Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.

Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications
REST API plugin for Unreal Engine 4 - we love restfull backend and JSON communications!
ufna/VaRest
Folders and files, repository files navigation.
VaRest is the plugin for Unreal Engine 4 that makes REST server communications easier to use.
Key features:
- Flexible Http/Https request management with support of different Verbs and Content Types
- No C++ coding required , everything can be managed via blueprints
- Blueprintable FJsonObject wrapper with almost full support of Json features: different types of values, arrays , binary data content, both ways serializarion to FString, etc.
- Blueprintable FJsonValue wrapper - full Json features made for blueprints!
- Both bindable events and latent functions are provided to control the asynchronous requests
Check the Wiki for plugin usage examples and installation notes.
Current version: 1.1 R 33 (UE 5.2)

Unreal® is a trademark or registered trademark of Epic Games, Inc. in the United States of America and elsewhere.
Unreal® Engine, Copyright 1998 – 2021, Epic Games, Inc. All rights reserved.
Releases 31
Contributors 24.
Learn How To Get Data From API in Unreal Engine 5 by Building a Virtual Art Gallery
Learn in a fun way about APIs. This Unreal Engine C++ tutorial will guide you through making HTTP requests, deserializing JSON responses, extracting data from complex structures, and dynamically rendering images fetched from the internet using transient textures and dynamic material instances.
Marian Pekár
Read more posts by this author.
In today's post, I'd like to show you how to retrieve an image provided by The Art Institute of Chicago via its public API , how to create a texture from this image, and how to feed this texture to a material and render it on a plane accompanied by a floating text with title, name of the artist and some other details.
To achieve that, we're going to write a custom C++ class derived from Actor , with UStaticMeshComponent and UTextRenderComponent both attached to a USceneComponent set as a RootComponent .
The key property of this class will be CatalogId , an integer that tells which artwork to fetch from the collection of the above-mentioned art institute, using an HTTP request.
We'll be able to build an entire virtual gallery using just this single custom actor. Adding a new artwork would be as simple as placing an instance of the actor into our map and setting the CatalogId .
However, I like my tutorials to be beginner friendly even when it covers slightly advanced topics. Let's take a step back now and talk a little bit about what is an API.
If you're already well familiar with the term, feel free to skip the following few paragraphs and dive straight into the implementation from Preparing a new Unreal Engine project .
What Is an API?
For the rest, API stands for Application Programming Interface. It provides a set of instructions that enable different systems to communicate with one another, specifying the methods for sending and receiving data, as well as the actions that can be performed.
In our scenario, we have two systems, one is the application we're going to build, using the Unreal Engine, and the other is a web server that provides an API for retrieving data from a database of The Art Institute of Chicago (ARTIC) .
The web server acts as an API provider, while our application serves as its consumer. As long as a consumer makes a valid request, the API provider doesn't concern itself with who the consumer is or what they plan to do with the data; it simply provides it.
Actually, we could build a similar project in Unity or use an API from any other application that can make an HTTP request, such as a web browser. You can make a request right now by simply clicking on the following link: https://api.artic.edu/api/v1/artworks/ 129884 When you clicked the link, your web browser made a GET request using the HTTP protocol and received a response containing data in JSON (JavaScript Object Notation) format.
Upon examining the JSON data¹ that you received, you'll notice that it contains information about Alma Thomas's artwork, Starry Night and the Astronauts , which is stored in the ARTIC collection with an ID of 129884 .
1) To make the JSON data more readable, you may want to install a browser extension, such as "JSON Prettifier" or "JSON Formatter." These extensions will format the data in a more organized and easy-to-read manner.
How does this work? How can we retrieve and utilize data from a single API that doesn't differentiate between a web browser and an Unreal Engine application? The answer lies partly in the previous paragraphs.
This is because the API provides data using a standardized protocol and format. Your web browser knows how to send a request using the HTTP protocol and how to handle the response.
Fortunately, Unreal Engine is also equipped to handle this. It includes an HttpModule and JSON Serializer that we'll use to retrieve and parse data, respectively.
There's much more to learn about APIs and the technology behind them, including various protocols and data formats such as XML and others. Of significance is the fact that both requests and responses have headers , although I didn't cover them in this brief introduction.
Some APIs require authentication, which opens up another deep rabbit hole to explore. It's also worth noting that not all APIs are web APIs, and there's something called REST (Representational State Transfer) , which is a specific architectural style and widely used approach for building APIs.
Preparing a New Unreal Engine Project
Now let's start building something. If you want to follow along (and I highly recommend that you do), begin by creating an empty C++ project in Unreal Engine 5.1.1 .
It might work on other versions too, but version 5.1.1 is the one I've built the example project , so I cannot guarantee that it would also work on, for instance, version 4.27.
I've named my project FetchArt , but feel free to choose any name you like.
Creating a Material
We won't be needing any starter content, but we do require a material that we'll use to render texture to a plane later on.
In the Content Browser , create a new folder by right-clicking and selecting New Folder . Name the folder Materials . Then, create a new material by selecting New Material from the same context menu. Name the material ExampleMaterial .
Open the material and navigate to the Material Graph . From there, right-click inside the graph and search for Texture Sample . Once the TextureSample node appears, click on it to add it to the Material Graph .
Finally, right-click on the Texture Sample node and select Convert to Parameter . This will convert the Texture Sample node into a parameter node, allowing us to easily change the texture used by the material later on.
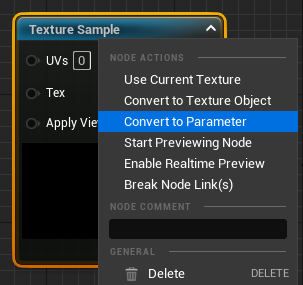
When converting the Texture Sample node into a Texture Parameter node, make sure to name the parameter TextureParameter and double-check the spelling. This is important because we'll be referencing this parameter by name in our C++ code.
With the Texture Parameter node selected, look for the Material Expression Texture Base section in the Details panel on the bottom left of the editor. To assign a default material, click on the small curved arrow icon located on the right side of the Param row.
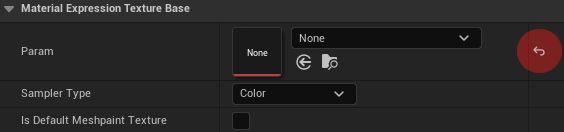
Finally, connect the TextureParameter output pin to the Base Color input pin of the ExampleMaterial . Make sure to save the material before closing the window or tab.
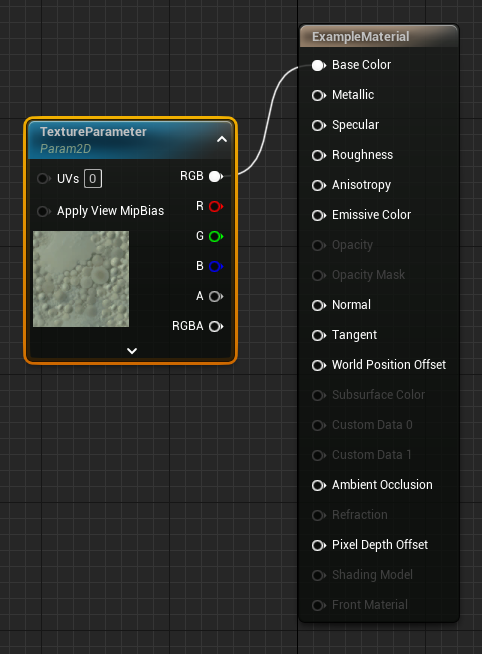
Adding a Custom Actor Class
In the Content Browser , enter the C++ Classes folder of your project. Right-click on the folder and select Add New C++ Class . From the pop-up window, select Actor as the parent class and name the class RemoteImagePlane .
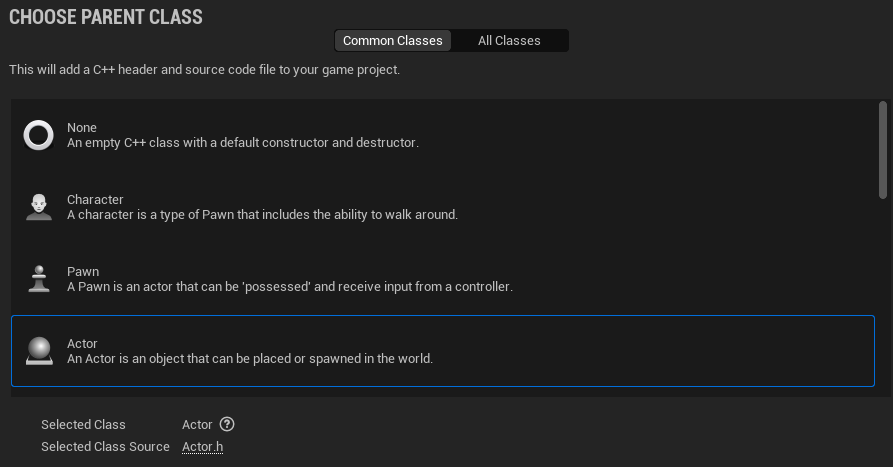
Importing Modules
Time to write some code. First, to prevent linker errors, you need to specify the modules that our project depends on in {YourProjectName}.Build.cs . This C# file is a part of the Unreal Build Tool (UBT) . When a project is built, UBT reads the file and uses it to generate the necessary build scripts and configuration files.
One typically adds modules when needed in Unreal Engine, but since I have already implemented the code for this tutorial, I already know which modules we will need, which are:
- JsonUtilities
- ImageWrapper
Add these module names to the PublicDependencyModuleNames list as follows:
Header File
Now that the modules have been added to the project, it's time to make changes to our RemoteImagePlane class. Open the header file for this class, which is RemoteImagePlane.h .
This actor doesn't need to run any logic in every tick, so you can safely delete the declaration of the Tick method. Don't forget to also delete the implementation of the method in the source file, RemoteImagePlane.cpp . Additionally, in the constructor change the value of the PrimaryActorTick.bCanEverTick flag from true to false .
