This browser is no longer supported.
Upgrade to Microsoft Edge to take advantage of the latest features, security updates, and technical support.

Extension Methods (C# Programming Guide)
- 26 contributors
Extension methods enable you to "add" methods to existing types without creating a new derived type, recompiling, or otherwise modifying the original type. Extension methods are static methods, but they're called as if they were instance methods on the extended type. For client code written in C#, F# and Visual Basic, there's no apparent difference between calling an extension method and the methods defined in a type.
The most common extension methods are the LINQ standard query operators that add query functionality to the existing System.Collections.IEnumerable and System.Collections.Generic.IEnumerable<T> types. To use the standard query operators, first bring them into scope with a using System.Linq directive. Then any type that implements IEnumerable<T> appears to have instance methods such as GroupBy , OrderBy , Average , and so on. You can see these additional methods in IntelliSense statement completion when you type "dot" after an instance of an IEnumerable<T> type such as List<T> or Array .
OrderBy Example
The following example shows how to call the standard query operator OrderBy method on an array of integers. The expression in parentheses is a lambda expression. Many standard query operators take lambda expressions as parameters, but this isn't a requirement for extension methods. For more information, see Lambda Expressions .
Extension methods are defined as static methods but are called by using instance method syntax. Their first parameter specifies which type the method operates on. The parameter follows the this modifier. Extension methods are only in scope when you explicitly import the namespace into your source code with a using directive.
The following example shows an extension method defined for the System.String class. It's defined inside a non-nested, non-generic static class:
The WordCount extension method can be brought into scope with this using directive:
And it can be called from an application by using this syntax:
You invoke the extension method in your code with instance method syntax. The intermediate language (IL) generated by the compiler translates your code into a call on the static method. The principle of encapsulation isn't really being violated. Extension methods can't access private variables in the type they're extending.
Both the MyExtensions class and the WordCount method are static , and it can be accessed like all other static members. The WordCount method can be invoked like other static methods as follows:
The preceding C# code:
- Declares and assigns a new string named s with a value of "Hello Extension Methods" .
- Calls MyExtensions.WordCount given argument s .
For more information, see How to implement and call a custom extension method .
In general, you'll probably be calling extension methods far more often than implementing your own. Because extension methods are called by using instance method syntax, no special knowledge is required to use them from client code. To enable extension methods for a particular type, just add a using directive for the namespace in which the methods are defined. For example, to use the standard query operators, add this using directive to your code:
(You may also have to add a reference to System.Core.dll.) You'll notice that the standard query operators now appear in IntelliSense as additional methods available for most IEnumerable<T> types.
Binding Extension Methods at Compile Time
You can use extension methods to extend a class or interface, but not to override them. An extension method with the same name and signature as an interface or class method will never be called. At compile time, extension methods always have lower priority than instance methods defined in the type itself. In other words, if a type has a method named Process(int i) , and you have an extension method with the same signature, the compiler will always bind to the instance method. When the compiler encounters a method invocation, it first looks for a match in the type's instance methods. If no match is found, it searches for any extension methods that are defined for the type, and bind to the first extension method that it finds.
The following example demonstrates the rules that the C# compiler follows in determining whether to bind a method call to an instance method on the type, or to an extension method. The static class Extensions contains extension methods defined for any type that implements IMyInterface . Classes A , B , and C all implement the interface.
The MethodB extension method is never called because its name and signature exactly match methods already implemented by the classes.
When the compiler can't find an instance method with a matching signature, it will bind to a matching extension method if one exists.
Common Usage Patterns
Collection functionality.
In the past, it was common to create "Collection Classes" that implemented the System.Collections.Generic.IEnumerable<T> interface for a given type and contained functionality that acted on collections of that type. While there's nothing wrong with creating this type of collection object, the same functionality can be achieved by using an extension on the System.Collections.Generic.IEnumerable<T> . Extensions have the advantage of allowing the functionality to be called from any collection such as an System.Array or System.Collections.Generic.List<T> that implements System.Collections.Generic.IEnumerable<T> on that type. An example of this using an Array of Int32 can be found earlier in this article .
Layer-Specific Functionality
When using an Onion Architecture or other layered application design, it's common to have a set of Domain Entities or Data Transfer Objects that can be used to communicate across application boundaries. These objects generally contain no functionality, or only minimal functionality that applies to all layers of the application. Extension methods can be used to add functionality that is specific to each application layer without loading the object down with methods not needed or wanted in other layers.
Extending Predefined Types
Rather than creating new objects when reusable functionality needs to be created, we can often extend an existing type, such as a .NET or CLR type. As an example, if we don't use extension methods, we might create an Engine or Query class to do the work of executing a query on a SQL Server that may be called from multiple places in our code. However we can instead extend the System.Data.SqlClient.SqlConnection class using extension methods to perform that query from anywhere we have a connection to a SQL Server. Other examples might be to add common functionality to the System.String class, extend the data processing capabilities of the System.IO.Stream object, and System.Exception objects for specific error handling functionality. These types of use-cases are limited only by your imagination and good sense.
Extending predefined types can be difficult with struct types because they're passed by value to methods. That means any changes to the struct are made to a copy of the struct. Those changes aren't visible once the extension method exits. You can add the ref modifier to the first argument making it a ref extension method. The ref keyword can appear before or after the this keyword without any semantic differences. Adding the ref modifier indicates that the first argument is passed by reference. This enables you to write extension methods that change the state of the struct being extended (note that private members aren't accessible). Only value types or generic types constrained to struct (see struct constraint for more information) are allowed as the first parameter of a ref extension method. The following example shows how to use a ref extension method to directly modify a built-in type without the need to reassign the result or pass it through a function with the ref keyword:
This next example demonstrates ref extension methods for user-defined struct types:
General Guidelines
While it's still considered preferable to add functionality by modifying an object's code or deriving a new type whenever it's reasonable and possible to do so, extension methods have become a crucial option for creating reusable functionality throughout the .NET ecosystem. For those occasions when the original source isn't under your control, when a derived object is inappropriate or impossible, or when the functionality shouldn't be exposed beyond its applicable scope, Extension methods are an excellent choice.
For more information on derived types, see Inheritance .
When using an extension method to extend a type whose source code you aren't in control of, you run the risk that a change in the implementation of the type will cause your extension method to break.
If you do implement extension methods for a given type, remember the following points:
- An extension method is not called if it has the same signature as a method defined in the type.
- Extension methods are brought into scope at the namespace level. For example, if you have multiple static classes that contain extension methods in a single namespace named Extensions , they'll all be brought into scope by the using Extensions; directive.
For a class library that you implemented, you shouldn't use extension methods to avoid incrementing the version number of an assembly. If you want to add significant functionality to a library for which you own the source code, follow the .NET guidelines for assembly versioning. For more information, see Assembly Versioning .
- Parallel Programming Samples (these include many example extension methods)
- Lambda Expressions
- Standard Query Operators Overview
- Conversion rules for Instance parameters and their impact
- Extension methods Interoperability between languages
- Extension methods and Curried Delegates
- Extension method Binding and Error reporting
Coming soon: Throughout 2024 we will be phasing out GitHub Issues as the feedback mechanism for content and replacing it with a new feedback system. For more information see: https://aka.ms/ContentUserFeedback .
Submit and view feedback for
Additional resources

C# - Extension Method
Extension methods, as the name suggests, are additional methods. Extension methods allow you to inject additional methods without modifying, deriving or recompiling the original class, struct or interface. Extension methods can be added to your own custom class, .NET framework classes, or third party classes or interfaces.
