Using that tool improved my team’s efficacy, leading to our most successful quarter.
Why is a cover letter important when changing careers?
The idea of a career path can sometimes be rigid, suggesting that people only follow one specific track. Although that perspective is starting to shift, it’s still prevalent. You can help recruiters and hiring managers understand more about your interest in a role by explaining why you’re changing careers and what you’ve done to streamline your transition.
It helps to align your cover letter with a resume objective, which can be especially useful for career changers. An objective on your resume is a place where you can contextualize your larger career aims, quickly summarizing what you’re hoping to achieve in your next role. Repeat that same information in your cover letter and expand on it slightly to give your application materials more cohesiveness.
Read more: How to Use Resume Sections to Shape Your Professional Story

Build job-ready skills with a Coursera Plus subscription
- Get access to 7,000+ learning programs from world-class universities and companies, including Google, Yale, Salesforce, and more
- Try different courses and find your best fit at no additional cost
- Earn certificates for learning programs you complete
- A subscription price of $59/month, cancel anytime
Career change cover letter sample
It's common practice nowadays to submit your cover letter digitally. In that case, include some of your contact information in the top left corner so recruiters can easily see how to get in touch.
Thomas Bennett
Nashville, TN
(555) 555-1234
Dear Ms. Tufte,
I’m writing to apply for the project manager role at Company X. I initially began my career as a marketing coordinator and eventually moved into email marketing , where I was responsible for strategizing and developing new campaigns. But in that time, I realized how much I thrived when managing our quarterly campaigns from start to finish. That’s why I’m interested in segueing into project management.
Knowing that, despite my experience, I still needed to learn more specifically about project management, I completed the Google Project Management Professional Certificate . Over six months, I’ve learned Agile project management as well as how to create product documentation, among other key skills. I believe this training, along with my previous experience, will help me transition to a project management role at Company X and make a big impact.
I’m an organized problem-solver with a sharp eye for detail, all important project management skills. I believe my previous work in email marketing provided hands-on training in managing projects, albeit without the official title. I identified new tools to help my team create more effective quarterly campaigns. As a result, we increased our click-through rate (one of our key metrics) to 1.87 percent, bringing it closer to the industry standard—an immense achievement.
I’m proud of the foundation I gained through marketing, but in realizing where my true passion lies, I’m keen to transition into a project management role with more growth opportunities. I appreciate your consideration.
Tips for strengthening your cover letter
Much like you would for a standard cover letter, you can strengthen your cover letter as a career changer using the following tips:
Tailor your letter for each role.
You should tailor your resume for each role you apply to, and the same goes for your cover letter. Research the company, find out about aspects of their work that interest you, and insert those details into your cover letter. You should also tailor your experience and skills, highlighting each job's most relevant skills and accomplishments.
Get specific.
Your cover letter should expand upon your resume rather than repeating the same information. One way to do this is by giving details about your past achievements. When possible, quantify your impact with numbers and explain how these accomplishments make you uniquely qualified for this new role.
Use action words.
Build action words into your resume and your cover letter. Rather than more staid words that don’t capture your unique story or responsibilities, action verbs can liven up your cover letter and make it more enticing to read. Find verbs that succinctly and accurately depict your previous experience.
Start advancing your skills today
Brush up on your cover letter writing skills by taking the University of Maryland’s free course, Writing Winning Resumes and Cover Letters . Or develop important skills for an in-demand career with a Professional Certificate from industry leaders like Google, Meta, and IBM. Most certificate programs take less than seven months to complete, and you can start for free with a seven-day, all-access trial.
Article sources
US Bureau of Labor Statistics. “ Employee Tenure in 2020 , https://www.bls.gov/news.release/pdf/tenure.pdf.” Accessed April 26, 2024.
CNBC. “ The Great Resignation is Likely to Continue , https://www.cnbc.com/2021/08/25/great-resignation-55-percent-are-looking-to-change-jobs-over-the-next-year-.html.” Accessed April 26, 2024.
ADP. “ Calculating the True Cost to Hire Employees , https://www.adp.com/spark/articles/2019/07/calculating-the-true-cost-to-hire-employees.aspx.” Accessed April 26, 2024.
Keep reading
Coursera staff.
Editorial Team
Coursera’s editorial team is comprised of highly experienced professional editors, writers, and fact...