Back in the header file, between the #include RemoteImagePlane.generated.h and UCLASS() macro, add the following forward declarations and type definitions:
Under the protected access specifier, declare the two delegate methods as follows:
To implement the desired functionality, our custom Actor requires a few components. These include:
- UStaticMeshComponent to render a plane mesh on which we'll set a dynamically created material instance. The fetched artwork will be rendered on this component as a texture.
- UTextRenderComponent to display the title of the artwork, the name of the artist, and other relevant information about the artwork.
- USceneComponent to be used as a root component and to attach the other two components.
We don't want to attach the UTextRenderComponent to the UStaticMeshComponent or the default root component of the Actor, because it would restrict our ability to adjust the position of the text.
We'll need to modify the scale of the Plane to match the aspect ratio of the fetched artwork. To address these issues, we'll use a USceneComponent as the root component and attach the other two components to it. Declare these three member variables, pointers to the above-mentioned classes, as follows.
Also declare two additional member variables to set the width of the texture for our material and the ID of the artwork we're going to fetch:
The last thing in our header file is a little helper method. This method pulls out properties from a received JSON without repeating the same code multiple times.
Source File
Let's move on to the source file, RemoteImagePlane.cpp . The first step is to include all necessary header files:
In the constructor, where we already set PrimaryActorTick.bCanEverTick to false , create a USceneComponent and set it as the Actor's root component.
To create a UStaticMeshComponent in a similar way, start by finding the Plane mesh among Unreal Engine basic shapes using FObjectFinder . If the search succeeds, set the Plane as the component's static mesh. In case of failure, log an error using the UE_LOG macro. Finally, attach the PlaneComponent to the SceneComponent .
The last thing we need to do in the constructor is to create a UTextRenderComponent and attach it to our SceneComponent as well.
Now that we've created and set the components of our custom Actor, let's move on to the BeginPlay method. After calling Super::BeginPlay() , which invokes the method in the parent class (in our case, the AActor class), we need to create an instance of FHttpModule .
Next, use the HttpModule to create an HttpRequest . Set the request method to GET and construct the RequestURL using the ARTIC Public API route for getting artwork data with our CatalogId member variable as a parameter, and the Content-Type header to application/json .
Finally, bind our delegate method OnResponseReceived , we yet need to implement, to OnProcessRequestComplete and invoke sending of the request using ProcessRequest method of HttpRequest class.
Let's now implement the body of our OnResponseReceived delegate method. First, check if the bWasSuccessful parameter is true and if the Response object is in a valid state. If not, print an error message to the Output Console and return early from the method.
Now when we know our Response is valid, we can get the content data by using its GetContentAsString method. The returned string is in JSON format and needs to be deserialized to a JsonObject using the TJsonReader and static FJsonSerializer::Deserialize method, as follows:
Now that we've deserialized our content into a JsonObject , we can begin extracting data from the object and storing them in FString variables. However, before doing so, let's first implement our helper method, TryGetStringField .
This method takes a JsonObject , a field name, and a reference to an FString variable whose value will be set to the value of the field.
With that done, let's continue where we left off in the OnResponseReceived delegate method.
The JSON response from the ARTIC API is not a flat structure; instead, it looks something like this:
Because of this, we first need to extract the individual data and config blocks, which are also of JsonObject type (yes, JSON objects can be, and often are, composed of multiple inner JSON objects). To do so, use the GetObjectField method.
Now that we have isolated the data block as an individual JSON object DataObject , we can declare FString variables for ImageId , Title , ArtistDisplay , and IIIFUrl , and use our TryGetStringField method to assign their values.
Now we can use the Title and ArtistDisplay strings we retrieved to set the text of our TextComponent . However, before doing so, we need to replace any en dashes with hyphens because there is no glyph for en dashes or many other special characters in the font material the UTextRenderComponent is using by default. We can accomplish this simply by using the Replace method.
Since we're talking about strings, it's worth mentioning there are three types of string in Unreal Engine:
- FString – a mutable type that can be modified at runtime. It is used for general-purpose string handling.
- FName – an immutable type that is used to represent names and identifiers within the engine. It is often used for things like object names and variable names.
- FText – a localized type. It is used for UI text, game messages, and other text that needs to be displayed to the user.
Now that we have set the TextComponent , we can move on to getting the URL of the actual image.
This can be done by concatenating the ImageId we already retrieved from the DataObject with the IIIFUrl we still need to get from the ConfigData , an another JsonObject.
You already know how to achieve that. Pulling IIIFUrl out from ConfigData is not much different from retrieving data from DataObject .
Now we have all the bits for concatenating the ImageUrl .
In the case of the artwork with CatalogId 129884, TextureWidth 512, a and retrieved ImageId e966799b-97ee-1cc6-bd2f-a94b4b8bb8f9 the ImageUrl should be set to https://www.artic.edu/iiif/2/ e966799b-97ee-1cc6-bd2f-a94b4b8bb8f9 /full/ 512 ,/0/default.jpg .
We can use the ImageUrl to make a second HTTP request, this time to retrieve the image data. The syntax for this request is almost identical to the first one, since we are still obtaining data over the HTTP protocol.
The key difference between the two HTTP requests is the delegate method bound to OnProcessRequestComplete . This marks the last piece of logic, we need to implement.
First, in the body of OnImageDownload method, ensure the request was successful and the response is valid, similarly as we did before.
After downloading the image data, it will be stored in memory. However, we still need to do a bit of work in order to render that image on our PlaneComponent .
Start by loading the ExampleMaterial we've created in the editor and creating an instance of UMaterialInstanceDynamic type based on this material.
With the dynamic material instance ready and the binary data of the fetched image stored in memory, the next step is to create an ImageWrapper using the IImageWrapperModule to decode the data.
Now, check if the ImageWrapper is in a valid state. If it is, decompress the image data from the ImageData buffer and store them in another buffer UncompressedBGRA , also declared as TArray<uint8> .
The UncompressedBGRA buffer stores the image data in raw BGRA format, where BGRA stands for the color channels blue, green, red, and alpha (the transparency channel).
We're almost there. Create a transient texture of the width and height taken from ImageWrapper , and the pixel format PF_B8G8R8A8 . The transient means, the texture will be created only in memory, without creating a file on disk and the pixel format is the 32-bit format with 8 bits for each channel (blue, green, red, and alpha).
Set the texture's compression to TC_Default and SRGB flag to true , this flag is indicating that the texture should be gamma-corrected when displayed on a screen. Then, call the AddToRoot method to prevent the object and its descendants from being deleted during garbage collection .
Now create a pointer to texture data, copy the data from UncompressedBGRA buffer and update resource with the new data. This involves sending the texture data to the GPU, where it can be used in rendering.
Great! We now have a texture ready to be assigned to the TextureParameter of our material instance. Once the texture is assigned, we can then set the material instance as the material on our PlaneComponent .
One last thing, not every artwork is a square, in fact, very few artworks has an aspect ratio of 1:1, most of them have different widths and heights and thus different aspect ratios.
That means we need to calculate the aspect ratio of the image and adjust the scale of our plane accordingly.
Fortunately, this is a straightforward process: we simply divide the image height by the image width to obtain the aspect ratio, and then scale the Y dimension of the plane by that value.
This ensures that the artwork will be displayed without any stretching. The width of our plane remains constant, while the height would be scaled proportionally to match the aspect ratio of the image.
Blueprint Class
Our code is now complete, and we can proceed to create a Blueprint class based on our custom C++ class in the Unreal Editor.
To do this, right-click on the class in the Content Browser and select Create Blueprint Class Based on RemoteImagePlane from the context menu. Name the new Blueprint class BP_RemoteImagePlane .
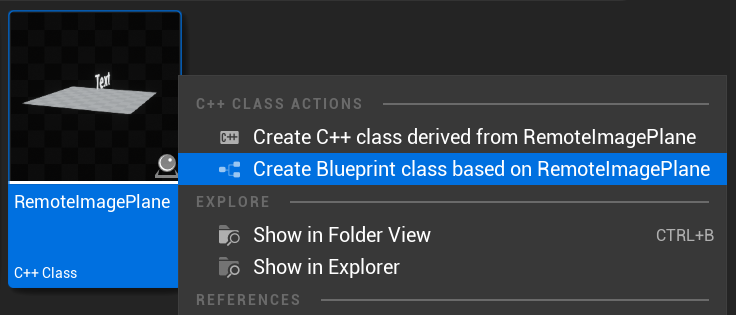
We could have set the default position and orientation of our plane and text in C++ code, or created an instance by drag-and-dropping the class from the Content browser into the map and then edit the values in the Details panel .
However, customizing our actor visually in the Blueprint editor will be much more convenient. To do this, open the BP_RemoteImagePlane in the Full Blueprint editor and select the Plane Component in the Components tab.
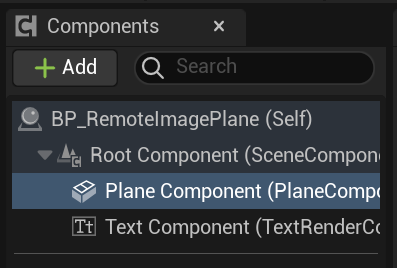
When you have the Plane Component selected, you can adjust its position and orientation using gizmos. To switch between the gizmos for setting the relative position and rotation, you can use the buttons located on the top-right corner of the Viewport tab, or you can use the W and E keys, respectively.