In the following example, IsGreaterThan() is an extension method for int type, which returns true if the value of the int variable is greater than the supplied integer parameter.
The IsGreaterThan() method is not a method of int data type (Int32 struct). It is an extension method written by the programmer for the int data type. The IsGreaterThan() extension method will be available throughout the application by including the namespace in which it has been defined.
The extension methods have a special symbol in intellisense of the visual studio, so that you can easily differentiate between class methods and extension methods.

Now let's see how to write an extension method.

An extension method is actually a special kind of static method defined in a static class. To define an extension method, first of all, define a static class.
For example, we have created an IntExtensions class under the ExtensionMethods namespace in the following example. The IntExtensions class will contain all the extension methods applicable to int data type. (You may use any name for namespace and class.)
Now, define a static method as an extension method where the first parameter of the extension method specifies the type on which the extension method is applicable. We are going to use this extension method on int type. So the first parameter must be int preceded with the this modifier.
For example, the IsGreaterThan() method operates on int, so the first parameter would be, this int i .
Now, you can include the ExtensionMethods namespace wherever you want to use this extension method.

- Extension methods are additional custom methods which were originally not included with the class.
- Extension methods can be added to custom, .NET Framework or third party classes, structs or interfaces.
- The first parameter of the extension method must be of the type for which the extension method is applicable, preceded by the this keyword.
- Extension methods can be used anywhere in the application by including the namespace of the extension method.
- Difference between Array and ArrayList
- Difference between Hashtable and Dictionary
- How to write file using StreamWriter in C#?
- How to sort the generic SortedList in the descending order?
- Difference between delegates and events in C#
- How to read file using StreamReader in C#?
- How to calculate the code execution time in C#?
- Design Principle vs Design Pattern
- How to convert string to int in C#?
- Boxing and Unboxing in C#
- More C# articles
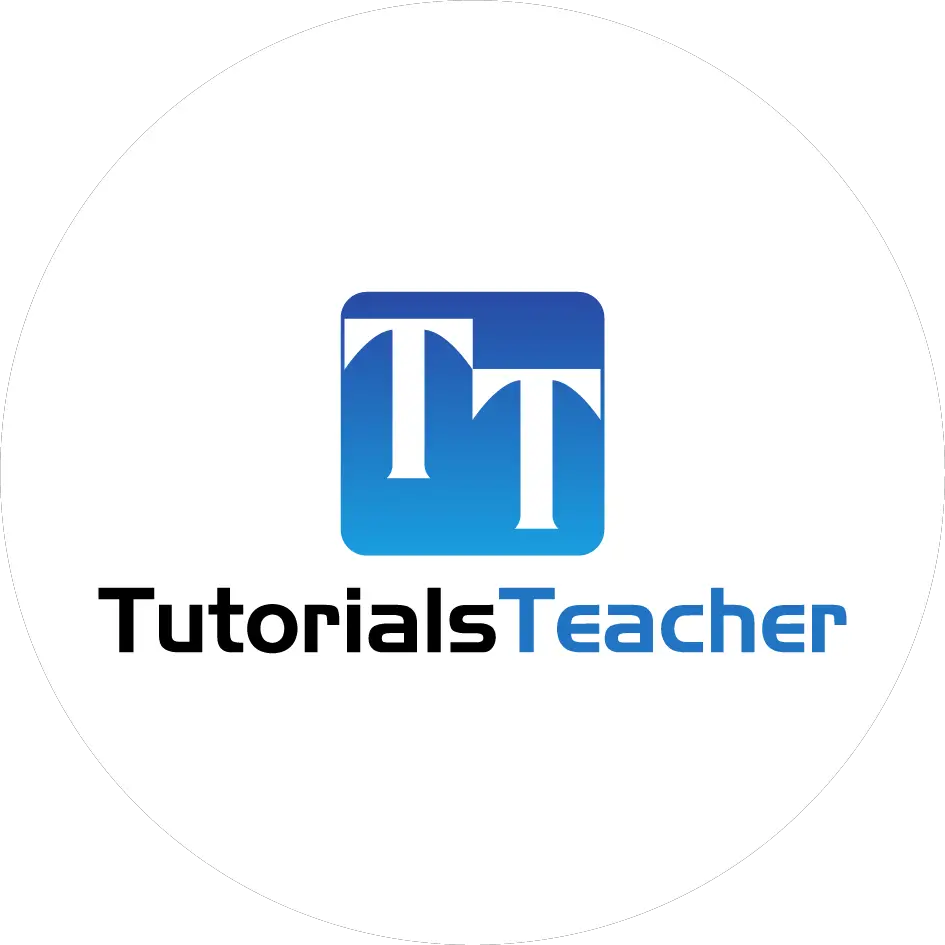
We are a team of passionate developers, educators, and technology enthusiasts who, with their combined expertise and experience, create in -depth, comprehensive, and easy to understand tutorials.We focus on a blend of theoretical explanations and practical examples to encourages hands - on learning. Visit About Us page for more information.
- C# Questions & Answers
- C# Skill Test
- C# Latest Articles
- .NET Framework
- C# Data Types
- C# Keywords
- C# Decision Making
- C# Delegates
- C# Constructors
- C# ArrayList
- C# Indexers
- C# Interface
- C# Multithreading
- C# Exception
Extension Method in C#
- Main Method in C#
- Method Hiding in C#
- DateTime.Add() Method in C#
- Decimal.Floor() Method in C#
- Stack.GetEnumerator Method in C#
- Decimal.Add() Method in C#
- DateTime.CompareTo() Method in C#
- C# | Clone() Method
- Double.GetTypeCode() Method in C#
- Anonymous Method in C#
- Decimal.Ceiling() Method in C#
- Java Extension Methods
- ASP GetExtensionName Method
- Dart Extension Methods in Flutter
- Extensions in Objective-C
- Can We Extend final Method in Java?
- C# File Format | .cs Extension
- jQuery.fn.extend() Method
- jQuery | extend() method
In C#, the extension method concept allows you to add new methods in the existing class or in the structure without modifying the source code of the original type and you do not require any kind of special permission from the original type and there is no need to re-compile the original type. It is introduced in C# 3.0 .
Let us discuss this concept with the help of an example. Suppose you have a class or a structure that contains three methods and you want to add two new methods in this class or structure, you do not have the source code of the class/structure or do not have permissions from the class/structure, or the class is a sealed class, but you still want to add new methods in it, then you can use the concept extension method to add the new method in the existing class/structure.
Now you create a new class that is static and contains the two methods that you want to add to the existing class, now bind this class with the existing class. After binding you will see the existing class can access the two newly added methods. As shown in the below program.
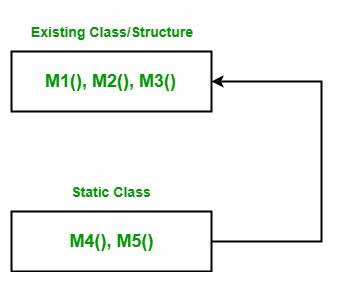
First we create a class named as Geek in Program1.cs file. It contains three methods that is M1() , M2() , and M3() .
Now we create a static class named as NewMethodClass in Program2.cs file. It contains two methods that are M4() and M5() . Now we want to add these methods in Geek class, so we use the binding parameter to bind these methods with Geek class. After that, we create another named as GFG in which Geek class access all the five methods.