This content has been made available for informational purposes only. Learners are advised to conduct additional research to ensure that courses and other credentials pursued meet their personal, professional, and financial goals.
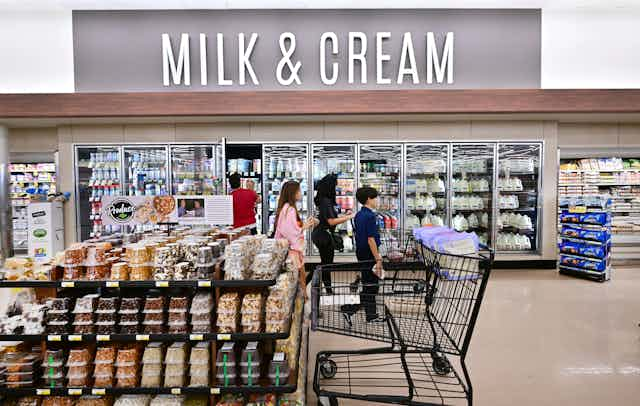
What is pasteurization? A dairy expert explains how it protects against foodborne illness, including avian flu
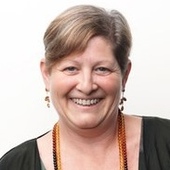
Associate Research Professor of Food Science, Penn State
Disclosure statement
Kerry Elizabeth Kaylegian receives funding from USDA.
Penn State provides funding as a founding partner of The Conversation US.
View all partners
Recent reports that the H5N1 avian flu virus has been found in cow’s milk have raised questions about whether the U.S. milk supply is safe to drink. According to the federal Food and Drug Administration, the answer is yes, as long as the milk is pasteurized .
Nonetheless, raw (unpasteurized) milk sales are up , despite health experts’ warning that raw milk could contain high levels of the virus , along with many other pathogens.
As an extension food scientist in a state where raw milk sales are legal , I provide technical support to help processors produce high-quality, safe dairy foods. I also like to help people understand the confusing world of pasteurization methods on their milk labels, and why experts strongly discourage consuming raw milk and products made from it.
What can make milk unsafe
Dairy products, like many foods, have inherent risks that can cause a variety of illnesses and even death. Our milk comes from animals that graze outdoors and live in barns. Milk is picked up from the farm in tanker trucks and delivered to the processing plant. These environments offer numerous opportunities for contamination by pathogens that cause illness and organisms that make food spoil.
For example, listeria monocytogenes comes from environmental sources like soil and water. Mild infections with listeriosis cause flu-like symptoms. More serious cases are, unfortunately, too common and can cause miscarriages in pregnant women and even death in extreme cases .
Other pathogens commonly associated with dairy animals and raw milk include E. coli , which can cause severe gastrointestinal infections and may lead to kidney damage; Campylobacter , the most common cause of diarrheal illness in the U.S.; and Salmonella , which cause abdominal pain, diarrhea and other symptoms.
Keeping beverages safe with heat
In the 1860s, French microbiologist Louis Pasteur discovered that heating wine and beer killed the organisms that caused spoilage, which then was a significant problem in France.
This heating process, which became known as pasteurization, was adopted in the U.S. prior to World War II, at a time when milk was responsible for 25% of all U.S. outbreaks of foodborne illnesses . In 1973, the federal government required that all milk sold across state lines in the U.S. had to be pasteurized, and in 1987, it banned interstate sales of raw milk .
Pasteurization heats every particle of a food to a specific temperature for a continuous length of time in order to kill the most heat-resistant pathogen associated with that product. Different organisms have different responses to heat, so controlled scientific studies are required to determine what length of time at a given temperature will kill a specific organism.
Since 1924, pasteurization in the U.S. has been guided by the Grade “A” Pasteurized Milk Ordinance , a federal guidance document that is updated every two years to reflect current science and has been adopted by all 50 states . Pasteurization equipment in the U.S. must meet stringent requirements that include sanitary design, safety controls and material standards.