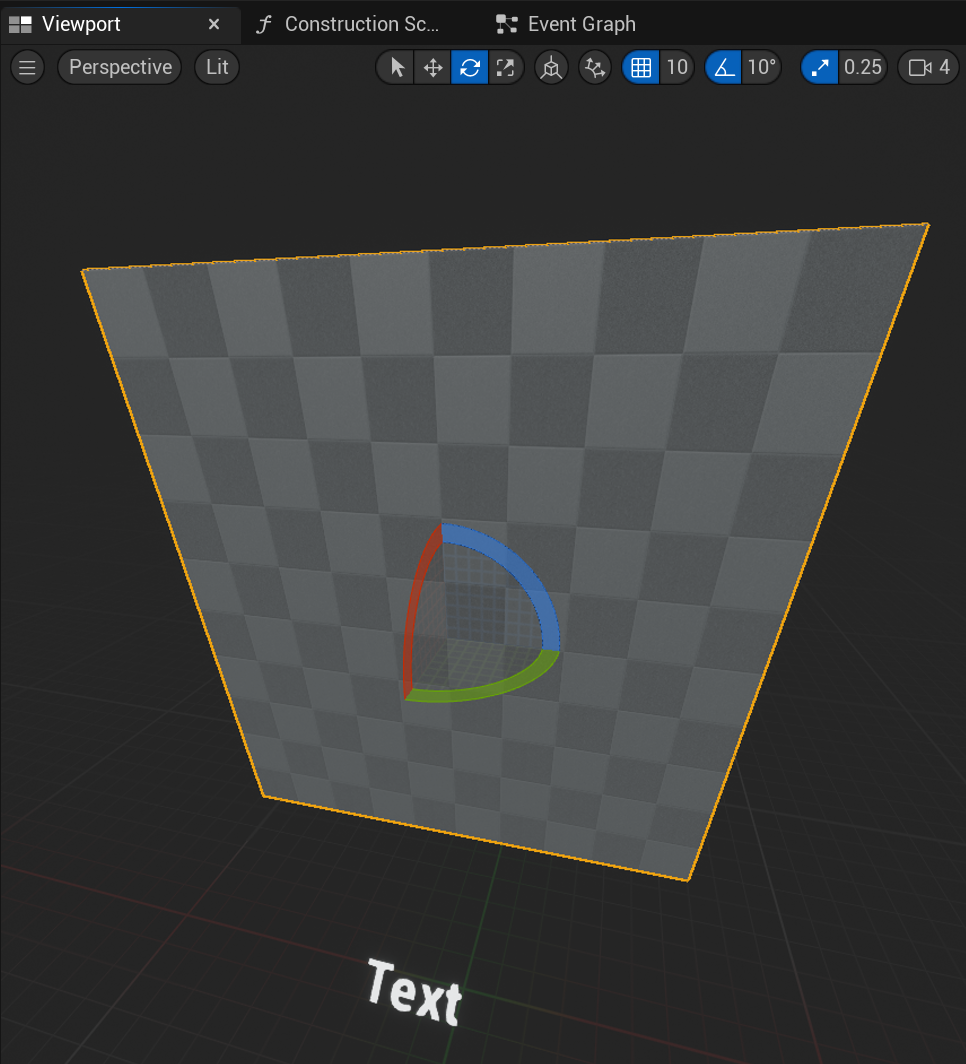
Also set the position and rotation of the Text Component . You can select it from the Components tab or by using the Select object (O) tool, which is the leftmost button located next to the buttons mentioned previously.
After positioning your plane and text as desired, hit the Compile button, save the blueprint, and exit the blueprint editor.
Finally, drag and drop the BP_RemoteImagePlane class into the map and click on Play button (or press Alt+P ). You should see something similar to this:
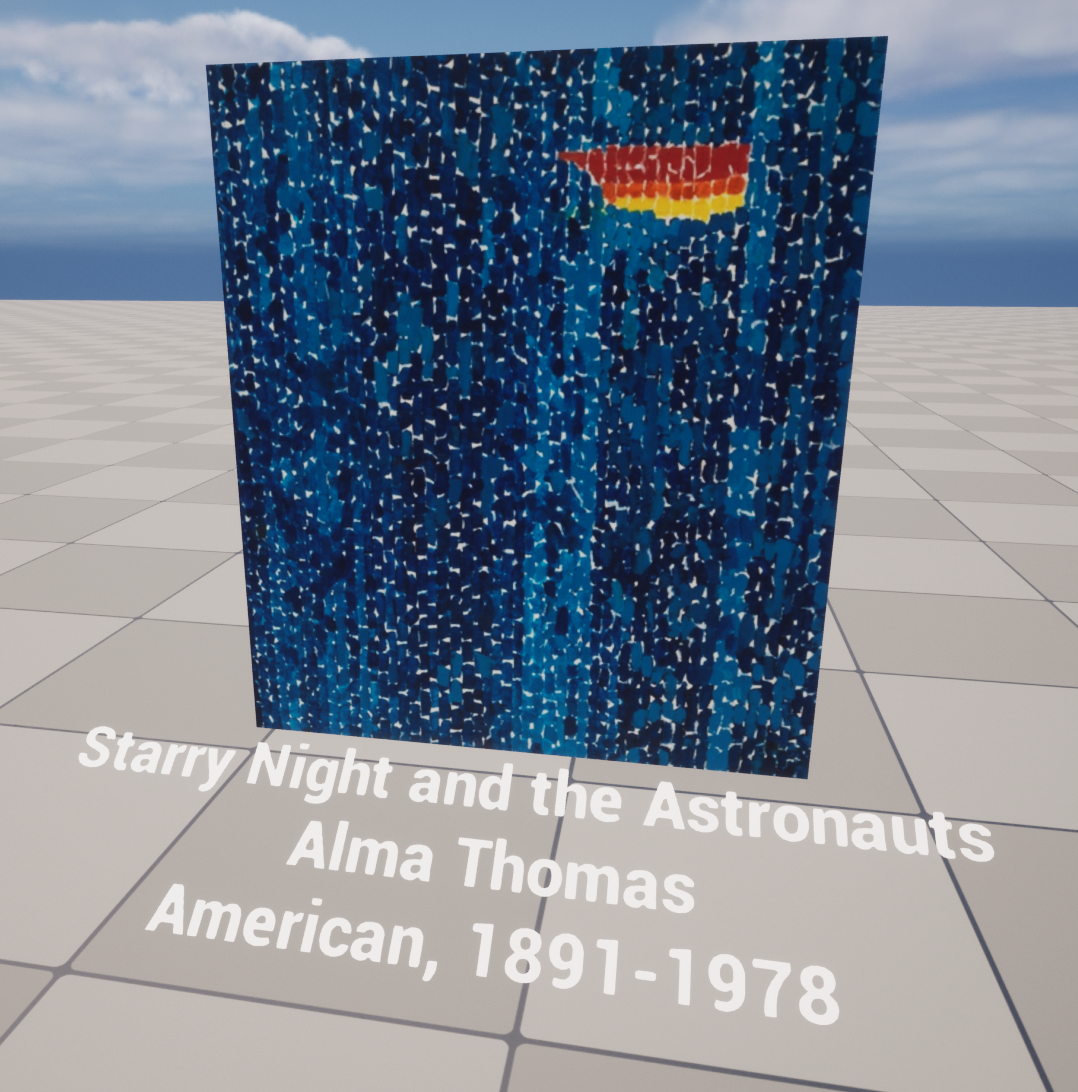
Place some more instances of BP_RemotePlaneImage in the map, and set a different CatalogId for each one in the Details tab while instance is selected.
To obtain more catalog numbers, you can browse the ARTIC collection at https://www.artic.edu/collection . The number is always present in the link to the artwork, such as in the following example: https://www.artic.edu/artworks/ 28560 /the-bedroom .
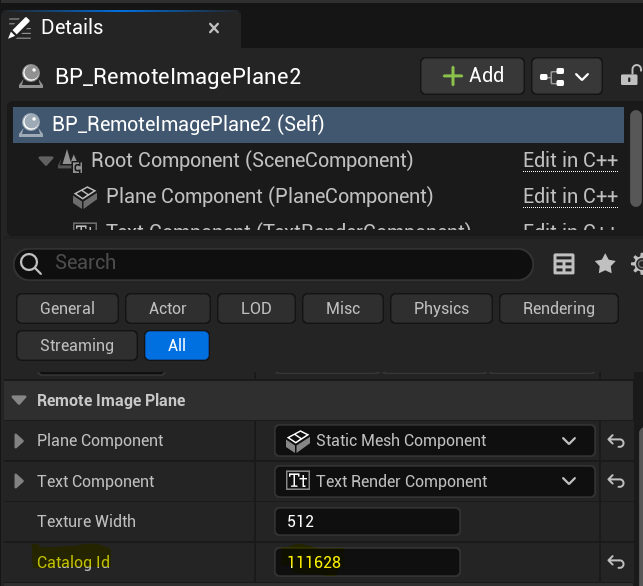
Alternatively, you can obtain catalog numbers and all other public API data from the Data Dump .
Final Thoughts
If you have followed along and managed to get this far, give yourself a pat on the back, especially if you are a beginner. You have learned about APIs, how to make an HTTP request, how to deserialize a JSON response, and how to extract data from complex JSON structures. You have also gained a few other bits of knowledge about Unreal Engine, such as the fact that there are three different types of strings.
On top of that, you now know how to create a dynamic material instance and a transient texture. You also know how to convert image data, such as JPEG format, into an array of raw data in a specific pixel format. You're able to push this data to the texture and set the texture as a texture parameter of the dynamic material in C++, which is a fairly advanced topic.
One final thought on refactoring: almost all the logic is implemented in a few functions, and most of them perform more than what a single function should.
Splitting the logic into smaller functions, each with descriptive names, would be a reasonable thing to do. This can help create self-documented code, which is code that is easy to read and understand without the need for additional comments.
I've decided to keep the tutorial implementation as is so that I can explain it in a linear way. However, the example project has been carefully commented. If you choose to refactor the code, it would be a great exercise and a way to solidify what you've learned today.
Thank you for reading this post. If you enjoyed this post, follow me on Twitter where I post almost exclusively about game development and programming topics.