Important Points:
- Here, Binding parameters are those parameters which are used to bind the new method with the existing class or structure. It does not take any value when you are calling the extension method because they are used only for binding not for any other use. In the parameter list of the extension method binding parameter is always present at the first place if you write binding parameter to second, or third, or any other place rather than first place then the compiler will give an error. The binding parameter is created using this keyword followed by the name of the class in which you want to add a new method and the parameter name. For example: this Geek g Here, this keyword is used for binding, Geek is the class name in which you want to bind, and g is the parameter name.
- Extension methods are always defined as a static method, but when they are bound with any class or structure they will convert into non-static methods.
- When an extension method is defined with the same name and the signature of the existing method, then the compiler will print the existing method, not the extension method. Or in other words, the extension method does not support method overriding .
- You can also add new methods in the sealed class also using an extension method concept.
- It cannot apply to fields, properties , or events.
- It must be defined in top-level static class.
- Multiple binding parameters are not allowed means an extension method only contains a single binding parameter. But you can define one or more normal parameter in the extension method.
Advantages:
- The main advantage of the extension method is to add new methods in the existing class without using inheritance .
- You can add new methods in the existing class without modifying the source code of the existing class.
- It can also work with sealed class .
Please Login to comment...
Similar reads.
- CSharp-method
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
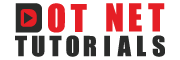
LINQ Extension Methods in C#
Back to: LINQ Tutorial For Beginners and Professionals
LINQ Extension Methods in C# with Examples
In this article, I will discuss the LINQ Extension Methods in C# with Examples. Please read our previous article before proceeding to this article, where we discussed the Differences between IEnumerable and IQueryable in C# . At the end of this article, you will understand the following three concepts in C#.
What are Extension Methods in C#?
- When should you use Extension Methods in C#?
How do you implement extension methods in C#?
- Understanding the LINQ Extension Methods.
What are LINQ Extension Methods?
The LINQ’s standard query operators, such as select, where, etc., are implemented in the Enumerable class. These methods are implemented as extension methods of the type IEnumerable<T> interface. Let us understand this with an example. We have the following code in our Main method.

The above Where() method does not belong to the List<T> class, but still, we can call it as it belongs to the List<T> class. Why can it be called using the List<T> object? Let’s find out. If you go to the definition of the Where method, then you will find the following definition.

As you can see in the signature, the where Where() method is implemented as an extension method on the IEnumerable<T> interface, and we know List<T> implements the IEnumerable<T> interface. This is the reason why we can call the Where() method using the List<T> object. With this in mind, let us understand what extension methods are and how they are implemented in C#.
According to MSDN, Extension Methods allow us to add methods to existing types without creating a new derived type, recompiling, or modifying the original type.
In simple words, we can say that the Extension methods can be used as an approach to extending the functionality of a class by adding new methods into the existing class if the source code of the class is not available or if we don’t have any permission in making changes to the existing class.
The most important point you need to remember is that extension methods are the static methods of a static class, but they will be called as if they were instance methods on the extended type.
When should you use extension methods in C#?
You need to use an extension method if any of the following conditions are true:
- You need a method on an existing type, and you are not the owner of the source code of that type.
- You need a method on an existing type; you do not own the source code of that type, but that type is an interface.
- You need a method on an existing type, you do not own the source code, and that type is not an interface, but adding the method creates undesired coupling.
Otherwise, you should go with the normal method of the actual type itself.
Let us understand this with an example. Our requirement is that we want to add a method in the built-in string class. Let’s call this method GetWordCount(), which will count the word present in a string separated by a space.
For example, if the string is “Welcome to Dotnet Tutorials,” it should return the word count as 4. The most important point is that we need to call this method on the String object, as shown below. int wordCount = sentence.GetWordCount();
Note: We cannot define the GetWordCount() method directly in the string class as we do not own the string class. The string class belongs to the System namespace, owned by the .NET framework. So, the alternative solution to achieve this is to write a wrapper class, as shown below.
The above ExtensionHelper Wrapper class works fine, but the problem is that we cannot call the GetWordCount() method using the string object, as shown below. int wordCount = sentence.GetWordCount();
Instead, we need to call the GetWordCount() method, as shown below. int wordCount = ExtensionHelper.GetWordCount(sentence);
How do you convert the above GetWordCount() method to an Extension Method of the String class?
Now, let’s convert the GetWordCount() method to an extension method on the String class. So that we can able to call the GetWordCount() method using the following syntax. int wordCount = sentence.GetWordCount();
To make the above GetWordCount() method an extension method, we need to make the following changes.
- First, we need to make the ExtensionHelper class a static class.
- Second, the type the method extends (i.e., string) should be passed as the first parameter preceding the “this” keyword to the GetWordCount() method.
With the above two changes in place, the GetWordCount() method becomes an extension method, and hence, we can call the GetWordCount() method in the same way as we call an instance method of a class. The complete example code is given below.
Now run the application, and you will see the word count as expected in the console window. Here, we can still call the GetWordCount() extension method using the wrapper class style syntax and get the output as expected, as shown below.
So, the point that I need to keep focus on is that this is how the extension methods are called internally behind the scenes.
That means it is also possible to call the LINQ extension methods such as select, where, etc., using the wrapper class style syntax. As all the LINQ extension methods are implemented in the Enumerable class, the syntax to call those methods should look as shown below.
LINQ Extension Method in C#:
LINQ (Language Integrated Query) extension methods in C# are a set of methods provided by the System.Linq namespace that extends the capabilities of collections (like arrays, lists, and other types implementing IEnumerable<T>) by adding query functionality. These methods enable querying collections using a SQL-like syntax directly in C#. Here’s an overview of some commonly used LINQ extension methods:
Where: Filters a sequence based on a predicate. var filteredResult = collection.Where(item => item.Property == someValue);
Select: Projects each element of a sequence into a new form. var projectedResult = collection.Select(item => new { item.Property1, item.Property2 });
OrderBy / OrderByDescending: Sorts the elements of a sequence in ascending or descending order. var sortedResult = collection.OrderBy(item => item.Property); var sortedDescendingResult = collection.OrderByDescending(item => item.Property);
GroupBy: Groups the elements of a sequence. var groupedResult = collection.GroupBy(item => item.Property);
FirstOrDefault / LastOrDefault: Returns the first or last element of a sequence or a default value if no element is found. var firstItem = collection.FirstOrDefault(); var lastItem = collection.LastOrDefault();
Sum / Min / Max / Average: Computes the sum, minimum, maximum, or average of a sequence. var total = collection.Sum(item => item.NumericProperty);
Any / All: Checks if any or all sequence elements satisfy a condition. bool hasItems = collection.Any(); bool allMatchCondition = collection.All(item => item.Property > someValue);
Distinct: Returns distinct elements from a sequence. var distinctItems = collection.Distinct();
Count: Counts the elements in a sequence, optionally matching a specific condition. int count = collection.Count(); int conditionalCount = collection.Count(item => item.Property == someValue);
Intersect / Except / Union: Performs set operations like intersection, difference, and union on sequences. var commonElements = collection1.Intersect(collection2);
In the next article, I will discuss the LINQ Operators in C# with Examples. In this article, I try to explain the Linq Extension Methods in C# with Examples. I hope you enjoy this LINQ Extension Methods in C# article.