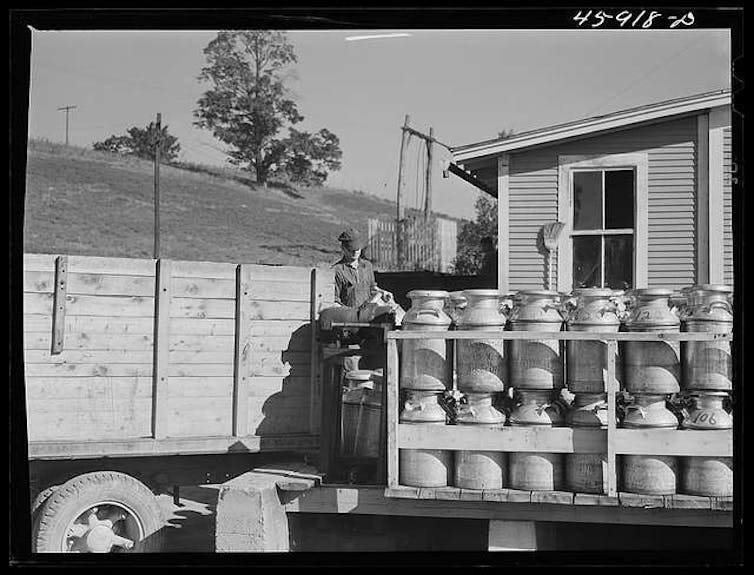
Pasteurization methods
Dairy processors can choose among several different types of pasteurization. When executed properly, all of these methods produce the same result: pathogen-free milk. Processors may treat milk beyond minimum times or temperatures to provide an extra margin of safety, or to reduce bacteria that can cause milk to spoil, thus increasing the product’s shelf life.
Vat pasteurizers , also known as batch pasteurizers, often are used by smaller-scale processors who handle limited volumes. The milk is pumped into a temperature-controlled tank with a stirrer, heated to a minimum of 145 degrees Fahrenheit (63 Celsius) and held there continuously for 30 minutes. Then it is cooled and pumped out of the vat.
The most common method used for commercial milk is high-temperature short-time pasteurization , which can treat large volumes of milk. The milk is pumped through a series of thin plates at high speed to reach a minimum temperature of 161 F (71 C). Then it travels through a holding tube for 15 seconds, and the temperature is checked automatically for safety and cooled.
The most complex and expensive systems are ultra-pasteurizers and ultra-high-temperature pasteurizers , which pasteurize milk in just a few seconds at temperatures above 285 F (140 C). This approach destroys many spoilage organisms, giving the milk a significantly longer shelf life than with other methods, although sometimes products made this way have more of a “cooked” flavor.
Ultra-high-temperature products are processed in a sterile environment and packaged in sterile packaging, such as lined cartons and pouches. They can be shelf-stable for up to a year before they are opened. Ultra-high-temperature packaging makes taking milk to school for lunch safe for kids every day.
Avian flu in milk
The detection of avian flu virus fragments in milk is a new challenge for the dairy industry. Scientists do not have a full picture of the risks to humans but are learning.
Research so far has shown that virus particles end up in the milk of infected cows, but that pasteurization will inactivate the virus . However, the FDA is advising consumers not to drink raw milk because there is limited information about whether it may transmit avian flu .
The agency also is urging producers not to manufacture or sell raw milk or raw milk products, including cheese, made with milk from cows showing symptoms of illness.
It’s never a good time to get a foodborne illness, and this is the beginning of ice cream season . At a time when avian flu is showing up in new species and scientists are still learning about how it is transmitted, I agree with the FDA that raw milk poses risks not worth taking.
- Avian flu H5N1
- Food safety
- Dairy products
- US agriculture
- Significant Terms
- Foodborne illness

Compliance Lead

Lecturer / Senior Lecturer - Marketing

Assistant Editor - 1 year cadetship

Executive Dean, Faculty of Health

Lecturer/Senior Lecturer, Earth System Science (School of Science)
Derived from the Greek words for “hidden writing,” cryptography is the science of obscuring transmitted information so that it may only be read by the intended recipient. The applications of cryptography are endless. From the quotidian end-to-end message authentication on WhatsApp to the practical digital signatures on legal forms or even the CPU-draining ciphers that are used for mining cryptocurrency , cryptography became an essential aspect of the digital world and a critical cybersecurity component for protecting sensitive data from hackers and other cybercriminals.
The practice of cryptology dates back to ancient times, with one of the earliest examples being attributed to Julius Caesar himself. Modern cryptosystems are far more advanced but still function in similar ways. Most cryptosystems begin with an unencrypted message known as plaintext, which is then encrypted into an indecipherable code known as ciphertext by using one or more encryption keys. This ciphertext is then transmitted to a recipient. If the ciphertext is intercepted and the encryption algorithm is strong, the ciphertext is useless to any unauthorized eavesdroppers because they will not be able to break the code. However, the intended recipient will easily be able to decipher the text, assuming that they have the correct decryption key.