Subscribe to GameDev.tv Blog - Learning to Code through Video Game Development
Get the latest posts delivered right to your inbox
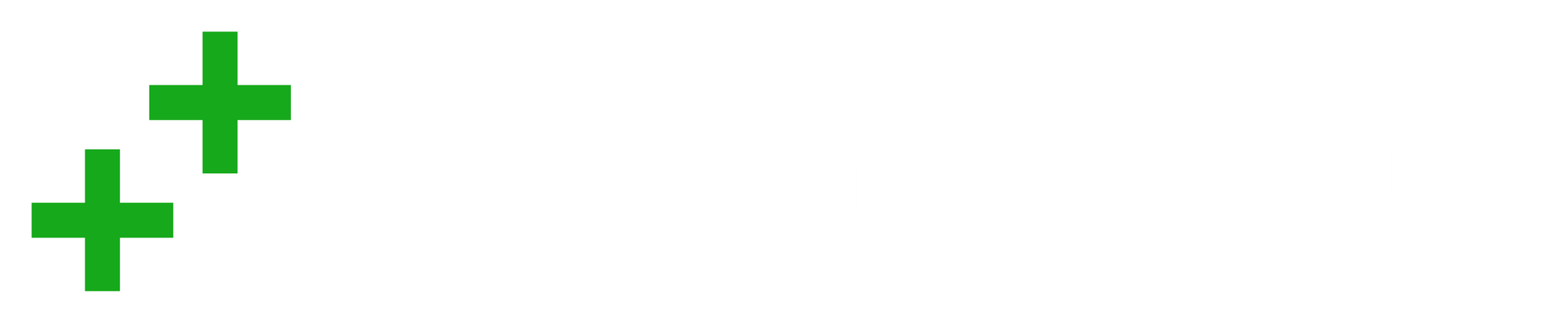
Stay up to date! Get all the latest & greatest posts delivered straight to your inbox
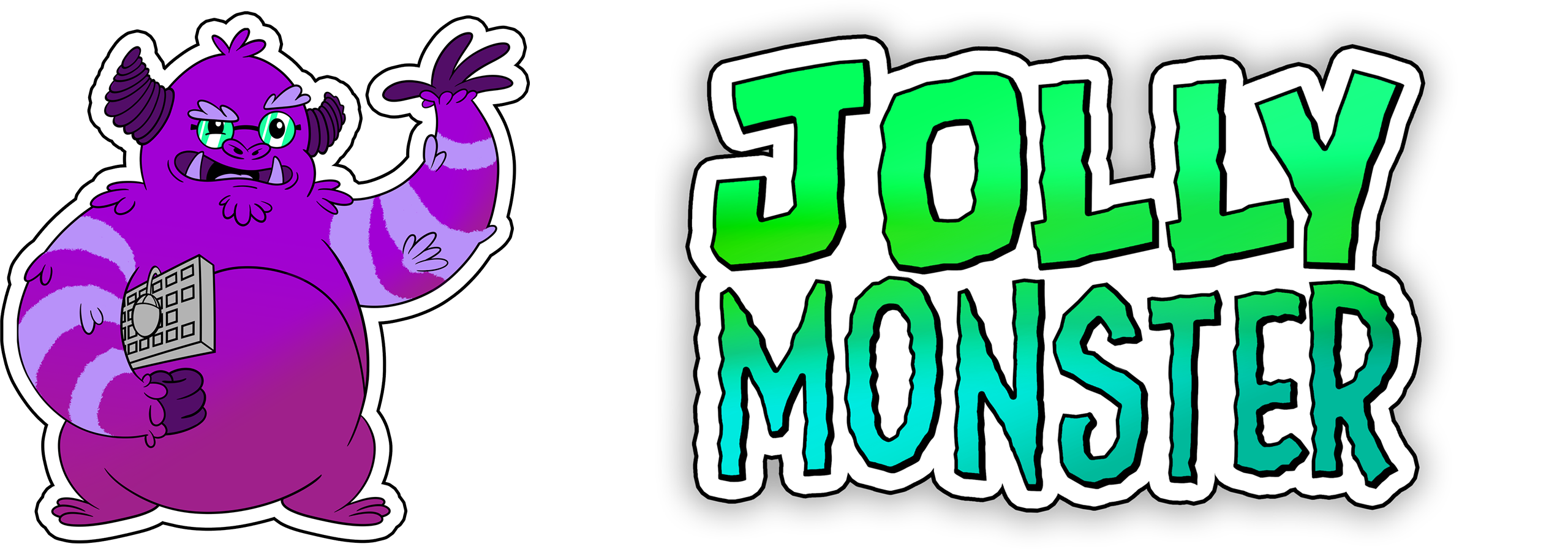
- Unreal C++ Networking – HTTP GET JSON Request Using REST API
Today we are going to start looking at some new content related to networking operations with the Unreal Engine.
Specifically we are going to review how to make HTTP GET calls from within Unreal to an external REST API.
The project files for this video & article can be found on our GitHub page .
In order to start using the HTTP module we first need to modify our Build.cs file to include a few new public dependencies. Specifically Http , Json and JsonUtilities .
With these dependencies included we can define our Actor that is going to handle the work of doing the network communication.
In this case we are using an actor that will relay it’s information to a custom UUserWidget that is attached to this actor via a WidgetComponent .
The other property is our reference to the FHttpModule that will be used for all of our network communication with the server. This is available to us by including the “Http.h” header in our Actor.
Let’s step through our functions one by one and see how they all communicate.
First thing is our overlap that is triggered when the character interacts with this Actor as well as the instantiation of our components and the FHttpModule .
With our objects all setup we can now proceed to looking at the OnBeginOverlap method as that is the first thing our character will interact with.
In the begin overlap we do a few things. We update our user widget ( HTTPResponseWidget ) that a loading operation has started and we try to retrieve the username of the player that interacted with our component.
We then send the players username over to SendHTTPGet() which will try to determine if it’s a valid username or not and make it’s HTTP calls out to an external service.
Here is where we start creating our request structure for the HTTP GET call. This means we have to provide a URL and depending on the username we either use it to retrieve a single record or instead get all users available to us.
We also create callbacks via OnProcessRequestComplete to two separate methods: OnGetUsersResponse and OnGetUserByUsernameResponse .
This allows us to handle the response structure per each GET call separately and offload the processing of those requests to different components if required.
We also include some custom headers that can be used for things like the Content-Type definition but also for more complex security and authentication situations.
The OnGetUsersResponse method will be processing this JSON Payload which includes an array of elements as part of the response.
While the OnGetUserByUsernameResponse will process a single user entity response.
Now let’s take a look at the implementation details for these two payloads.
Lastly in our OnGetUsersResponse and OnGetUserByUsernameResponse methods we can use the reference to the FHttpRequestPtr and FHttpResponsePtr parameters to determine if our calls were successful and came back with a status code of 200
Additionally we can observe how the JSON structure is parsed by first using the FJsonObject in combination with the TJsonReader
This gives us the ability to then get at the individual properties of a JSON response. Specifically since one requires access to an Array.
By using the various getters ( GetArrayField / GetIntegerField / GetStringField ) we can iterate and access the various properties of our payload.
That’s it, you successfully processed a JSON response from an external service using your Unreal code.
Hope this helps you guys make your own network requests.
If you would like to see more examples check out the video as well as the GitHub project for examples of child classes that move the player and particles around.
Recent Posts
- Unreal C++ – Puzzle Mechanics – Pressure Plates and Doors by moving Static Meshes & using Interfaces
- Unreal Engine C++ Fundamentals – Using Inheritance by moving the player & particles along a spline
- Jolly Monster Studio + Patreon & Discord Launch !
- Unreal Engine C++ Fundamentals – Moving Static Meshes along a Spline Component
- December 2021
- November 2021
- October 2021
- October 2019
- September 2019
- February 2019
- January 2019
- December 2018
- November 2018
- October 2018
- February 2018
- BadGuysGoodGuys
- Development
- Tiny Soldiers
Leave a Reply Cancel Reply
You must be logged in to post a comment.
- Privacy Policy
© 2024 Jolly Monster Studio. All Rights Reserved.
Unreal Engine API Reference
The API reference is an early work in progress, and some information may be missing or out of date. It serves mainly as a low level index of Engine classes and functions. For tutorials, walkthroughs and detailed guides to programming with Unreal, please see the Unreal Engine Programming home on the web.
To explore the API from some of the most frequently encountered Unreal concepts and types, see the API getting started page.
Getting started · All constants · All functions · All enums · All classes · Class hierarchy
A Developer's Guide to UC 2024
UC 2024 is around the corner, and we’re incredibly excited to share some of the exciting sessions and events happening where GIS and software development intersect. Read on for information about the sessions being offered , the ArcGIS Developers area within the Showcase, and Developer Day — all happening at UC!