About the Author: Pranaya Rout
Pranaya Rout has published more than 3,000 articles in his 11-year career. Pranaya Rout has very good experience with Microsoft Technologies, Including C#, VB, ASP.NET MVC, ASP.NET Web API, EF, EF Core, ADO.NET, LINQ, SQL Server, MYSQL, Oracle, ASP.NET Core, Cloud Computing, Microservices, Design Patterns and still learning new technologies.
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Home » C# Tutorial » C# Extension Methods
C# Extension Methods
Summary : in this tutorial, you’ll learn about C# extension methods and how to use them to extend any type with additional methods.
Introduction to C# extension methods
To add a method to a class , we often subclass the existing class and add the method to the derived class.
C# introduced a new feature called extension methods that allows you to add new methods to an existing type (or class) without the need of using inheritance .
An extension method is a static method but it is called as if it was an instance method on the extended type.
Creating C# extension methods
To create an extension method, you define a static class that contains the extension method. For example, the following creates an extension method on the string type:
How it works.
- First, define the static class called StringExtensions .
- Second, define the extension method ToTitleCase() as a static method of the StringExtensions class. The extension method uses this keyword before the first parameter. In this example, the ToTitleCase() method uses the current culture’s text info to convert a string to a title case.
Using C# extension methods
The following program demonstrates how to call the ToTitleCase() extension method on a string:
A more practical example of the C# extension method
The following example demonstrates how to use extension methods to extend the int type by adding two methods IsEven() and IsOdd() :
In this example, the IsEven() method returns true if the number is even and false otherwise. The IsOdd() method is the reverse of the IsEven() method.
In the Main() method of the Program class, we define a list of integers, iterate over its elements using a foreach , and write the even number to the console. We use the IsEven() extension method to check if a number is even or not.
Advantages of C# extension methods
Extension methods have the following advantages:
- Allow you to add functionality to the types that you don’t control such as .NET classes without the need of subclassing them.
- Make your code cleaner and more readable by keeping related methods in one place.
- Better discoverability: When working with an object, you can find all its extension methods via IntelliSense.
The following picture shows the available extension methods ( IsEven & IsOdd ) of the integer type that we defined in the previous example:
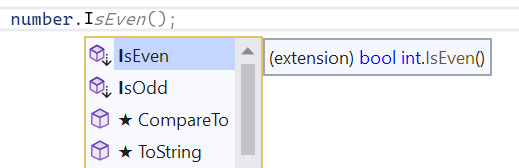
- C# extension methods allow you to extend an existing type without using inheritance.
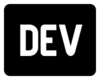
DEV Community

Posted on Apr 26, 2023
C# Extension Methods: the basics you need to know
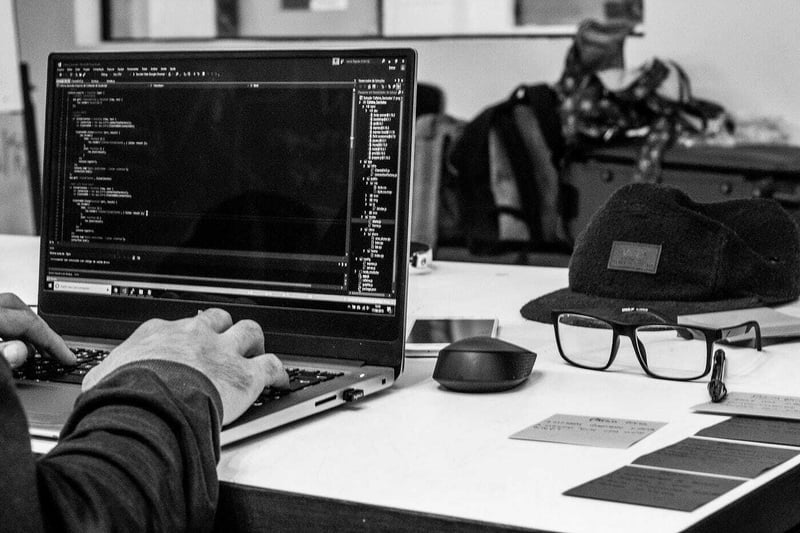
Extension methods are a powerful feature (introduced in C# 3.0) in object-oriented programming languages that allow a method to be added to an object, including a class or a type, after it has been compiled.
When creating extension methods, you would typically use static methods within static classes and the “this” modifier. Although extension methods are static, they can be invoked as if they were instance methods. However, extension methods cannot be defined for static classes, and they cannot override methods of a class or interface. If an extension method has the same name and signature as a class or interface method, it will not be called. Additionally, at compile time, instance methods defined in the type itself will always have a higher priority than extension methods.
Extension methods are actually widely used in C# development and is often leveraged indirectly through popular libraries like LINQ, which uses extension methods to add query functionality to existing types such as System.Collections.IEnumerable and System.Collections.Generic.IEnumerable . This allows developers to query collections with great ease and flexibility. For example:
The following logic is performed behind the scenes (simplified code of Where and Select LINQ methods):
Extension methods provide a more convenient way of writing code, leading to improved readability and discoverability. For example, consider the following two code snippets:
The following extension methods use cases are among the most popular:
1) The most significant benefit of using extension methods is that they provide a convenient means of adding extra features to the .Net Framework or third-party classes that cannot be modified or don’t have access to the source code. For example:
2) Extending the functionalities on a DTO. For example:
3) Collection Functionality. This is basically what we have seen higher. They allow the functionality to be called from any collection such as an System.Array or System.Collections.Generic.List that implements System.Collections.Generic.IEnumerable on that type.
In conclusion, extension methods in C# provide a powerful and flexible way to extend existing types without modifying their source code. They enable developers to add new functionality to types, including third-party types or types that are part of the .NET framework. Extension methods also make code more readable by allowing developers to write fluent and concise code. However, it is important to use extension methods judiciously and avoid creating ambiguous or confusing code. When used appropriately, extension methods can be a valuable tool for improving code quality and maintainability in C#.
Top comments (0)
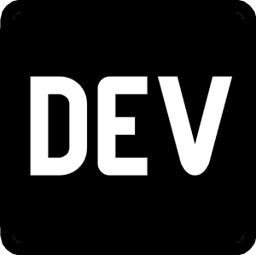
Templates let you quickly answer FAQs or store snippets for re-use.
Are you sure you want to hide this comment? It will become hidden in your post, but will still be visible via the comment's permalink .
Hide child comments as well
For further actions, you may consider blocking this person and/or reporting abuse
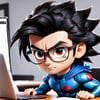
Optimizing CPU Load in C#: Key Approaches and Strategies
Devs Daddy - Apr 19
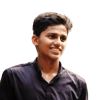
Why do modern projects prefer Microservices architecture?
Joel Jose - Apr 18
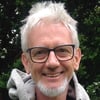
OpenAI and Assistants
john - Apr 17
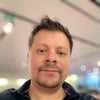
ObservableRangeCollection in .NET MAUI
Victor Hugo Garcia - Apr 16
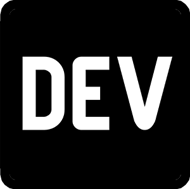
We're a place where coders share, stay up-to-date and grow their careers.
C# Extension Methods: Simplifying Code and Boosting Efficiency
C# extension methods are a powerful feature that allows developers to add new methods to existing types without modifying the original code. This means that developers can create new functionality for classes, structs, and interfaces without having to create a new derived type or recompile the original type. Extension methods are static methods that can be called as if they were instance methods on the extended type, making them easy to use and understand.