Before diving deeper, let’s look at the core features of strong cryptographic frameworks:
- Confidentiality: Encrypted information can only be accessed by the person for whom it is intended and no one else.
- Integrity: Encrypted information cannot be modified in storage or in transit between the sender and the intended receiver without any alterations being detected.
- Nonrepudiation: The creator/sender of encrypted information cannot deny their intention to send the information.
- Authentication: The identities of the sender and receiver—as well as the origin and destination of the information—are confirmed.
- Key management: The keys that are used in encrypting and decrypting data (and associated tasks like key length, distribution, generation, rotation) are kept secure.
Although hybrid systems do exist (such as the SSL internet protocols), most encryption techniques fall into one of three main categories: symmetric cryptography algorithms , asymmetric cryptography algorithms or hash functions.
Also known as private key cryptography, secret key cryptography or single-key encryption, symmetric key encryption uses only one key for both the encryption process and decryption process. For these types of systems, each user must have access to the same private key. Private keys might be shared either through a previously established secure communication channel like a private courier or secured line or, more practically, a secure key exchange method like the Diffie-Hellman key agreement.
There are two types of symmetric key algorithms:
- Block cipher: In a block cipher, the cipher algorithm works on a fixed-size block of data. For example, if the block size is eight, eight bytes of plaintext are encrypted at a time. Normally, the user’s interface to the encrypt/decrypt operation handles data longer than the block size by repeatedly calling the low-level cipher function.
- Stream cipher: Stream ciphers do not work on a block basis, but rather convert one bit (or one byte) of data at a time. Basically, a stream cipher generates a keystream based on the provided key. The generated keystream is then XORed with the plaintext data.
Some examples of symmetrical cryptography include the following:
- Data Encryption Standard: The Data Encryption Standard ( DES ) was developed by IBM in the early 1970’s, and while it is now considered to be susceptible to brute force attacks, its architecture remains highly influential in the field of modern cryptography.
- Triple DES: While advancements in computing made DES insecure by 1999, the DES cryptosystem built on the original DES foundation adds extra levels of security that cannot be broken by modern machines.
- Blowfish: A fast, free, publicly available block cipher designed by Bruce Schneer in 1993.
- Advanced Encryption Standard: The Advanced Encryption Standard ( AES ) is the first and only publicly accessible cipher that is approved by the US National Security Agency for top secret information.
In asymmetric encryption, a pair of keys is used: one secret key and one public key. For this reason, these algorithms are also referred to as public key algorithms. Public key cryptography is considered to be more secure than symmetric encryption techniques because even though one key is publicly available, an encrypted message can only be decrypted with the intended recipient’s private key.
Some examples of asymmetrical cryptography include the following:
- RSA: Named for its founders—Rivest, Shamier and Adleman—in 1977, the RSA algorithm is one of the oldest widely used public key cryptosystems used for secure data transmission.
- ECC: Elliptic curve cryptography is an advanced form of asymmetric encryption that uses the algebraic structures of elliptic curves to create strong cryptographic keys.
A cryptographic hash algorithm produces a fixed-length output string (often called a digest) from a variable-length input string. The input serves as the plaintext, and the output hash is the cipher. For all practical purposes, the following statements are true of a good hash function:
- Collision resistant: If any portion of the data is modified, a different hash is generated, ensuring data integrity.
- One-way: The function is irreversible. That is, given a digest, it is not possible to find the data that produces it, ensuring data security .
For these reasons, hash algorithms make for effective cryptosystems because the hash algorithm encrypts the data directly without the need for different keys. In essence, the plaintext is its own key.
Consider the security vulnerability of a database of stored bank account passwords. Anyone with either authorized or unauthorized access to the bank’s computer systems might potentially read every password. To maintain data security, banks and other businesses encrypt sensitive information like passwords into a hash value and store only that encrypted value in their database. Without knowing the user’s password, the hash value cannot be broken.
Quantum cryptography
Keeping pace with advancing technology and increasingly sophisticated cyberattacks , the field of cryptography continues to evolve. Quantum cryptography , or quantum encryption, refers to the applied science of securely encrypting and transmitting data based on the naturally occurring and immutable laws of quantum mechanics for use in cybersecurity. While still in its early stages, quantum encryption has the potential to be far more secure than previous types of cryptographic algorithms and, theoretically, even unhackable.