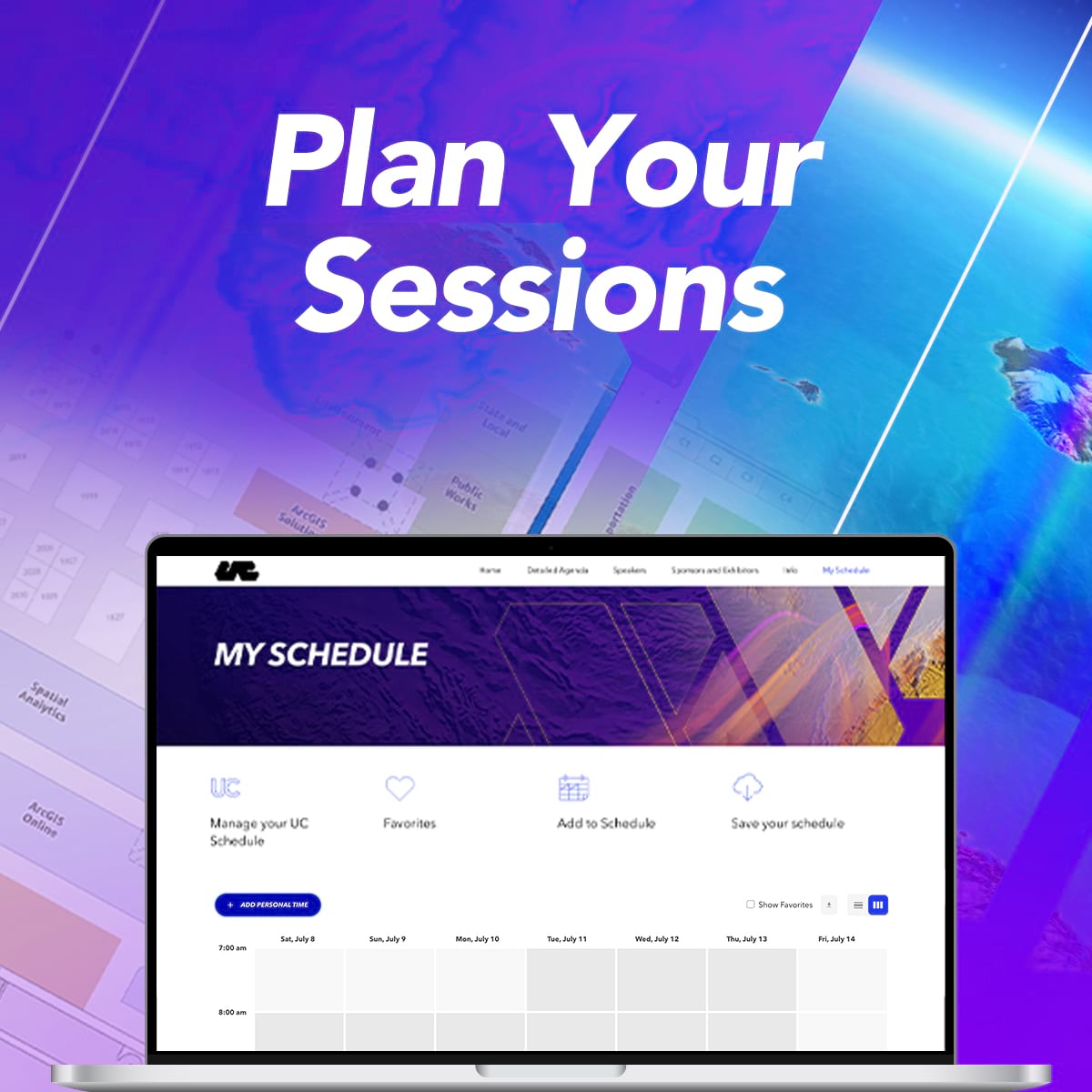
Developer-Focused Sessions at UC 2024
Tuesday, July 16
8:30am Web Development Strategies (Room 31ABC) – This talk is for those new to web development with ArcGIS and interested in learning about the most important technologies available for creating web applications. We will introduce key components of the system architecture, performant web services, ready-to-use location service, configurable applications, low-code solutions, specs for reusing 2D and 3D maps, and a language for dynamic content between applications. We will also explore JavaScript libraries for custom applications, including the fully featured ArcGIS Maps SDK for JavaScript and its web components – the new simplified way to build custom apps.
10:00am JavaScript for Future Web Developers (Demo Theater 1) – Has Desktop development got you down, or have you been too busy to learn web stuff? This is the perfect session for you to start web development! Our enthusiastic team members will guide you though your first web mapping app with JavaScript and web components, introduce web application architecture, and direct you to more resources about web development.
11:30am ArcGIS Experience Builder – Building Your First Widget (Demo Theater 1) – You will learn how to use TypeScript and React to build your first widget in ArcGIS Experience Builder developer edition. We will also show you how to debug your widget to improve your debugging experience.
1:00pm ArcGIS API for Python: Working with Low- and No-Code Application Builders (Demo Theater 1) – With the growing number of apps that can be created using app builders such as ArcGIS Experience Builder, ArcGIS Dashboards, and ArcGIS StoryMaps, finding easy ways to manage all of your organization’s apps becomes more important. This session focuses on using the arcgis.apps module of the ArcGIS API for Python to copy and update apps and working directly with the new WebExperience class.
1:00pm Development Strategies for Desktop and Mobile Apps (Room 5AB) – ArcGIS provides app developers with many tools and options for building useful desktop and mobile mapping applications that use the power of GIS. In this session, we will tour these products and capabilities, so that you can deploy your apps on a variety of devices. We will explore no-code/low-code tools such as ArcGIS AppStudio, the ArcGIS Pro SDK for .NET, ArcGIS Maps SDKs for Native Apps, and ArcGIS Maps SDKs for Game Engines. We will also tour resources such as: ArcGIS location services, usage analytics, samples, open-source projects, training, videos, and interactive community forums.
2:30pm ArcGIS Maps SDK for JavaScript: Data Visualization (Demo Theater 1) – This session will discuss and demonstrate visualization and analytical techniques that are performed client-side. You’ll learn the basics of how to turn your raw data into information that tells a story with data-driven visualizations. We will provide an overview to the various visualization techniques available in the SDK specific to vector data, and will share tips and tricks to help you feel more confident in making smart choices for visualizing your data.
2:30pm ArcGIS API for Python: Administering ArcGIS Online (Room 14AB) – This technical workshop will teach GIS administrators how to use the ArcGIS API for Python to manage their web GIS and automate common repetitive tasks. This talk will cover common workflows in managing users, their credits and licenses, as well as managing groups and content. You will also learn about content migration strategies.
4:00pm Customize Your ArcGIS Pro Experience with Configurations (Demo Theater 1) – This session embarks on a journey to personalize your ArcGIS Pro experience like never before. Using the ArcGIS Pro SDK for .NET, learn about the art of customization using configurations, empowering you to mold ArcGIS Pro to fit your organization’s unique needs and preferences. Whether you’re a GIS manager seeking to streamline operations or a developer eager to explore new customization possibilities, this session offers practical insights and actionable strategies to help unlock the full potential of ArcGIS Pro. From fine-tuning the user interface to automating repetitive tasks and optimizing workflows, you’ll learn how to build your own configurations to transform ArcGIS Pro into your ultimate GIS toolkit.
4:00pm ArcGIS Maps SDK for JavaScript: What’s New and Road Ahead (Room 8) – Attend this session to get a tour of the most important advancements in the ArcGIS Maps SDK for JavaScript that were introduced over the last year and get inspired with new ideas for your web apps. We’ll also give you a sneak peek at several capabilities that are being worked on and discuss our short- and long-term roadmap.
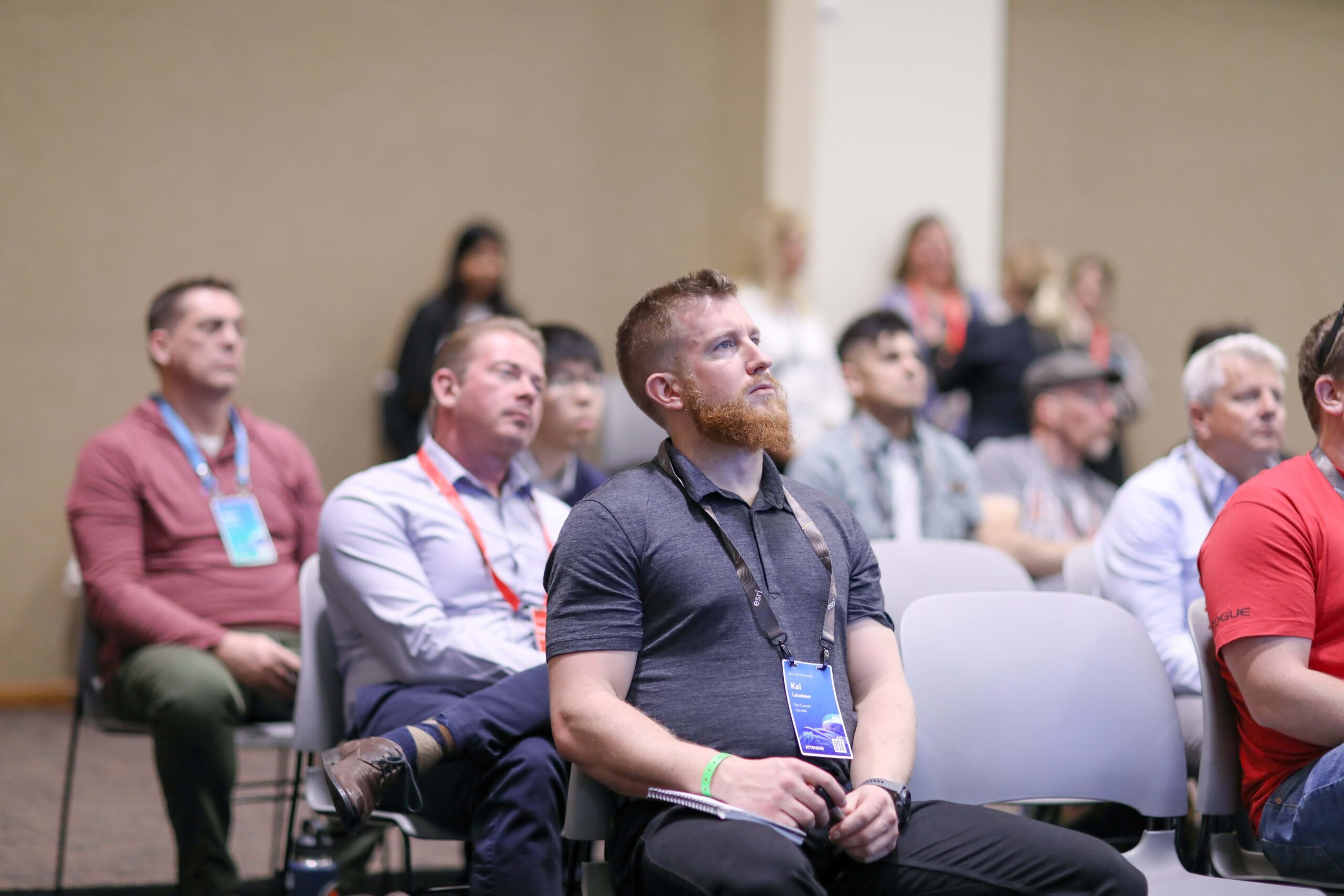
Wednesday, July 17
11:30am ArcGIS Maps SDK for JavaScript: Using ArcGIS Arcade in Your Apps (Demo Theater 1) – This demo theater will demonstrate how you can transform your data from one or more field values to computed data values for use in data visualizations, popup content, forms, and labels. We’ll also provide a beginner’s overview to the Arcade language.