One of the benefits of using C# extension methods is that they can be added to any class, including .NET framework classes, third-party classes, and custom classes. This means that developers can extend the functionality of existing code without having to modify the original source code. Extension methods can be used to simplify complex code, improve readability, and reduce the amount of code that needs to be written.
To create an extension method in C#, developers need to define a static class and a static method within that class. The first parameter of the method must be preceded by the ‘this’ keyword, which indicates the type being extended. The method can then be called on any instance of that type, as if it were a member of that type. C# extension methods are a powerful tool for developers, providing a way to add new functionality to existing code without modifying the original source.
Table of Contents
What are Extension Methods?
Extension methods are a feature in C# that allows developers to add new methods to existing types, including those defined in the .NET Framework. They are defined as static methods but are called using instance method syntax. This means that they appear as if they were instance methods of the extended type, although they are not actually part of the type’s definition.
Extension Method Syntax
The syntax for defining an extension method is straightforward. First, the method must be defined in a static class. The class must be marked as static, and the method must also be marked as static. The first parameter of the method must be preceded by the this keyword, which specifies the type that the method extends. This parameter represents the instance that the method is called on.
Here is an example of an extension method that adds a Reverse method to the string type:
With this extension method defined, any string instance can now call the Reverse method as if it were a built-in method of the string type:
Extension methods can be defined in any namespace, but they are only in scope when the namespace is explicitly imported with a using directive.
In summary, extension methods allow developers to add new methods to existing types without modifying the original type’s definition. They are defined as static methods in a static class, and the first parameter is preceded by the this keyword to specify the type that the method extends.
Advantages of Extension Methods
Extension methods offer several advantages to C# developers. Here are some of the benefits of using extension methods:
Extending Functionality
Extension methods allow developers to add new functionality to an existing class or interface without modifying its source code. This can be useful when you want to add a new feature to a class that you don’t have access to modify, such as a third-party library. By extending the functionality of an existing class, you can avoid the need to create a new class or modify the existing one.
Code Readability
Extension methods can improve the readability of your code. By using extension methods, you can make your code more concise and easier to read. For example, instead of calling a utility method with the class instance as a parameter, you can call the method as if it were an instance method of the class. This can make your code more readable and easier to understand.
Modifying Values
Extension methods can also be used to modify the values of an existing class. This can be useful when you need to modify a class that you don’t have access to modify. By using an extension method, you can modify the value of an existing class without changing its source code.
Private Access
Extension methods can access private members of a class. This can be useful when you need to access private members of a class but don’t want to modify its source code. By using an extension method, you can access private members of a class without breaking encapsulation.

Avoiding This Keyword
Extension methods can also be used to avoid the use of the “this” keyword. This can be useful when you want to call a method without specifying the instance of the class. By using an extension method, you can call a method as if it were a static method, without specifying the instance of the class.
Extension methods are used extensively in LINQ (Language Integrated Query). LINQ provides a powerful way to query data from different data sources, such as collections, arrays, and databases. By using extension methods, you can extend the functionality of LINQ and write more concise and readable code.
Overall, extension methods provide several advantages to C# developers. By using extension methods, you can extend the functionality of an existing class, improve the readability of your code, modify values, access private members, avoid the use of the “this” keyword, and write more concise and readable code in LINQ.
Using Extension Methods
Extension methods are a powerful feature of C# that allows developers to add functionality to existing types without modifying them. This section will cover how to use extension methods and the considerations that come with them.
Using Directives
To use an extension method, a using directive for the namespace in which the method is defined must be added to the code. If the extension method is defined in a top-level static class, the namespace can simply be added to the using directive. If the extension method is defined in a nested class, the namespace of the containing class must be added to the using directive.
Instance Methods
Extension methods can be used to add instance methods to a type. However, it is important to note that an extension method with the same name and signature as an instance method will not be called. This means that extension methods cannot be used to override existing methods.
Recompiling
Extension methods can be added to any type, including .NET framework classes and third-party classes. This means that developers can add functionality to types that they do not have control over. However, it is important to note that if the type is updated, the extension method may no longer work. This is because the extension method is compiled against the original version of the type and not the updated version.
In summary, using extension methods can greatly increase the functionality of existing types in C#. However, it is important to use them carefully and consider the implications of adding functionality to types that are not under your control.
Creating Custom Extension Methods
To create a custom extension method in C#, you need to define a static class with at least one static method. The static method should have the this keyword followed by the type that it will extend as the first parameter. The following is the syntax for creating an extension method:
Here are some examples of creating custom extension methods in C#:
In the first example, a custom extension method is created for the IEnumerable<T> interface. The method is called CustomWhere and it takes a Func<T, bool> predicate as a parameter. The method returns an IEnumerable<T> that contains only the elements that satisfy the predicate.
In the second example, a custom extension method is created for the string class. The method is called CustomReverse and it returns a reversed version of the input string.
Scope and Behavior
Extension methods are only visible within the namespace in which they are defined. They can be called on instances of the original class just like any other method. Extension methods cannot access protected fields or events of the original class.
When you create an extension method, you are essentially adding behavior to the original class without changing its structure. This can be useful when you want to add functionality to a class that you cannot modify, such as a class in a third-party library.
Visual Studio Integration
Visual Studio makes it easy to create extension methods. When you create a static class with a method that has the this keyword followed by a type, Visual Studio will automatically recognize it as an extension method and provide IntelliSense for it.
Changes in C# Programming Guide
Extension methods were introduced in C# 3.0 and have been an important feature of the language ever since. The C# Programming Guide has been updated to include information on how to create and use extension methods.
Limitations of Extension Methods
Extension methods in C# provide a way to add methods to existing types without modifying the original type. However, there are certain limitations to extension methods that developers should be aware of.
Access to Private Members
One of the main limitations of extension methods is that they cannot access private members of the extended type. This is because extension methods are static methods and cannot access the internal state of the class. As a result, developers must use instance method syntax to access private members.
LINQ Standard Query Operators
Another limitation of extension methods is that they cannot be used to create new LINQ standard query operators. While extension methods can be used to extend existing LINQ operators, they cannot be used to create new ones. This is because LINQ standard query operators require the use of instance methods.
Static Members
Extension methods cannot be used to extend static members of a class. This is because extension methods require an instance of the class to be called, and static members do not have instances.
Overriding Existing Methods
Extension methods cannot be used to override existing methods of the extended type. This is because extension methods are static methods and cannot be used to override instance methods.
Granularity
Another limitation of extension methods is that they are imported at a namespace level, which can lead to a lack of granularity. Developers cannot import a single extension method from a namespace without importing all the other methods as well.
In conclusion, while extension methods in C# provide a convenient way to add methods to existing types, developers should be aware of their limitations. These limitations include the inability to access private members, create new LINQ standard query operators, extend static members, override existing methods, and lack of granularity.
Best Practices for Extension Methods
Naming conventions.
When creating custom extension methods, it is important to follow naming conventions that are clear and concise. The name should reflect the functionality of the method and should not be too long or too short. It is recommended to use a verb as the first word in the method name, followed by a noun that describes the action being performed. For example, “SortAscending” or “FilterByCategory”.
Parameter Binding
When binding parameters to an extension method, it is important to use the correct type and to ensure that the parameter is not null. If the parameter is a reference type, it should be checked for null before using it in the method. If the parameter is a value type, it should be passed by value rather than by reference.