Post-quantum cryptography
Not to be confused with quantum cryptography, which relies on the natural laws of physics to produce secure cryptosystems, post-quantum cryptographic algorithms use different types of mathematical cryptography to create quantum computer-proof encryption. Although not yet viable, quantum computing is a quickly developing field of computer science with the potential to exponentially increase processing power—dwarfing even the fastest super computers operating today. While still theoretical, prototypes have demonstrated that practical quantum computers might be expected to break even the most secure public key cryptography systems within the next 10 to 50 years. According to the National Institute of Standards and Technology (link resides outside ibm.com), the goal of post-quantum cryptography (also called quantum-resistant or quantum-safe cryptography) is to “develop cryptographic systems that are secure against both quantum and classical computers, and [that] can interoperate with existing communications protocols and networks.”
The six primary areas of quantum-safe cryptography are:
- Lattice-based cryptography
- Multivariate cryptography
- Hash-based cryptography
- Code-based cryptography
- Isogeny-based cryptography
- Symmetric key quantum resistance
IBM cryptography solutions combine technologies, consulting, systems integration and managed security services to help ensure crypto agility, quantum-safety and solid governance and risk policies. From symmetric to asymmetric cryptography to hash functions and beyond, ensure data and mainframe security with end-to-end encryption tailor made to meet your business needs.
Get digestible, high-impact insights from IBM security experts, offering smart strategies and invaluable expertise to combat modern cyber threats-straight to your inbox.
Explore IBM cryptography solutions
Get our newsletters and topic updates that deliver the latest thought leadership and insights on emerging trends.

COMMENTS
Example Explained. myMethod() is the name of the method static means that the method belongs to the Main class and not an object of the Main class. You will learn more about objects and how to access methods through objects later in this tutorial. void means that this method does not have a return value. You will learn more about return values later in this chapter
Defining Methods. Here is an example of a typical method declaration: double length, double grossTons) {. //do the calculation here. The only required elements of a method declaration are the method's return type, name, a pair of parentheses, (), and a body between braces, {}. More generally, method declarations have six components, in order:
The getName () method of java.lang.reflect .Method class is helpful to get the name of methods, as a String. To get name of all methods of a class, get all the methods of that class object. Then call getName () on those method objects. Syntax: Return Value: It returns the name of the method, as String. Example:
Programming principles tell us that naming in code is important. This article aims to be a complete learner's guide for naming in code. It also aims to be a reference about naming that you can refer to in the future if you need to. For certain things, such as naming methods, there are different naming conventions.
3. Method Name: the rules for field names apply to method names as well, but the convention is a little different. It is Mandatory in syntax.. 4. Parameter list: Comma-separated list of the input parameters is defined, preceded by their data type, within the enclosed parenthesis. If there are no parameters, you must use empty parentheses (). It is Optional in syntax.
Kent Beck suggests: One test fixture per 'unit' (class of your program). Test fixtures are classes themselves. The test fixture name should be: [name of your 'unit']Tests. Test cases (the test fixture methods) have names like: test[feature being tested] For example, having the following class: class Person {.
etc. These names are meaningless, they don't bring us any value. Look at this code: actvUsr(String text) {. User usr = rpstry.find(text); usr.actv(); } We have a method called actvUsr that ...
To call a method in Java, write the method name followed by a set of parentheses (), followed by a semicolon (;). A class must have a matching filename (Main and Main.java). Using Multiple Classes. Like we specified in the Classes chapter, it is a good practice to create an object of a class and access it in another class.
Declaring a Java Method. The syntax to declare a method is: returnType methodName() { // method body } Here, returnType - It specifies what type of value a method returns For example if a method has an int return type then it returns an integer value. If the method does not return a value, its return type is void.; methodName - It is an identifier that is used to refer to the particular method ...
It's okay if method names are long - Martin states that "A long descriptive name is better than a short enigmatic name." It's also good convention to use similar verbage for actions taken or abstract concepts such as get whenever retrieving a value, set whenever setting a value, or write whenever writing something to a file.
Below are some naming conventions of the java programming language. They must be followed while developing software in java for good maintenance and readability of code. Java uses CamelCase as a practice for writing names of methods, variables, classes, packages, and constants. Camel's case in java programming consists of compound words or ...