1:00pm ArcGIS Maps SDK for Native Apps: What’s New and Road Ahead (Demo Theater 1) – ArcGIS Maps SDKs for Native Apps enable you to build applications with .NET, Java, Qt, Swift, and Kotlin that work with the ArcGIS system and run directly on desktop and mobile devices, online or offline. Over the last year, several new features have been introduced, including real-time support, clustering, offline editing and tracing of utility networks, and the introduction of new data sources such as 3D Tiles. This session will cover these and other improvements and provide a sneak peek at what’s coming next.
2:30pm ArcGIS Maps SDK for Game Engines: What’s New and Road Ahead (Demo Theater 1) – ArcGIS Maps SDKs for Game Engines enable you use Unity and Unreal Engine to build immersive, cutting-edge 3D and XR experiences with real-world data. Over the last year several new features have been introduced such as clip, mask, replace and filter to modify geospatial data on the fly and support for virtual tabletops. This session will cover these and other improvements and provide a sneak peek at what’s coming next.
4:00pm ArcGIS API for Python: Managing Web Maps and Layers (Demo Theater 1) – In this technical workshop, we’ll demonstrate how to use the ArcGIS API for Python to author, edit, and visualize web maps and web layers. We will demonstrate some common workflows including editing pop-ups, group layers, switching basemaps and layers, and finally cloning GIS content.
4:00pm ArcGIS Location Platform: What’s Is It and Who Is It For? (Room 2) – ArcGIS location services are web services hosted by Esri that you can use to build mapping and spatial analysis applications. Learn about the capabilities of each service, how to access them securely, and the many client APIs available to use in your apps. Intended for the Technical Manager/CTO/CEO level review of the use cases and business model when developing applications with ArcGIS Location Platform, ArcGIS Online, and ArcGIS Enterprise.
Thursday, July 18
10:00am ArcGIS Experience Builder: Building Your First Widget (Demo Theater 1) – You will learn how to use TypeScript and React to build your first widget in ArcGIS Experience Builder developer edition. We will also show you how to debug your widget to improve your debugging experience.
11:30am ArcGIS API for Python: Automating Tools to Monitor Your Organization (Demo Theater 1) – This demo theater will explore using the ArcGIS API for Python to monitor any organization’s usage and costs. Using the tools provided by ArcGIS Enterprise, attendees will learn how the ArcGIS API for Python can automate monitoring tasks such as logging and reporting to build a complete picture of consumption and usage within the organization.
1:00pm ArcGIS AppStudio: Options for Migration (Demo Theater 1) – With ArcGIS App Studio Retiring February 2025, current users will need migrate their current AppStudio applications to other supported ArcGIS options. This session will outline how the different ArcGIS SDKs can replicate AppStudio workflows and functionalities, and as well as demonstrate some of the little to no code solutions.
4:00pm ArcGIS Maps SDK for JavaScript: Improving UX with Pop-ups (Room 30AB) – In this technical workshop, you’ll learn how to utilize recently added features in the Map Viewer and the ArcGIS Maps SDK for JavaScript that allow you to modify the popup’s appearance and behavior. We’ll cover how to configure different elements within the pop-up, display charts, and more to modify the look and feel the of the popup to meet your application’s requirements.
Showcase: ArcGIS Developers
Stop by the ArcGIS Developers area of the Showcase in Hall C to talk with any of our developers and product engineers about our SDKs and how they can be utilized with your projects and solutions.
The following products will be covered:
ArcGIS Maps SDK for JavaScript
Calcite Design System
ArcGIS Maps SDKs for Native Apps
ArcGIS Maps SDKs for Game Engines
ArcGIS API for Python
ArcGIS Location Platform
ArcGIS Location Services
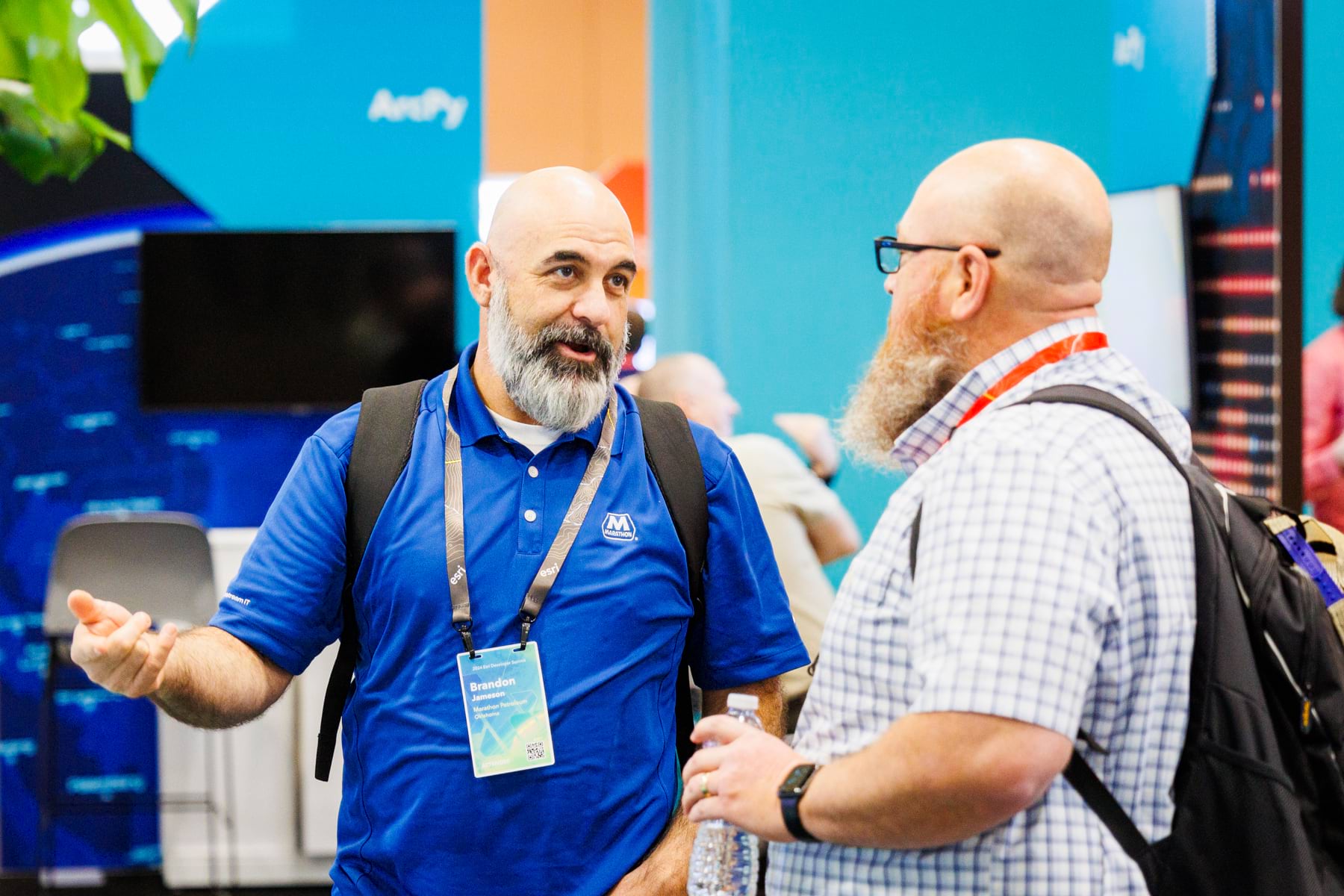
Don’t miss our Expo Open Platform Spotlight Talks happening in the Showcase as well!
10:40am Esri and Open Source – Open-source geospatial libraries are a popular tool used in many geospatial workflows. Join us for a brief review of important open-source projects that are sponsored by Esri. Learn about how open source works as well as how to get involved and contribute to open-source technology.
10:00am Esri Services: Integrating with Open Source Libraries – Presenters provide a brief, high-level overview of how to integrate Esri Services with open source libraries such as Leaflet, MapLibre, OpenLayers, and CesiumJS.
11:30am Using Esri’s ArcGIS REST JS – Presenters briefly describe how to use open-source ArcGIS REST JS for lightweight service integration into any JavaScript app, whether server or front-end.
Developer Day at UC 2024
Join us on Wednesday, July 17th, at the San Diego Convention Center for Developer Day . This all-day event caters to all developer levels, from exploring low-code solutions to deep diving into GIS app development. The general session and technical sessions will take place in Room 7AB.
Included with your UC registration, Developer Day offers a unique opportunity to:
- Learn from Esri’s development teams and connect with fellow GIS developers.
- Gain insights into building real-time, performance-optimized apps using various ArcGIS tools.
- Explore the integration of GIS with advanced technologies like Python, game engines, and knowledge graphs.
This is more than just a learning experience; it’s a chance to shape the future of GIS development in your organization.