Properties vs. Parameters
When designing an extension method, it is important to consider whether to use properties or parameters. Properties are useful for storing data that is used across multiple methods, while parameters are useful for passing data between methods. It is recommended to use properties when the data is used across multiple methods and to use parameters when the data is only used within a single method.
When extending a class, it is important to consider whether to use a custom class or to extend a .NET Framework class. If a custom class is used, it should be derived from the appropriate .NET Framework class to ensure that it has the necessary functionality. If a .NET Framework class is extended, it should be done in a way that does not break the existing functionality of the class.
When creating additional methods in a console application or a class library, it is important to ensure that the methods are well-documented and easy to understand. The programmer should provide clear and concise descriptions of each method and should include examples of how the method can be used.
Overall, following these best practices can help ensure that extension methods are well-designed, easy to use, and provide useful functionality to the programmer.
Related posts:
- C# Optional Parameters: Simplify Your Code with Flexibility
- Proposed Default Interface Methods In C# 8
- Simplifying Multithreaded Scenarios With PostSharp Threading
- .NET Core Dependency Injection Lifetimes Explained
Leave a Comment Cancel reply
Save my name, email, and website in this browser for the next time I comment.
Study through a pre-planned curriculum designed to help you fast-track your DotNet career and learn from the world’s best collection of DotNet Resources.
Find us on social media:
As Featured On

Contact: [email protected] | Phone Number: (973) 916-2695 | Address: 288 Rosa Parks Blvd, Paterson, New Jersey 07501, USA
Disclaimer: Efforts are made to maintain reliable data on all information presented. However, this information is provided without warranty. Users should always check the offer provider’s official website for current terms and details. Our site receives compensation from many of the offers listed on the site. Along with key review factors, this compensation may impact how and where products appear across the site (including, for example, the order in which they appear). Our site does not include the entire universe of available offers. Editorial opinions expressed on the site are strictly our own and are not provided, endorsed, or approved by advertisers.
2022 © DotNetCoreTutorials All rights reserved.

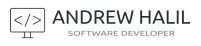
andrewhalil.com
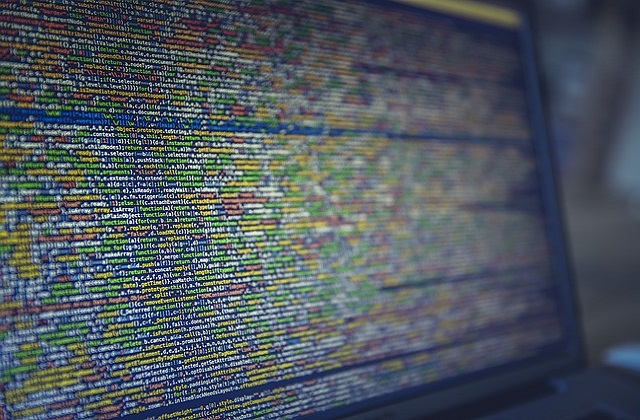
Using Extension Methods in a .NET Core Application
Welcome to today’s post.
In today’s post I will be discussing extension methods and how they can be used in a .NET Core application.
Before I go into the details of how they are used within ASP.NET Core applications, I will give a brief overview of what an extension method is, why they are used, and the rules behind their use. I will also provide a basic example of how they are used with an example in C#.
What is an Extension Method?
Extension methods have already been part of the C# language library since they were introduced in version 3.0. Custom extension methods allow you to create additional methods to types without having to create methods within new derived types.
Custom extension methods allow you to create additional methods to types without having to create methods within new derived types.
For example, we want to create a method to compute the number of alphanumeric characters within a string, AlphanumericCount(). We can create this as a method within a new class, which we would have to build and use separately from our String type.
Extension types that allow query methods for enumerable types are provided by the System.Linq library.
Creating Extension Methods
Each extension method follows the rules:
- The method must be under a namespace.
- The method must be under a public static class.
- The method must be public visibility of static type.
- The method must contain a parameter with the type being extended and preceded by this modifier.
For example, our new string extension method would be implemented as shown:
To use the above extension method, declare the namespace, StringExtensionMethods then declare a variable of the type that is extended and use the extension method:
Note : Extension methods cannot access private or protected members of the extended class. Also, extension methods cannot override methods within the extending class.
Using Extension Methods within the .NET Core Web Application Builder Pipeline
In the application pipeline IAppBuilder within ASP.NET Core , we can make use of useful middleware extensions, including those for authentication, authorization, http requests. For example, to enable authentication we call the following extension method:
Authentication is then handled by the authentication service middleware service, IAuthenticationService . This allows our ASP.NET Core web application to authenticate users with Cookie based or JWT Bearer based tokens. In one of my previous posts, I show how to implement JWT token authentication in ASP.NET Core applications .
Extending Error Handlers within a .NET Core Web API
In a .NET Core API service application, the use of extension methods is quite abundant within the application builder pipeline. In particular, the use of the UseExceptionHandler() extension method middleware for handling errors is one of the more useful middleware extensions we can utilise to delegate our application errors, filter the error, then output the error response is a more user-friendly way to the caller.
To be able to implement an error handler extension method, we use the above extension method guidelines. A general exception handler is used in the production environment where we wish to sanitise the error instead of displaying the full error and stack trace, which might contain sensitive details.
Below is an example general extension handler (as a lambda function) we use within the Configure() method of the startup.cs file:
The above error handler looks quite cumbersome, so we can move the error handler into a it’s own custom extension method, which is the error handler.
We implement a custom.NET Core middleware extension class as follows:
We also include a model to encapsulate the status code and error message:
To use the middleware extension method from the startup.cs we do this as shown:
I have omitted logging in the above example. In a future post I will be discussing using the general exception handling with logging.
The above details have provided you with an overview of what extension methods are and how they are used in a few common scenarios, such as enabling security middleware and extending the exception handling middleware within an ASP.NET Core web application or ASP.NET Core web API application.
That’s all for today’s post.
I hope you found this post useful and informative.
Andrew Halil is a blogger, author and software developer with expertise of many areas in the information technology industry including full-stack web and native cloud based development, test driven development and Devops.
Similar Posts
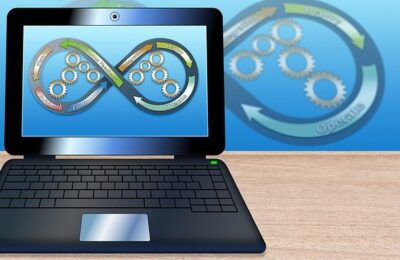
How to Publish a .NET Core Application into a Docker Hub Container Registry
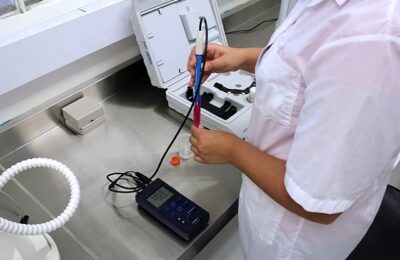
An Overview of Error and Status Handling of HTTP Requests in a .NET Core Web API Application
InfoQ Software Architects' Newsletter
A monthly overview of things you need to know as an architect or aspiring architects.
View an example
We protect your privacy.
Facilitating the Spread of Knowledge and Innovation in Professional Software Development
- English edition
- Chinese edition
- Japanese edition
- French edition
Back to login
Login with:
Don't have an infoq account, helpful links.