While defining a method, remember that the method name must be a verb and start with a lowercase letter. If the method name has more than two words, the first name must be a verb followed by adjective or noun. In the multi-word method name, the first letter of each word must be in uppercase except the first word. For example: Single-word method ...
Don't reuse same variable name in the same class in different contexts: e.g. in method, constructor, class. So you can provide more simplicity for understandability and maintainability.
After all, the reason we write functions is to decompose a larger concept (in other words, the name of the function) into a set of steps at the next level of abstraction. The stepdown rule. This ...
Advertisements. How do I write method names in Java - While writing a method name we should follow the camel case i.e. first letter of the first word should be small and the first letters of the remaining (later) words should be capital. Example public class Test { public void sampleMethod () { System.out.println (This is sample method);
Spring Data JPA supports a variable called entityName. Its usage is select x from # {#entityName} x. It inserts the entityName of the domain type associated with the given repository. The entityName is resolved as follows: If the domain type has set the name property on the @Entity annotation, it is used.
Java Naming Convention. Java naming convention is a rule to follow as you decide what to name your identifiers such as class, package, variable, constant, method, etc. But, it is not forced to follow. So, it is known as convention not rule. These conventions are suggested by several Java communities such as Sun Microsystems and Netscape.
1. Creating Spring Boot Project. Spring Boot provides a web tool called https://start.spring.io to bootstrap an application quickly. Just go to https://start.spring.io and generate a new spring boot project. Use the below details in the Spring boot creation: Project Name: spring-data-jpa-course. Project Type: Maven.
2. Structure of Derived Query Methods in Spring. Derived method names have two main parts separated by the first By keyword: List<User> findByName(String name) The first part — such as find — is the introducer, and the rest — such as ByName — is the criteria. Spring Data JPA supports find, read, query, count and get.
No sentence is complete without a punctuation mark! Learn about the common types of punctuation marks & typographical symbols and how to use them.
Create a new file object using the open() function, specifying the file name with the mode set as 'w' for writing. ... Use the writerows() method to write the data to the CSV file writer.writerows(data) This will create a new file named 'Students_Data.csv' with Name, M1 Score, and M2 Score as the header/column names and the data values ...
4. Since camel cases capitalizes the first letter of each word to substitute spaces, we are left with the challenge of how to differentiate a capitalized title, like we would in English for a proper noun. As a solution to this, the first word in a camel case identifier is capitalized to indicate the title or identifier is capitalized.
Free project management software provides flexibility for managing projects without paying a cent. Our list includes free and freemium products that can manage most projects or introduce you to ...
Complete: Your account must have a display name and profile photo. Active use: Your account must be active in the past 30 days to subscribe to X Premium. Security: Your account must have a confirmed phone number. Non-Deceptive: Your account must have no recent changes to your profile photo, display name, or username (@handle)
How to write a career change cover letter. A cover letter is a chance to expand upon the bullet points outlined in your resume. It's a space where you can explain your interest in the role and company, highlight your experience and skills, and sell a recruiter on the overall fit you'd make. But a career changer needs to do all of that and more.
The CanConstruct method checks a data source reference for valid syntax. The CanConstruct method must have the default data system defined as part of the object name. For example, the object name AI would not work correctly. Object.CanConstruct(ObjectName As String, (Returned)_ CanConstruct As Boolean) Example: Script Using CanConstruct Method
Kerry Elizabeth Kaylegian receives funding from USDA. Recent reports that the H5N1 avian flu virus has been found in cow's milk have raised questions about whether the U.S. milk supply is safe ...
It's very easy to write such code to be secure and I have done so in multiple languages. For example, one could make a set of allowable methods, and only allow a method to be invoked if it's name is in the set. Even more secure (yet still bone-head simple) would be limiting each allowed method to a specific state, and not allowing the method to ...
How to Write a Marketing Proposal. Follow these steps to write a persuasive marketing proposal that impresses your prospective clients. 1. Design a Polished and Visually Appealing Cover Page. Your cover page is the window to the rest of your proposal. So you should aim to make an excellent first impression.
Derived from the Greek words for "hidden writing," cryptography is the science of obscuring transmitted information so that it may only be read by the intended recipient. The applications of cryptography are endless. From the quotidian end-to-end message authentication on WhatsApp to the practical digital signatures on legal forms or even the CPU-draining ciphers that are used for mining ...