The day begins with a general session which is a comprehensive overview of what the ArcGIS System is, highlighting its benefits and architecture through demonstrations. We kick off the morning with a warm welcome and an introduction to community through the developer story and discovering how leveraging APIs provides underlying capabilities to you as a developer. Then we continue l everaging those c apabilities shared as services and APIs to build apps that can be tailored to your requirements. Finally, we will share some of the highlights of ArcGIS developer tools that are available to use and get started with today.
After the general session, we will offer the following technical sessions:
1:00pm ArcGIS Maps SDK for JavaScript: Fundamentals (Room 7AB) – Attend this session to get a tour of the most important capabilities in the ArcGIS Maps SDK for JavaScript, the fundamentals of the API, and get inspired with new ideas for your web apps. We’ll cover bringing in data with any format, basic layer styling, editing, client-side analysis, shortcuts for building your UI, and more, with engaging demos and detailed explanation about the techniques and capabilities.
2:30pm Faster Web Development with ArcGIS Web Components (Room 7AB) – We’ll introduce the brand-new web components that are part of the ArcGIS Maps SDK for JavaScript for bringing ArcGIS experiences into your app with a few lines of code. We’ll also demonstrate how you can use Calcite Design System web components to build accessible, consistent web applications faster. When you use Calcite and the JavaScript Maps SDK components and capabilities together, you can create a compelling web mapping application with maximum productivity.
4:00pm ArcGIS Experience Builder for Developers (Room 7AB) – ArcGIS Experience Builder enables you to quickly build web apps and web pages with no-low code. It is highly configurable with flexible design, mobile optimization, smart widgets, and integration. It is extensible so you can add your own tools to deliver production ready solutions fast. Come to learn the developer edition from installation, collaboration, deployment, automation, to upgrade, including importing apps from ArcGIS Online, adding custom widgets to apps or ArcGIS Enterprise, etc.
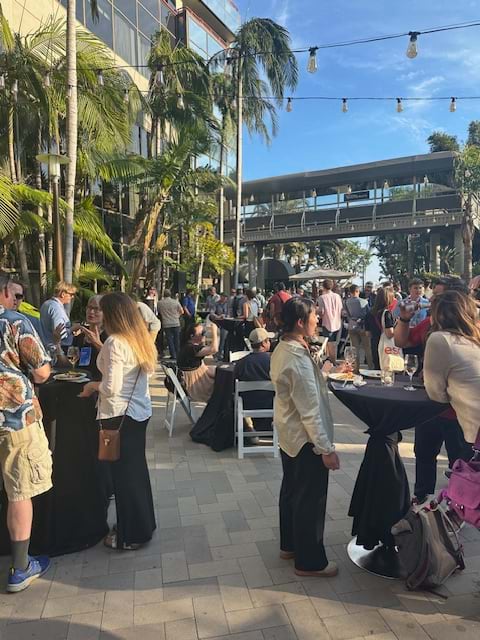
Developer Social
Developer Day finishes off with a networking social over at the Marriott Marquis South Pool. We will have delicious appetizers and beverages to enjoy while connecting with other attendees and developers. Catch up, learn from others, and share some of your stories all while listening to the best DJ ever!
If you are not registered for UC but would like to attend the Developer Social, you can register here .
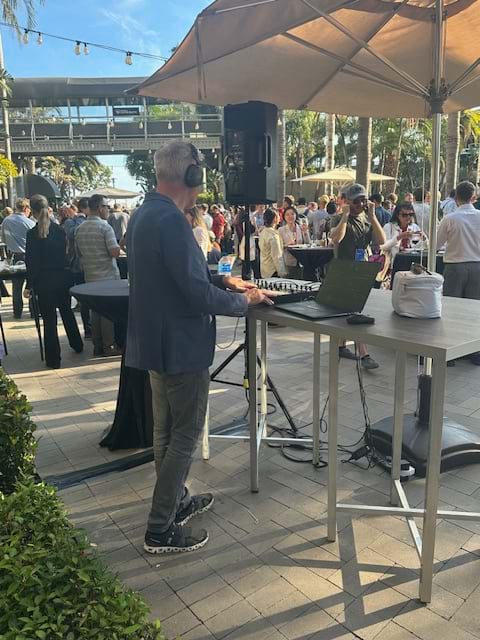
About the author
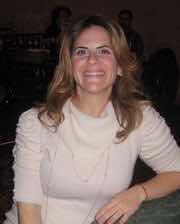
Amy Niessen
Amy is the Developer Community Manager in Product Management. She has an MA in English Literature and Composition and an MBA with a focus on Global Business. Follow the @EsriDevs and @EsriDevEvents accounts on Twitter for updates on developer community events and happenings!
Article Discussion:
Related content:.
- developer day
- developer social
- esri uc 2024
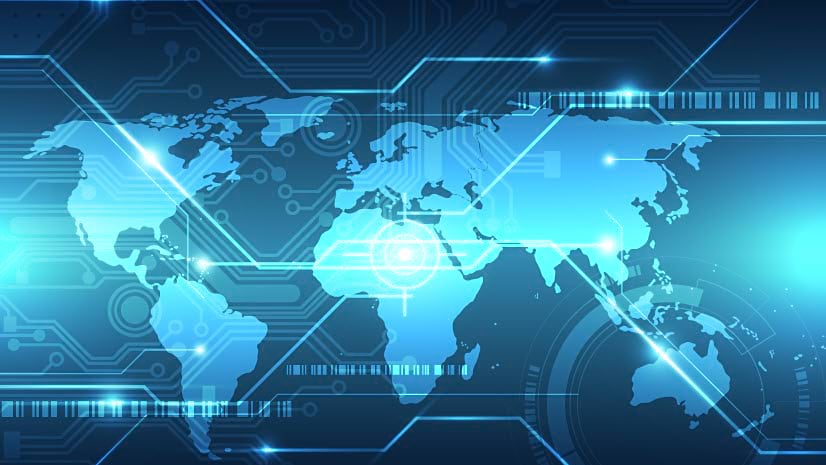
Next Article
Changing the Map Projection in ArcGIS Online
Read this article
Calling a remote REST API
I’d like to be able to call my own REST API to implement systems such as voting, analytics and global in-game events. Is it going to be possible in the future?
This is not currently possible, and there are no firm plans at the moment I’m afraid. This would obviously be challenging from a security and moderation perspective, for example.
Roblox has this capability. We should have it, too. Please reconsider this one and please make it a priority. There is so much we can do with this capability.
Hey, I’m curious what would be the worst thing that could happen from adding HTTP Request support? Roblox has a limit of 500 request per minute per server so it can’t be used for any specific illegal activities. And Roblox has blocked any and all requests to their own domains, I can’t think of ANY possible security issues if those two measurements are implemented.
You could, for example, circumvent the moderation processes (for disallowed content or monetization)
You should just let us only call GET requests than at the very least, I can understand the possibility of bypassing monetization, however, but I do not understand how we might bypass the disallowed content.
Why not simply allow HTTP requests if they are validated developers through the Epic Online Services portal? Making it require coming from the same domain.
Thank you for the feedback, I will pass it on to the team.
Oh, this is an Epic fail. Seriously, there is nothing open or interoperable about this decision. This is a walled-garden mentality and frankly an innovation killer. Not being able to integrate functionality that exists outside the Fortnite fortress walls means that Fortnite will not give creators the ability to use the most important interactive innovations - generative AI. Look at services like inworld - that is a critical piece for more engaging experiences.
Furthermore, it has a negative knock-on effect on the economy. With creators not able to bring in functionality that exists outside the Fortnite system, Epic then owns the “means of production” . Epic can build whatever features suit themselves, Creators just have to live with the functionality they are given.
Look around at all the advances in LLM’s being used as coding assistants (Codex, Copilot) the need to “eliminate runtime errors” and the “security” bogeyman are antiquated ideas. Then look at what Rust has to offer. Do you really need to invent a new language? If @Tim_Sweeney is going to look back to Pascal for syntax examples, why not look back to Anders Hejlsberg who made Pascal usable with Delphi and then went on to design C#.
Please, please reconsider. Make the system smarter. Make the system easier. Make the system approachable.
Please DO NOT force a new language with a unique, perhaps innovative structure on people. Millions and millions of people have spent a tremendous amount of time and effort learning procedural languages. They make sense to people who are not about “math”. I understand the challenges that dynamic languages pose at an analysis level, I would happily give up dynamic features.
On the syntax level, if you want to do something that is accessible and easy to learn, you should look back to SmallTalk. If you are going to build a virtual world, you really should have syntax that models the real world.
Honestly, the whole world is being turned upside-down by LLM’s. Writing code is being revolutionized - and the revolution is not functional syntax. The future of coding is natural language based . Put your effort into getting things at the compiler level, don’t push the hard work on the creators.
Here is another example. Look at the success of Visual Studio Code. Would it have been successful if you could not bring in functionality from the outside. A world without Codex (from OpenAI) and there would be no competition for Copilot (from GitHub). You need to have competition for functionality/capability.
At the end of the day, it is all about the INTEROP. Without Interoperability, you will never, ever build an ecosystem that will thrive. Without Interoperability you are, by definition, creating a walled garden.
I look forward to continuing this conversation here on the forums and out in the open.
Sincerely yours from a real believer in the rest of the Epic metaverse vision - and a massive fan or Unreal Engine (full version) as one of the most empowering creative force in the world today.
@Dig_Squid - would appreciate your thoughts or your colleagues.
Thanks very much for your feedback, I have passed it on. There is a lot to think about there, I do agree that generative AI is a hugely important topic.
Agreed that Fortnite Creative 2.0 is basically a walled garden right now. It’s open for UGC which is nice, and they’re cutting us in which is nice, but it’s still a walled garden.
I think I understand why now, though. Epic’s intention is to foster the “open metaverse” as per State of Unreal '23 and multiple interviews but it’s not quite clear yet (to them or to us) how that’s going to happen.
In other words, we’re still in the early days and we’re along for the ride.