- About InfoQ
- InfoQ Editors
Write for InfoQ
- About C4Media
Choose your language
Special Memorial Day Sale with significant discounts of up to 60% off . Register now.
Get practical advice from senior developers to navigate your current dev challenges. Use code LIMITEDOFFERIDSMUNICH24 for an exclusive offer.
Level up your software skills by uncovering the emerging trends you should focus on. Register now.
Your monthly guide to all the topics, technologies and techniques that every professional needs to know about. Subscribe for free.
InfoQ Homepage News Azure API Center Now Generally Available
Azure API Center Now Generally Available
May 16, 2024 2 min read
Steef-Jan Wiggers
Microsoft has announced Azure API Center 's general availability (GA), which offers centralized API inventory for easy API discovery, reuse, and governance regardless of API type, lifecycle stage, or deployment location.
With the GA release of Azure API Center, a follow-up of the earlier public preview , developers can now leverage its features to build a complete and current API inventory. This includes enforcing API design best practices at scale, streamlining development with improved developer experiences, and API discovery through the API Center Portal.
An essential capability of Azure API Center is managing API inventory with enhanced visibility and governance. It offers built-in metadata , including title, description, type, lifecycle stage, owner, version, deployment, and specification information. Developers can create their API inventory using the Azure portal, Azure CLI , or Visual Studio Code .
Furthermore, API Center is not just a standalone tool but a fully integrated solution. It seamlessly integrates with CI/CD pipelines in GitHub and Azure DevOps, ensuring the developers’ organization API inventory stays current. Additionally, API Center's API Analysis tool helps enforce API design best practices and consistency across your organization.
The Visual Studio Code extension streamlines API discovery and consumption. It makes it easy for developers to browse and search the organization's API catalog, view API documentation inline, and test APIs using industry-standard tools. Additionally, developers can generate SDKs for their preferred languages.
The API Center Portal , a web-based discovery and engagement portal for APIs, allows API Platform teams to provide API consumers with a seamless API discovery and consumption environment. With the portal, they can browse, filter, and search for APIs, import them into their favorite developer tools, or explore them in the API Center extension for Visual Studio Code.
API Center Portal (Source: Azure Tech Community blog post )
Justin Yoo , a cloud advocate at Microsoft, concluded in a blog post on Azure API Center:
Managing many APIs in one place is crucial as your organization grows. You might think you don't need Azure API Center if your organization's API structure is relatively simple. However, even if your organization is small, Azure API Center will give you a better overview of APIs and how they can be interconnected.
In addition, Wessel Beulink , a cloud architect at Rubicon, concluded in his blog post on Azure API Center:
By adopting Azure API Center and its complementary tools, organizations can enhance their API management practices, boost developer productivity, and unlock new opportunities for innovation and growth. As an Azure Solution Architect, the key to success lies in understanding the strategic importance of APIs in your digital transformation journey and leveraging the right tools and practices to manage them effectively.
Lastly, Azure API Center is available in the Australia East, Central India, East US, UK South, and West Europe Azure regions. Details of the service are also available on the documentation landing page .
About the Author
Rate this article, this content is in the cloud topic, related topics:.
- Development
- Architecture & Design
- Microsoft Azure
- API Strategy&Practice
Related Editorial
Related sponsored content, popular across infoq, how to build and foster high-performing software teams: experiences from engineering managers, typespec: a practical typescript-inspired api definition language, if llms do the easy programming tasks - how are junior developers trained what have we done, java news roundup: new jeps, payara platform, spring boot 10th anniversary podcast, rust-written borgo language brings algebraic data types and more to go, unraveling the enigma: debunking myths surrounding lambda cold starts, related content, the infoq newsletter.
A round-up of last week’s content on InfoQ sent out every Tuesday. Join a community of over 250,000 senior developers. View an example
How we used Java Flight Recorder to resolve authentication issues
A success story with Java Flight Recordings (JFR) and Red Hat Technical Account Management (TAM)

Imagine one of the following scenarios:
- Your kids are going on vacation with their grandparents; you want to increase their cell phone plan with more bandwidth and gigabytes so you can see them enjoy the beach through a video call with your parents.
- You’re on a business trip and you're relying on your company’s telco provider for roaming.
But you can’t do either of those things, because there’s an issue with the mobile application provider. Enter Red Hat Technical Account Management to assist in this situation.
Diagnosing the problem
Let’s add a little more context. A telecommunications service provider (telco) provides a mobile application whose front end targets Red Hat's single-sign on (SSO) tool to authenticate and authorize users to either pay plans or enlarge plans.
The issue occurred after the company decided to "eliminate certain users." But something else had changed in the production system, and nobody knew what that was.
After realizing that there was more to the situation than just deleting users, all parties agreed that the priority was to resuscitate production systems. This meant having the single sign-on tool up and running. The steps taken meant scaling down both Red Hat single sign-on pod and the PostgreSQL pod. Once the database pod was up and running, the next step was tuning the requests and limits (because SSO runs on top of Red Hat OpenShift ) and changing the garbage collection algorithm.
Here’s a little snippet of what the Technical Account Management team proposed (note these are not the exact values used and might not be suitable to your needs):
Environment variables JAVA_MAX_MEM_RATIO and JAVA_INITIAL_MEM_RATIO are added to reserve more heap of the requested memory; by default, the Java images and the heap only take a quarter of the memory assigned to the pod.
The environment variable JAVA_OPTS_APPEND is added to change the default garbage collection algorithm to use G1.
So, after proposing these changes and rolling out the pods, the production system was up and running and everyone was happy with the result. The telco's customers managed to authenticate and use the application for a while. But after a couple of hours, the team realized there was an issue between the mobile front-end application and the single sign-on technology. So, the Technical Account Manager was then summoned back to the war room.
The challenge was that no one knew where the issue was occurring. After much debate with the developers of the application, it was understood that the issue was between the code on the front end and Red Hat's single sign-on technology. They reported it as a loop in between these two components.
From the Red Hat single sign-on side, it was relevant to prove to the telco which URL or method was invoked so that they could point it on their code.
Collecting data using Java Flight Recorder
The Technical Account Manager proposed using Java Flight Recorder (JFR), which lets you see “under the hood” when the systems are productive. Java Flight Recorder is an open source tool that is bundled with the JDK, meaning it has a very low overhead. JFR is a feature available in OpenJDK builds, including the Red Hat build of OpenJDK. Therefore, it is possible to start capturing data with a “live pod.” More details on this knowledge-centered service (KCS): How to use JDK Flight Recorder (JFR)?
The team had two choices:
- Edit the JAVA_OPTS_APPEND to include certain parameters so that when the pod begins it starts recording (something like JAVA_OPTS_APPEND= -XX:-UseParallelOldGC -XX:+UseG1GC -XX:+ExplicitGCInvokesConcurrent -XX:MaxGCPauseMillis=500 -XX:StartFlightRecording=duration=200s,filename=flight.jfr ) This means that the pods would be rolled out with this configuration and only start a 200 seconds recording.
- Start all of the replicas of Red Hat single sign-on, connect to each pod via a terminal (Terminator, to be specific) , and send the signals to start the recording.
The solution
Option 2 is the better choice, because the recording starts when the front end pod of the mobile app is started, and allows us to capture data we need once the external front-end pod is started. (Details on how to connect to a live pod are provided on the KCS; basically, grab the Java PID (the command $JAVA:_HOME/BIN/jcmd will output the process ID of Java processes), and then on the same terminal, execute the following command:
After the capture process is finished, you must send the signals to jfr.stop and jfr.dump to write the contents to disks, making sure they are not corrupt. The command-line tool for OpenShift client oc fits perfectly with all of our command executions ( rsh to connect to pods and execute the JFR commands). We can use oc rsync to extract the JFR files from the path we used. Once these files are in your local file system, you can analyze them with the Java Mission Control tool.
The recording for the front-end pod can’t be publicly shown, but it was revealed that there was a bug on the front-end application (and many others), which caused the loop on the authentication process. This was evident to see in the method profiling and the times accumulated in the methods. Once these were detected and hotfixed, the application worked perfectly. Red Hat's single sign-on tool and Technical Account Management were key to the success of this situation.
As a bonus, the Java Mission Control GUI revealed the already explained need to update the product, as shown in Figures 1 and 2. This data revealed certain exceptions and excessive times on classes related to Infinispan, a key component to cache sessions.
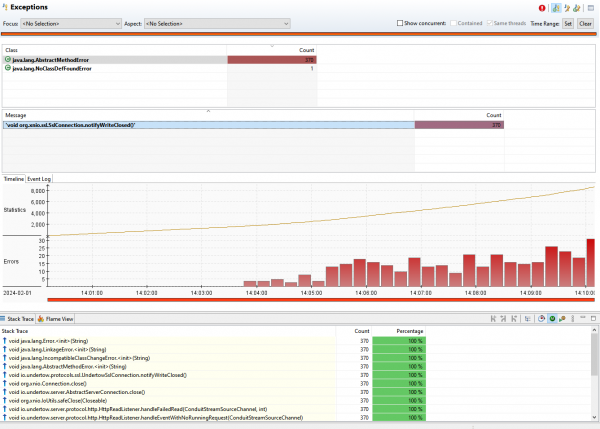
- Red Hat Enterprise Linux
- Red Hat OpenShift
- Red Hat Ansible Automation Platform
- See all products
- See all technologies
- Developer Sandbox
- Developer Tools
- Interactive Tutorials
- API Catalog
- Operators Marketplace
- Learning Resources
- Cheat Sheets
Communicate
- Contact sales
- Find a partner
Report a website issue
- Site Status Dashboard
- Report a security problem
RED HAT DEVELOPER
Build here. Go anywhere.
We serve the builders. The problem solvers who create careers with code.
Join us if you’re a developer, software engineer, web designer, front-end designer, UX designer, computer scientist, architect, tester, product manager, project manager or team lead.
Red Hat legal and privacy links
- About Red Hat
- Contact Red Hat
- Red Hat Blog
- Diversity, equity, and inclusion
- Cool Stuff Store
- Red Hat Summit
- Privacy statement
- Terms of use
- All policies and guidelines
- Digital accessibility

IMAGES
VIDEO
COMMENTS
Extension methods enable you to "add" methods to existing types without creating a new derived type, recompiling, or otherwise modifying the original type. Extension methods are static methods, but they're called as if they were instance methods on the extended type. For client code written in C#, F# and Visual Basic, there's no apparent ...
Extension methods allow you to inject additional methods without modifying, deriving or recompiling the original class, struct or interface. Extension methods can be added to your own custom class, .NET framework classes, or third party classes or interfaces. In the following example, IsGreaterThan() is an extension method for int type, which ...
Let us understand Extension Methods in C# with an example. First, create a console application and then add a class file with the name OldClass.cs and then copy and paste the following code into it. As you can see in the below code, here we created the OldClass with one data member i.e. x, and two methods i.e. Test1 and Test2.
In C#, the extension method concept allows you to add new methods in the existing class or in the structure without modifying the source code of the original type and you do not require any kind of special permission from the original type and there is no need to re-compile the original type. It is introduced in C# 3.0.. Let us discuss this concept with the help of an example.
LINQ Extension Method in C#: LINQ (Language Integrated Query) extension methods in C# are a set of methods provided by the System.Linq namespace that extends the capabilities of collections (like arrays, lists, and other types implementing IEnumerable<T>) by adding query functionality. These methods enable querying collections using a SQL-like ...
To add a method to a class, we often subclass the existing class and add the method to the derived class. C# introduced a new feature called extension methods that allows you to add new methods to an existing type (or class) without the need of using inheritance. An extension method is a static method but it is called as if it was an instance ...
Extension methods are a powerful feature (introduced in C# 3.0) in object-oriented programming languages that allow a method to be added to an object, including a class or a type, after it has been compiled. When creating extension methods, you would typically use static methods within static classes and the "this" modifier.
Extension methods are an elegant way of introducing new functionality to classes without having to change the classes themselves. In order to create an extension method, we are required to create a static class with static methods. The first parameter in the static method must have the this keyword before the type.
C# extension methods are a powerful feature that allows developers to add new methods to existing types without modifying the original code. This means that developers can create new functionality for classes, structs, and interfaces without having to create a new derived type or recompile the original type. Extension methods are static methods ...
public double Add(double num1, double num2) return num1 + num2; // Below is the extension class which have one extension method. // It is extension method and it's first parameter is a calculator class.It's behavior is going to extend. public static double Division(this Calculator cal, double num1,double num2){.
C# Extension Method. C# extension method is a static method of a static class, where the "this" modifier is applied to the first parameter. The type of the first parameter will be the type that is extended. Extension methods are only in scope when you explicitly import the namespace into your source code with a using directive.
To use the above extension method, declare the namespace, StringExtensionMethods then declare a variable of the type that is extended and use the extension method: 1. 2. 3. using StringExtensionMethods; string myString = "I am over 21 years of age."; int intVal = myString.AlphanumericCount(); Note: Extension methods cannot access private or ...
The syntax for calling an extension method may look like a class method (e.g. myObj.Foo() ), but it's pure syntactic sugar to hide the real static call that's happening (e.g. MyHelper.Foo(myObj) ). When statics methods are pure, i.e. only input/output driven without any state or side effects (as statics should be), you can simply use the real ...
Found. Redirecting to /en-US/docs/Mozilla/Firefox/Writing-extension/Writing-a-C
The Visual Studio Code extension streamlines API discovery and consumption. It makes it easy for developers to browse and search the organization's API catalog, view API documentation inline, and ...
66. To extend any class. public static T DoSomething<T>(this T obj) //... To extend a specific generic class. //... For me I forgot the <T> after the NeedExtension. More specifically I wanted to write an extention for the generic Dictionary class, so I needed <TKey,TValue>.
From the Red Hat single sign-on side, it was relevant to prove to the telco which URL or method was invoked so that they could point it on their code. Collecting data using Java Flight Recorder. The Technical Account Manager proposed using Java Flight Recorder (JFR), which lets you see "under the hood" when the systems are productive.
18. I think you are trying to write a extension method to encrypt the password with a key. So you function header should be: public static string ToEncrypt(this string passWord, string key) Later you can use this extension like below: string encrpted = password.ToEncrypt("your key here");
Using extension methods on an enum would make me feel dirty. Create a class to encapsulate what is needed. Keep an enum as simple as possible. If you need more logic associated with it, then create a Duration class that exposes day, week, month plus contains any other logic that would have been in the extension method. -
0. In C # and Java, each time a method is called, it is a new stack frame. Does that mean that from memory, these two methods are not the same, even if their contents are the same. When it comes to dependency injection, I used Singleton instead of Transient because I believe it is stateless for services and using singleton injection should ...