Tim Sweeney recently said “no” here when asked if NFTs would be implemented in Fortnite Creative 2.0 (original post/question since deleted). But he previously implied it was a possibility during State of Unreal '23. So, I’m taking it as a no for now .
I also saw a comment from @Dig_Squid here that one of the reasons for not giving us REST functionality is that it would allow us to “ circumvent the moderation processes (for disallowed content or monetization) .”
By the way, one thing to take note of that was not explicitly called out by @Dig_Squid is that because Fortnite is available across almost every platform, and each platform has varying monetization policies, I think we’ve so far ended up with the least common denominator. Were Fortnite to allow UGC to bypass these policies, they (Epic) would be liable and it could get Fortnite in hot water with the platform owners.
thanks so much @Dig_Squid
Here is an article that sheds some light on their outlook on the metaverse and their current strategy:
Tim said over and over, no walled gardens. He also said Interoperable and Open. End of story. Either it is open and not a walled garden or it is not. No access to APIs outside the “Fortnite” system is, by definition, a closed system. Moderation policies are not compromised. There is plenty that can be implemented at runtime to keep an eye on that. Finally, being able to circumvent the Monetization system is part and parcel of an open and interoperable (not a walled garden) environment. I mean come on, after all the fighting with the Apple AppStore, I find it curious that Epic “require” that all apps participate in the nacent Fortnite Economy outlined by Tim.
The aim is to get from here towards the open metaverse along an incremental path that respects privacy rights and privacy laws around the world, and doesn’t retrace the steps that made the open web a fiasco in this regard and are putting Roblox on a shaky foundation.
The next step we’re working on right now is a system to provide simple but useful persistence soon that doesn’t expose private player information to external web servers, designed to be player friendly and respect the relevant laws around private information, deletion requests, and so on. Over time this can evolve into an open spec for federated persistence across different services, built on top of OpenAuth and various data privacy standards. (Our account system already OAuths with PlayStation, Nintendo, Xbox, Android, Steam, Google, and Apple, and we’re working towards a federated approach.)
The long term aim for persistence isn’t the wild west of everyone sending player data to servers around the world, but that it’s built on the foundation of automatically persisting the contents of Verse data structures and making them accessible (live) across different game sessions, with sensitive built-in data types such as players designed to protect privacy with respect to federation boundaries, without complicating user-made data structures.
If you want to make API calls to servers around the world, why not run Unreal Engine on your own server?
It seems like you want UEFN to be something it’s not. There is room in an ecosystem for both walled garden spaces and fully open hosting style. Maybe some people want to play games within a closed platform where they know their activity isn’t being sent off to servers in China, or Russia, or who knows where?
Even if you prevent images and files from being downloaded with these requests, what happens when your external server goes down and your game starts throwing errors–this makes Fortnite Creative look bad and they will probably get support calls.
I understand why Epic is creating this as a more controlled space and I think there is a place for it among everything else.
@Tim_Sweeney I really appreciate the thoughtful reply. I do believe that there needs to be an ongoing discussion between Epic and the Creators on fundamental aspects like this. I totally get that you are taking an incremental approach and I whole-heartedly support that. I trust that Epic/UE will continue to put awesome tools in every creators hands. The actions over the past 18 months makes me certain of this. There are 2 issues I am concerned about. Verse and Language accessibility What I am concerned about is putting too much plumbing into the creator workflow. Verse is tough. I really wish we were looking backwards a bit when designing a language for regular folks. Alan Kay shares amazing insights on the Smalltalk journey here - The Early History Of Smalltalk Free the users/creators from being saddled with a language that is non-obvious and hard to read. The Kay paper has some excellent examples.
2. Calling Outside APIs I am coming to this with the perspective of a creator who has been using UE. I have come to rely on the innovations made by members of the ecosystem. I look at systems like PlayFab and inworld. PlayFab being a battle tested back end, certainly up to the load. Second would be the emerging offerings like inworld which give creators incredible power. My hope is that use of these two an many in between will be possible.
At the end of the day Tim, I laud your efforts to avoid the mistakes made in the recent past. I also firmly stand by the ethos of “rough consensus and running code”. Your code is running, let’s get to rough consensus. Without getting the creators productive on the platform, I fear the explosive growth will be greatly tempered.
Once again, thanks for taking the time. It shows you really care.
I’d also love the ability to make external REST calls and, to take it one step further!!, even make Websocket requests. I’m working on a simple web based game that’s strictly UI, but it’d be super awesome to integrate into the FN ecosystem and playerbase using UEFN and create a 3D world that builds on top of what can be played in-browser.
Is there an update on this topic? I agree that it’s a pretty important feature to be able to make external API calls.

COMMENTS
Call a SatCat REST API from Unreal Engine. Example application on GitHub. Location of example application implementation: ASAT Debris Unreal Engine Example on GitHub. If you follow the full tutorial, or build the example UE5 Application from GitHub you'll complete a 3D map displaying satellite test debris. And, here we learned:
VaRest is the plugin for Unreal Engine 4 that makes REST server communications easier to use.. Key features: Flexible Http/Https request management with support of different Verbs and Content Types; No C++ coding required, everything can be managed via blueprints; Blueprintable FJsonObject wrapper with almost full support of Json features: different types of values, arrays, binary data content ...
The HTTP Streamer's REST API provides functionality for streaming replays from the game server to the replay server (uploading), for streaming live or pre-recorded replays from the replay server to the game, or viewer, server (downloading), or for querying information about the replays available on the server (searching).
Unreal Engine's HTTP and JSON modules make it ultra-easy to send requests over Http. Learn how to make your own Live 3D visualization of space debris orbiting Earth, by querying a Satellite Catalog REST API. https://dev… Unreal Engine's HTTP and JSON modules make it ultra-easy to send requests over Http. ...
In this video, we go over how to make HTTP requests in Unreal Engine using the HTTP module and without relying on an external plugin or third-party library. ...
Supported Platforms. Supported Engine Versions. 4.11 - 4.27, 5.0 - 5.4. Download Type. Engine Plugin. This product contains a code plugin, complete with pre-built binaries and all its source code that integrates with Unreal Engine, which can be installed to an engine version of your choice then enabled on a per-project basis.
VaRest is the plugin for Unreal Engine 4 that makes REST server communications simple to use with full Json query support.. Key features: Flexible Http/Https request management with support of different Verbs and Content Types; No C++ coding required, everything can be managed via blueprints; Blueprintable Json wrapper with almost full support of Json features: different types of values ...
Texture->AddToRoot(); Now create a pointer to texture data, copy the data from UncompressedBGRA buffer and update resource with the new data. This involves sending the texture data to the GPU, where it can be used in rendering. void* TextureData = Texture->GetPlatformData()->Mips[0].BulkData.Lock(LOCK_READ_WRITE);
In this video we look at how to make HTTP GET calls against a custom API that retrieves a list of users. We also process the JSON response and populate a use...
For Unreal Engine 4 and Unreal Engine 5. This is a quick tutorial to walk you though using Unreal Engine to get data through APIs and webservices. It makes u...
Games, programs and the Unreal Editor are all targets built by UnrealBuildTool. Each target is compiled from C++ modules, each implementing a particular area of functionality. Your game is a target, and your game code is implemented in one or more modules. Code in each module can use other modules by referencing them in their build rules.
The API reference is an early work in progress, and some information may be missing or out of date. It serves mainly as a low level index of Engine classes and functions. For tutorials, walkthroughs and detailed guides to programming with Unreal, please see the Unreal Engine Programming home on the web. To explore the API from some of the most ...
Specifically we are going to review how to make HTTP GET calls from within Unreal to an external REST API. The project files for this video & article can be found on our GitHub page. In order to start using the HTTP module we first need to modify our Build.cs file to include a few new public dependencies. Specifically Http, Json and JsonUtilities.
VaRest is the plugin for Unreal Engine 4 that makes REST server communications simple to use with full Json query support.. Current version: 1.1 RC 8 Key Features: Flexible Http/Https request management with support of different Verbs and Content Types; No C++ coding required, everything can be managed via blueprints; Blueprintable Json wrapper with almost full support of Json features ...
An Unreal Engine plugin for interacting with Rest API, performing Http Requests, and parsing Jsons! Provides a simple Blueprint exposed C++ interface to easily integrate the some of the C++ Http, Json, and Json Utilities modules' functionality into Unreal Engine Blueprints.
Unreal already has built in json classes so if you use c++ you can again do it this way . Wraiyth's Blog - 1 Mar 15 Using Json in Unreal Engine 4 - Part 1. Unreal Engine 4 provides some great JSON utility structures and functions for parsing and creating JSON.
Restful API Json Web Token HTTP. WanWanHa -Code Plugins -Jul 27, 2022. Not Yet Rated. 5 of 5 questions answered. ... This product contains a code plugin, complete with pre-built binaries and all its source code that integrates with Unreal Engine, which can be installed to an engine version of your choice then enabled on a per-project basis ...
Hi. I've created a tutorial that will show you step by step how to utilize and connect with an API (programmable interface). In this tutorial we connect with...
The API reference is an early work in progress, and some information may be missing or out of date. It serves mainly as a low level index of Engine classes and functions. For tutorials, walkthroughs and detailed guides to programming with Unreal, please see the Unreal Engine Programming home on the web. To explore the API from some of the most ...
2:30pm ArcGIS Maps SDK for Game Engines: What's New and Road Ahead (Demo Theater 1) - ArcGIS Maps SDKs for Game Engines enable you use Unity and Unreal Engine to build immersive, cutting-edge 3D and XR experiences with real-world data. Over the last year several new features have been introduced such as clip, mask, replace and filter to ...
unreal-editor-for-fortnite, fortnite. interacsion (interacsion) March 25, 2023, 1:54pm 1. I'd like to be able to call my own REST API to implement systems such as voting, analytics and global in-game events. Is it going to be possible in the future?